How to Convert Date to String in Java
-
Convert
Date
toString
UsingSimpleDateFormat
in Java -
Convert
Date
toString
UsingDateFormatUtils
Class in Java -
Convert
Date
toString
UsingDateTimeFormatter
in Java -
Convert
Date
toString
With the Time Zone in Java -
Convert
Date
toString
WithString
Class in Java
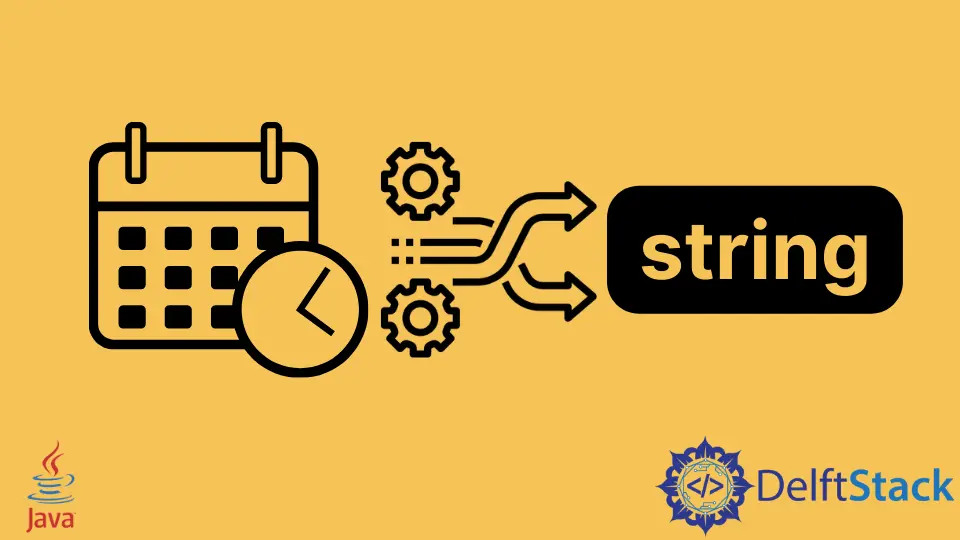
This tutorial introduces how to convert java.util.Date
to String in Java and lists some example codes to understand it.
Java has several classes and methods that help convert Date
to String
like using SimpleDateFormat
, DateFormatUtils
, and DateTimeFormatter
class.
Convert Date
to String
Using SimpleDateFormat
in Java
Here, We use format()
method of SimpleDateFormat
class to get String
from the util.Date
object in Java.
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) throws ParseException {
DateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy HH:mm:ss");
Date date = new Date();
String dateToStr = dateFormat.format(date);
System.out.println("Date is " + dateToStr);
}
}
Output:
Date is 21/09/2020 08:48:01
Convert Date
to String
Using DateFormatUtils
Class in Java
If you are using the Apache
library then use format()
method of DateFormateUtils
class. It returns a string after converting java.util.Date
to string in Java.
import java.text.ParseException;
import java.util.Date;
import org.apache.commons.lang3.time.DateFormatUtils;
public class SimpleTesting {
public static void main(String[] args) throws ParseException {
Date date = new Date();
String dateToStr = DateFormatUtils.format(date, "yyyy-MM-dd HH:mm:SS");
System.out.println("Date is " + dateToStr);
}
}
Output:
Date is 2020-09-21 08:51:203
Convert Date
to String
Using DateTimeFormatter
in Java
Here, we use the format()
method that takes the ofPattern()
method as an argument and returns a string representation of a date. See the example below.
import java.text.ParseException;
import java.time.ZoneOffset;
import java.time.format.DateTimeFormatter;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) throws ParseException {
Date date = new Date();
String dateToStr =
date.toInstant().atOffset(ZoneOffset.UTC).format(DateTimeFormatter.ofPattern("dd-MM-yyyy"));
System.out.println("Date is " + dateToStr);
}
}
Output:
Date is 21-09-2020
Convert Date
to String
With the Time Zone in Java
Here, we use format()
method of the DateTimeFormatter
class to get string after conversion from java.util.date
. We get time zone along with date because we specified the date-time format in the ofPattern()
method.
import java.text.ParseException;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) throws ParseException {
Date date = new Date();
DateTimeFormatter format =
DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss:SSS Z").withZone(ZoneId.systemDefault());
String dateToStr = format.format(date.toInstant());
System.out.println("Date is " + dateToStr);
}
}
Output:
Date is 2020-09-21 09:10:23:991 +0530
Convert Date
to String
With String
Class in Java
This is one of the simplest solutions to get a String of java.util.date
object. Here, we use the format()
method of the String
class that formats the date based on the specified format. See the example below.
import java.text.ParseException;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) throws ParseException {
Date date = new Date();
String dateToStr = String.format("%1$tY-%1$tm-%1$td", date);
System.out.println("Date is " + dateToStr);
}
}
Output:
Date is 2020-09-21
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java