Gregorian Calendar Class in Java
-
Java.util.Calendar
Class -
java.util.Locale
Class -
Difference Between
GregorianCalendar()
Class andCalendar
Class in Java - Implementation of Gregorian Calendar Class in Java
- Summary
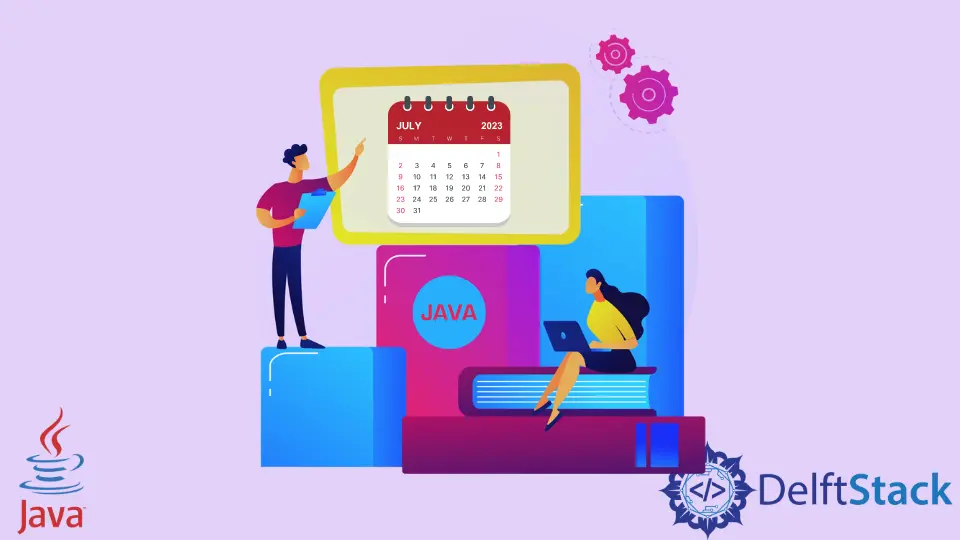
This article will show you how to use Java’s Gregorian calendar system. It is a specific subclass of the Calendar
class.
In addition, it offers the most widely used calendar system in the world. Besides, it is a hybrid calendar that is interoperable with the Julian and Gregorian calendar standards.
Java.util.Calendar
Class
The Calendar
class is an abstract class that contains methods for converting between a specific point in time and a collection of calendar fields such as YEAR
, MONTH
, DAY OF MONTH
, HOUR
, WEEK_OF_YEAR
, ZONE_OFFSET
, FIELD_COUNT
, MILLISECOND
and a lot of others.
Also, it allows users to modify the calendar fields, such as fetching the following week’s date.
Calendar
class object is created.Syntax:
Calendar ModifyCal = Calendar.getInstance();
java.util.Locale
Class
A Locale
object denotes a particular geographic, political, or cultural territory. A locale-sensitive action requires a Locale
to perform its task.
It also applies the Locale
to customize information for the user.
Difference Between GregorianCalendar()
Class and Calendar
Class in Java
The difference is quite simple to understand. As you already know that when we use Calendar
in most programs, it constructs a Gregorian Calendar object: getInstance()
.
There are, nevertheless, a few outliers. Notably, the location/region you can set to display a particular calendar system based on your region.
In other words, suppose you need your Calendar
to be a GregorianCalendar()
. In that case, all you need to do is to write a new: GregorianCalendar()
.
However, we recommend letting Java Virtual Machine decide what region to choose by default. You must use the word Calendar.getInstance()
and be ready to deal with the potential of selecting another calendar type.
Some Notable Calendar
Functions in Java
To begin with, These methods and their types are helpful. Note that there are a wide variety of methods besides them.
It would help if you opened the java.util.GregorianCalendar
class or the calendar
class files to study.
