How to Compare Two Dates in Java
-
before()
Method to Compare Two Dates in Java -
after()
Method to Compare Two Dates in Java -
Use the
equals()
Method to Compare Two Dates in Java -
compareTo()
Method to Compare Two Dates in Java
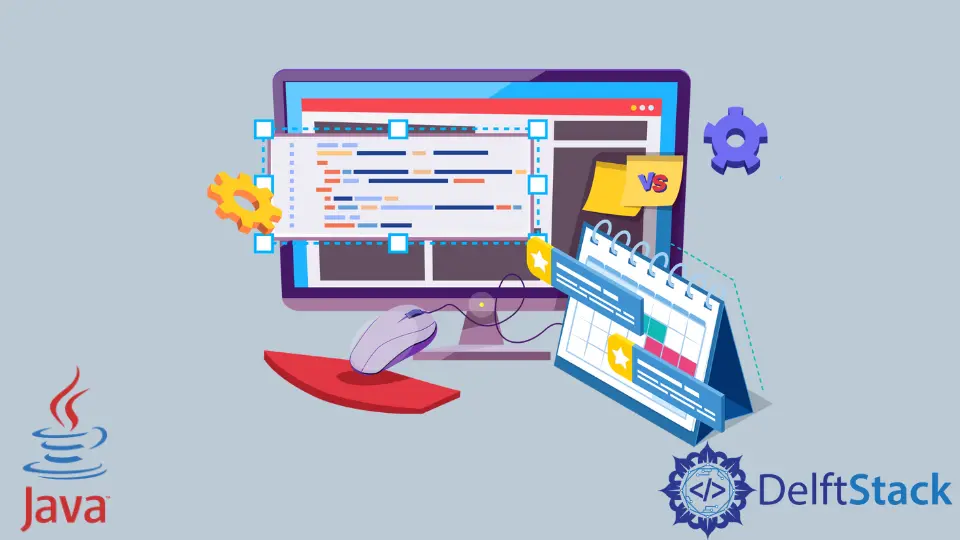
In this tutorial, we will learn multiple methods to compare dates
in Java. To achieve desirable results, we need methods i.e., before()
, after()
, equals()
and compareTo()
.
before()
Method to Compare Two Dates in Java
The first method is the before()
method in Java Date
class that performs the comparison of the Date
object and the given Date
instant. It returns true
when the Date
object comes earlier than the given Date
object.
Example Codes:
// java 1.8
package simpletesting;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
try {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date date1 = dateFormat.parse("2019-09-16");
Date date2 = dateFormat.parse("2020-01-25");
System.out.println("Date-1: " + dateFormat.format(date1));
System.out.println("Date-2: " + dateFormat.format(date2));
if (date1.before(date2)) {
System.out.println("Date-1 is before Date-2");
}
} catch (ParseException ex) {
}
}
}
Output:
Date-1: 2019-09-16
Date-2: 2020-01-25
Date-1 is before Date-2
after()
Method to Compare Two Dates in Java
Another approach to achieve this comparison is using the after()
method in Java Date
class. This method is opposite to the above before
method and returns true
when the Date
object comes later than the given Date
object.
Example Codes:
// java 1.8
package simpletesting;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
try {
SimpleDateFormat sdformat = new SimpleDateFormat("yyyy-MM-dd");
Date date1 = sdformat.parse("2020-01-25");
Date date2 = sdformat.parse("2019-09-16");
System.out.println("Date-1: " + sdformat.format(date1));
System.out.println("Date-2: " + sdformat.format(date2));
if (date1.after(date2)) {
System.out.println("Date-1 is after Date-2");
}
} catch (ParseException ex) {
}
}
}
Output:
Date-1: 2020-01-25
Date-2: 2019-09-16
Date-1 is after Date-2
Use the equals()
Method to Compare Two Dates in Java
Another approach is to use the equals()
method in Java Date
class. It compares two dates and returns true
if they are equal.
Example Codes:
// java 1.8
package simpletesting;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
try {
SimpleDateFormat sdformat = new SimpleDateFormat("yyyy-dd-mm");
Date date1 = sdformat.parse("2020-01-25");
Date date2 = sdformat.parse("2020-01-25");
System.out.println("Date-1: " + sdformat.format(date1));
System.out.println("Date-2: " + sdformat.format(date2));
if (date1.equals(date2)) {
System.out.println("Date-1 is same as Date-2");
}
} catch (ParseException ex) {
}
}
}
Output:
Date-1: 2020-01-25
Date-2: 2020-01-25
Date-1 is same as Date-2
compareTo()
Method to Compare Two Dates in Java
The last approach is compareTo()
method in Java Date
class. It compares two dates and returns different values based on the comparison result.
- It returns
0
if the argument date is equal to aDate
object - It returns a positive value if a
Date
object is after the argument date - It returns a negative if a
Date
object is before the argument date
Example Codes:
// java 1.8
package simpletesting;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
try {
SimpleDateFormat sdformat = new SimpleDateFormat("yyyy-MM-dd");
Date date1 = sdformat.parse("2020-01-25");
Date date2 = sdformat.parse("2020-01-23");
System.out.println("Date-1: " + sdformat.format(date1));
System.out.println("Date-2: " + sdformat.format(date2));
if (date1.compareTo(date2) > 0) {
System.out.println("Date-1 is after Date-2");
} else if (date1.compareTo(date2) < 0) {
System.out.println("Date-1 is before Date-2");
} else if (date1.compareTo(date2) == 0) {
System.out.println("Date-1 is same as Date-2");
}
} catch (ParseException ex) {
}
}
}
Output:
Date-1: 2020-01-25
Date-2: 2020-01-23
Date-1 is after Date-2