How to Get Current Timestamp in ISO 8601 Format
- Importance and Usage of the ISO 8601 Format in Java
-
Use of
T
andZ
in the ISO 8601 Format - Convert Current Timestamp to ISO 8601 Using the UTC Time Zone
- Advantages of Using the ISO 8601 Format
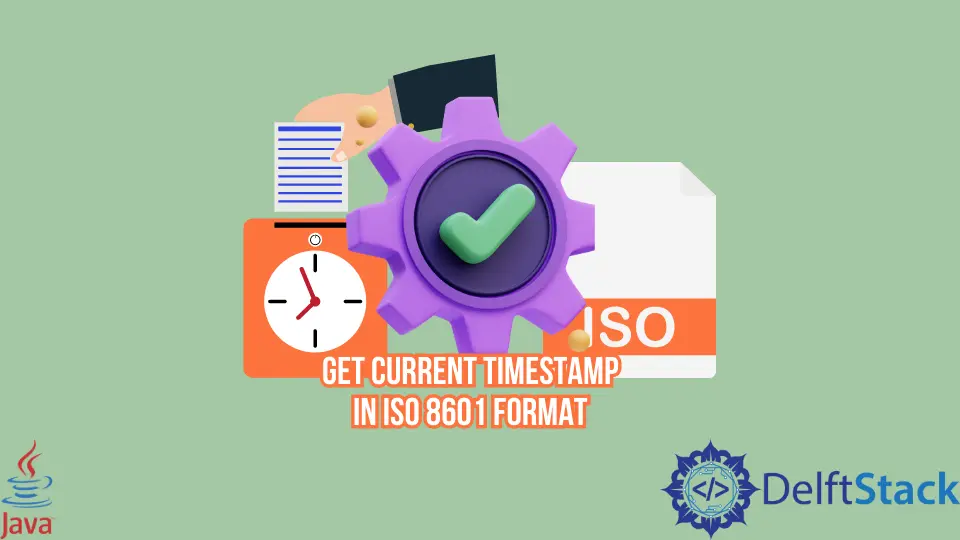
In Java or any other programming language, converting a string to date is one of the fundamental skills. It is pretty straightforward to store a date in string format and then convert it into the Date
object to move ahead in the project.
This article will teach us how to get the current timestamp in the ISO 8601 format. Let’s start learning the ISO 8601 format and why and how we can use it in our project.
Importance and Usage of the ISO 8601 Format in Java
Date and Time formats are specifically important when we have to arrange conferences and meetings or deal with our customers. However, in this digital era, it doesn’t seem easy to have a standard format to specify different time zones worldwide that is easy to understand for everyone.
The international standard ISO 8601 format is the one that precisely solves this problem. Using the international organization for standardization (ISO) date/time format, we can specify a numerical date/time where time is optional.
All the stakeholders across the borders can specify the date/time using this international standard. Remember that the ISO 8601 date representation is based on the Gregorian Calendar.
In the ISO 8601 format, we specify a date/time starting with a year, followed by a month, day, hour, minutes, seconds, and milliseconds.
For instance, 2022-09-30 15:00:00.000
shows the 30th of September 2022 at 3 PM (this timestamp is in local time as we have not specified the zone offset, which we will learn later in this tutorial). Following is the tabular representation of the date/time using ISO 8601.
ISO 8601 Format | Value Range |
---|---|
Year (Y ) |
YYYY , four digits. We can abbreviate it to two digits as well. |
Month (M ) |
MM , 01-12 |
Week (W ) |
WW , 01-53 |
Day (D ) |
D , week’s day, 1-7 |
Hour (h ) |
hh , 00-23 where 24:00:00 as an end time |
Minutes (m ) |
mm , 00-59 |
Seconds (s ) |
ss , 00-59 |
Decimal Fraction (f ) |
seconds’ fractions, any degree of accuracy |
Use of T
and Z
in the ISO 8601 Format
Suppose we have a timestamp 2022-09-30T20:18:46.384Z
. Here, T
does not stand for anything; it is just a separator required by the ISO 8601 date/time format.
We can read T
as an abbreviation of Time while Z
is used for Zero timestamps because it’s offset by 0 from a UTC (Coordinated Universal Time).
Let’s understand the use of T
and Z
via the following examples.
2022-09-30T15:50+00
- 3:30 PM on September 30, 2022, in a universal time zone.2022-09-30T15:50+00Z
- 3:30 PM on September 30, 2022, in a time zone of universal time with an addition ofZ
in the notation.2022-09-30T15:50-04:00
- 3:30 PM on September 30, 2022, in a time zone in New York (UTC with daylight saving time).
We have learned enough about the ISO 8601 date format. Let’s see how we can do it in Java and get the current date/time in the UTC.
Convert Current Timestamp to ISO 8601 Using the UTC Time Zone
Example Code:
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
public class Test {
public static void main(String args[]) {
TimeZone timezone = TimeZone.getTimeZone("UTC");
//"Z" indicates UTC, which means no timezone offset
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
dateFormat.setTimeZone(timezone);
String ISODate = dateFormat.format(new Date());
System.out.println(ISODate);
}
}
Output:
2022-09-30T07:20:52Z
In the main()
method of Test
, we first use the getTimeZone()
method, which takes a string-type argument to get a time zone. This method returns a time zone associated with a calendar of DateFormat
.
Then, we use the SimpleDateFormat
class, which helps to parse and format data. It helps us to transform the date from one format to another.
We instantiate this class by specifying our required date/time format.
Next, we set the time zone using the setTimeZone()
method and pass a parameter that holds the value returned by the getTimeZone()
method. After that, we use the dateFormat
object to format the given date.
Finally, we print the date, which is now in the ISO 8601 format (see the output given above).
Now, the question is, why are we using this specific format? Do we have some advantages for it? Let’s have a look at them below.
Advantages of Using the ISO 8601 Format
Following are a few advantages of using the ISO 8601 format over other common variants:
- It is easy to read/write with software.
- It is easy to compare/sort with string comparison.
- Strings, where the date is followed by time, are easy to compare/sort. For instance,
2022-09-30 10:15:00
. - It is easy to understand regardless of the national language and can’t be confused with other commonly used date variants.
- Notation has constant length and is concise. Additionally, a four-digit year doesn’t cause any issues at the turn of the century.
- This date format
year-month-day
is widespread, for instance, in Korea, Hungary, Sweden, Japan, and other countries.
This format is identical to the Chinese date representation, meaning almost 25% are already familiar with this system.
As we can see, using the ISO 8601 format has multiple advantages and is also easy to use and understand. So, learning and using this date format in applications is important.