How to Get Current TimeStamp in Java Date
-
Get Current Timestamp Using the
Timestamp
Class in Java -
Get Current Timestamp Using the
Date
Class in Java -
Get Current Timestamp Using the
ZonedDateTime
Class in Java -
Get Current Timestamp Using the
LocalDateTime
Class in Java -
Get Current Timestamp Using the
Instant
Class in Java -
Get Current Timestamp Using the
Timestamp
Class in Java -
Get Current Timestamp Using the
toInstant()
Method in Java
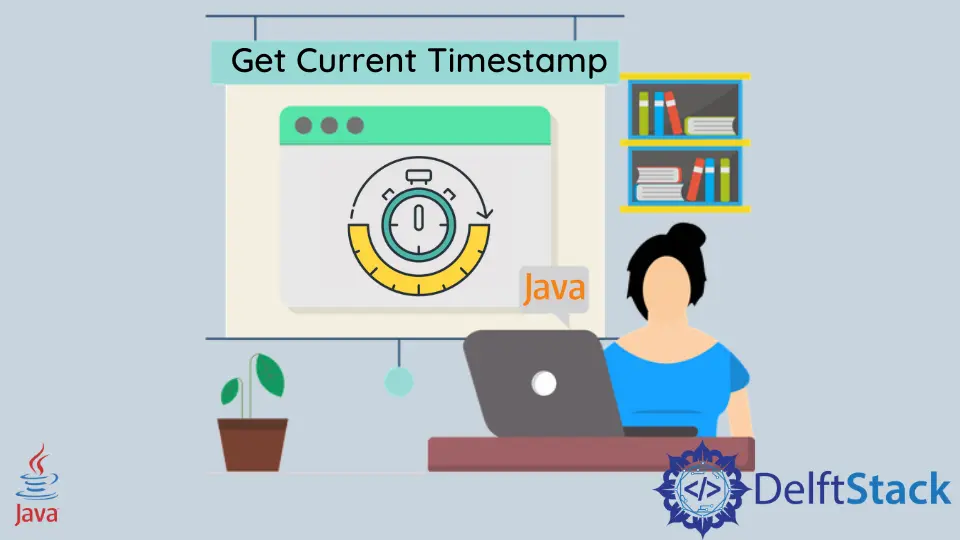
This tutorial introduces how to get the current timestamp in Java and lists some example codes to understand the topic.
There are several ways to get the current timestamp in Java, such as the Timspamp
class, Date
class, ZonedDateTime
class, LocalDateTime
class, etc. Let’s see some examples.
Get Current Timestamp Using the Timestamp
Class in Java
To get the current timestamp in Java, we can use the Timestamp
class. Since this class does not have a default constructor, so we pass the time in milliseconds. We use the currentTimeMillis()
method of System
class to get the time. See the example below.
import java.sql.Timestamp;
public class SimpleTesting {
public static void main(String[] args) {
Long datetime = System.currentTimeMillis();
Timestamp timestamp = new Timestamp(datetime);
System.out.println("Current Time Stamp: " + timestamp);
}
}
Output:
Current Time Stamp: 2021-03-06 17:24:57.107
Get Current Timestamp Using the Date
Class in Java
We can use use the Date
class of the util package to get the current timestamp. To format the timestamp in the yyyy.MM.dd
format, we can use the SimpleDateFormat
class. See the example below.
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
SimpleDateFormat date = new SimpleDateFormat("yyyy.MM.dd.HH:mm:ss");
String timeStamp = date.format(new Date());
System.out.println("Current Time Stamp: " + timeStamp);
}
}
Output:
Current Time Stamp: 2021.03.06.17:26:17
Get Current Timestamp Using the ZonedDateTime
Class in Java
The ZonedDateTime
class of Java datetime
package creates a timestamp with the zone information. We use the systemDefault()
method of ZoneId
to get the system’s default zone and the now()
method to get the current timestamp with the specified zoneId.
After getting the current timestamp, we use the ofPattern()
method of the DateTimeFormatter
class to get the timestamp in a specified format. See the example below.
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String[] args) {
ZoneId zid = ZoneId.systemDefault();
ZonedDateTime datetime = ZonedDateTime.now(zid);
System.out.println("Current Time Stamp: " + datetime);
// if want to format into a specific format
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("uuuu.MM.dd.HH:mm:ss");
String timeStamp = datetime.format(formatter);
System.out.println("Current Time Stamp: " + timeStamp);
}
}
Output:
Current Time Stamp: 2021-03-06T17:35:52.722720362Z[Etc/UTC]
Current Time Stamp: 2021.03.06.17:35:52
Get Current Timestamp Using the LocalDateTime
Class in Java
If you are working with Java 8, use the LocalDateTime
class to get the current timestamp in Java. See the below example.
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String[] args) {
LocalDateTime dateTime = LocalDateTime.now();
System.out.println("Current Time Stamp Default Format: " + dateTime);
String timeStamp = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss").format(dateTime);
System.out.println("Current Time Stamp: " + timeStamp);
}
}
Output:
Current Time Stamp Default Format: 2021-02-27T14:15:46.196564
Current Time Stamp: 2021-02-27 14:15:46
Get Current Timestamp Using the Instant
Class in Java
The Instant
class can be used to get the current timestamp. We use the now()
method of the Instant
class to fetch the current timestamp in instants.
import java.time.Instant;
public class SimpleTesting {
public static void main(String[] args) {
Instant timeStamp = Instant.now();
System.out.println("Current Time Stamp Default Format: " + timeStamp);
}
}
Output:
Current Time Stamp Default Format: 2021-02-27T08:48:28.001913Z
Get Current Timestamp Using the Timestamp
Class in Java
This is another solution to get the current timestamp in Java with the Timestamp
and Instant
class. See the example below.
import java.sql.Timestamp;
import java.time.Instant;
public class SimpleTesting {
public static void main(String[] args) {
long time = System.currentTimeMillis();
Timestamp timestamp = new Timestamp(time);
Instant instant = timestamp.toInstant();
System.out.println("Current Time Stamp: " + instant);
}
}
Output:
Current Time Stamp: 2021-02-27T08:50:05.125Z
Get Current Timestamp Using the toInstant()
Method in Java
If we have a date object representing the timestamp, we can also use the toInstant()
method of the Date
class to get the current timestamp.
import java.time.Instant;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
Date date = new Date();
Instant instant = date.toInstant();
System.out.println("Current Time Stamp: " + instant);
}
}
Output:
Current Time Stamp: 2021-02-27T08:51:34.223Z