在 Java 日期中获取当前时间戳
-
使用 Java 中的
Timestamp
类获取当前时间戳 -
使用 Java 中的
Date
类获取当前时间戳 -
使用 Java 中的
ZonedDateTime
类获取当前时间戳 -
使用 Java 中的
LocalDateTime
类获取当前时间戳 -
使用 Java 中的
Instant
类获取当前时间戳 -
使用 Java 中的
Timestamp
类获取当前时间戳 -
使用 Java 中的
toInstant()
方法获取当前时间戳
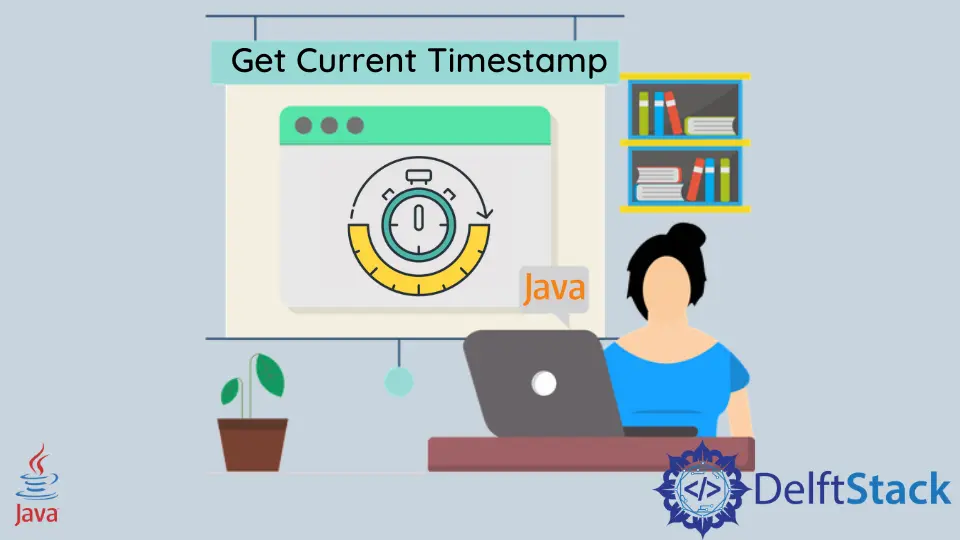
本教程介绍如何获取 Java 中的当前时间戳,并列出一些示例代码以了解该主题。
有几种获取 Java 当前时间戳的方法,例如 Timspamp
类,Date
类,ZonedDateTime
类,LocalDateTime
类等。让我们来看一些示例。
使用 Java 中的 Timestamp
类获取当前时间戳
要获取 Java 中的当前时间戳,可以使用 Timestamp
类。由于此类没有默认的构造函数,因此我们以毫秒为单位传递时间。我们使用 System
类的 currentTimeMillis()
方法获取时间。请参见下面的示例。
import java.sql.Timestamp;
public class SimpleTesting {
public static void main(String[] args) {
Long datetime = System.currentTimeMillis();
Timestamp timestamp = new Timestamp(datetime);
System.out.println("Current Time Stamp: " + timestamp);
}
}
输出:
Current Time Stamp: 2021-03-06 17:24:57.107
使用 Java 中的 Date
类获取当前时间戳
我们可以使用 util 包的 Date
类来获取当前时间戳。要以 yyyy.MM.dd
格式格式化时间戳,我们可以使用 SimpleDateFormat
类。请参见下面的示例。
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
SimpleDateFormat date = new SimpleDateFormat("yyyy.MM.dd.HH:mm:ss");
String timeStamp = date.format(new Date());
System.out.println("Current Time Stamp: " + timeStamp);
}
}
输出:
Current Time Stamp: 2021.03.06.17:26:17
使用 Java 中的 ZonedDateTime
类获取当前时间戳
Java datetime
包的 ZonedDateTime
类创建带有区域信息的时间戳。我们使用 ZoneId
的 systemDefault()
方法获取系统的默认区域,并使用 now()
方法获取具有指定 zoneId 的当前时间戳。
获取当前时间戳后,我们使用 DateTimeFormatter
类的 ofPattern()
方法来获取指定格式的时间戳。请参见下面的示例。
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String[] args) {
ZoneId zid = ZoneId.systemDefault();
ZonedDateTime datetime = ZonedDateTime.now(zid);
System.out.println("Current Time Stamp: " + datetime);
// if want to format into a specific format
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("uuuu.MM.dd.HH:mm:ss");
String timeStamp = datetime.format(formatter);
System.out.println("Current Time Stamp: " + timeStamp);
}
}
输出:
Current Time Stamp: 2021-03-06T17:35:52.722720362Z[Etc/UTC]
Current Time Stamp: 2021.03.06.17:35:52
使用 Java 中的 LocalDateTime
类获取当前时间戳
如果你使用的是 Java 8,请使用 LocalDateTime
类获取 Java 中的当前时间戳。请参见以下示例。
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String[] args) {
LocalDateTime dateTime = LocalDateTime.now();
System.out.println("Current Time Stamp Default Format: " + dateTime);
String timeStamp = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss").format(dateTime);
System.out.println("Current Time Stamp: " + timeStamp);
}
}
输出:
Current Time Stamp Default Format: 2021-02-27T14:15:46.196564
Current Time Stamp: 2021-02-27 14:15:46
使用 Java 中的 Instant
类获取当前时间戳
Instant
类可用于获取当前时间戳。我们使用 Instant
类的 now()
方法来即时获取当前时间戳。
import java.time.Instant;
public class SimpleTesting {
public static void main(String[] args) {
Instant timeStamp = Instant.now();
System.out.println("Current Time Stamp Default Format: " + timeStamp);
}
}
输出:
Current Time Stamp Default Format: 2021-02-27T08:48:28.001913Z
使用 Java 中的 Timestamp
类获取当前时间戳
这是通过 Timestamp
和 Instant
类获取 Java 中当前时间戳的另一种解决方案。请参见下面的示例。
import java.sql.Timestamp;
import java.time.Instant;
public class SimpleTesting {
public static void main(String[] args) {
long time = System.currentTimeMillis();
Timestamp timestamp = new Timestamp(time);
Instant instant = timestamp.toInstant();
System.out.println("Current Time Stamp: " + instant);
}
}
输出:
Current Time Stamp: 2021-02-27T08:50:05.125Z
使用 Java 中的 toInstant()
方法获取当前时间戳
如果我们有一个表示时间戳的日期对象,我们还可以使用 Date
类的 toInstant()
方法来获取当前的时间戳。
import java.time.Instant;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
Date date = new Date();
Instant instant = date.toInstant();
System.out.println("Current Time Stamp: " + instant);
}
}
输出:
Current Time Stamp: 2021-02-27T08:51:34.223Z