S.N | Type | Function Syntax | Summary |
---|---|---|---|
1 | TimeZone |
getTimeZone() |
This function get you the current time zone. |
2 | int |
getWeekYear() |
It simply returns you the week year according to the Gregorian Calendar. |
3 | void |
setTimeZone |
You can add the value to set the time zone with this method. |
4 | void |
setWeekDate |
It changes the GregorianCalendar to the date specified by the date specifiers. |
5 | boolean |
isWeekDateSupported() |
It only returns true, showing that the class supports specific week dates. |
6 | void |
setGregorianChange(Date date) |
You can modify the date with this function. |
7 | int |
hashCode() |
It will give you a hash code object for this Gregorian calendar. |
the Importance of Calendar
Constructors in Java
These constructors help you extend the default class structure and modify locale, date, time, zone and other functional parameters that you want to customize. In most programs, we do not want default solutions, and sometimes, we want to try something of our own.
On the contrary, our client’s preferences regarding the format might be different. So, we highly recommend studying these functions.
S.N | Function Name & Syntax | Summary |
---|---|---|
1 | GregorianCalendar() |
Constructs a default format for the region and the current time in the default region. |
2 | GregorianCalendar(TimeZone zone) |
Based on the current time in the provided time zone. |
3 | GregorianCalendar(TimeZone zone, Locale aLocale) |
Based on the current time in the supplied time zone and region. |
4 | GregorianCalendar(int year, int month, int dayOfMonth) |
Constructs a GregorianCalendar with the specified date set in the default time zone and locale. |
5 | GregorianCalendar(int year, int month, int dayOfMonth, int hourOfDay, int minute) |
Constructs a GregorianCalendar with the specified date and time for the default time zone and region. |
Implementation of Gregorian Calendar Class in Java
A simple program to print today’s date using getTime()
.
Example 1:
package delftstack.calendar.com.util;
import java.util.Date;
import java.util.GregorianCalendar;
// A simple program to print today's date using getTime();
public class Example1 {
public static void main(String[] args) {
GregorianCalendar printtodaydate = new GregorianCalendar();
Date defualt = printtodaydate.getTime();
System.out.println(defualt);
}
}
Output:
Mon Mar 28 04:50:00 PDT 2022
Example 2: Get the current day of the week.
package delftstack.calendar.com.util;
import java.text.SimpleDateFormat;
import java.util.GregorianCalendar;
// get current day of the weak
public class Example2 {
public static void main(String[] args) {
GregorianCalendar DOW = new GregorianCalendar();
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
String dayOfWeek = formatter.format(DOW.getTime());
System.out.println(dayOfWeek);
}
}
Output:
Monday
Example 3: Modify the current date after 100 years on this day.
package delftstack.calendar.com.util;
import java.util.Calendar;
import java.util.GregorianCalendar;
// modify current date
// after 100 years on this day
public class Example3 {
public static void main(String[] args) {
GregorianCalendar gregorianCalendar = new GregorianCalendar();
java.util.Date currentdate = gregorianCalendar.getTime();
gregorianCalendar.set(Calendar.YEAR, gregorianCalendar.get(Calendar.YEAR) + 100);
System.out.println("It is: " + currentdate + " today");
System.out.println("After 100 year on today: " + gregorianCalendar.getTime());
}
}
Output:
It is: Mon Mar 28 04:53:53 PDT 2022 today
After 100 year on today: Sat Mar 28 04:53:53 PDT 2122
Example 4: Reformat the calendar date as you want YY-MMM-dd
.
package delftstack.calendar.com.util;
import java.text.SimpleDateFormat;
import java.util.GregorianCalendar;
public class Example4 {
// reformat calendar date as you want
// YY-MMM-dd
public static void main(String[] args) {
GregorianCalendar gregorianCalendar = new GregorianCalendar();
SimpleDateFormat reformat = new SimpleDateFormat("YY/MM/dd");
String newformat = reformat.format(gregorianCalendar.getTime());
System.out.println(newformat);
}
}
Output:
22/03/28
Example 5: What is my current time zone?
package delftstack.calendar.com.util;
// what is my current time zone
import java.util.GregorianCalendar;
public class Example5 {
public static void main(String[] args) {
// Create a new calendar
GregorianCalendar update = (GregorianCalendar) GregorianCalendar.getInstance();
System.out.println("What is my current time zone? ");
System.out.println("Your time zone is: " + update.getTimeZone().getDisplayName());
}
}
Output:
What is my current time zone?
Your time zone is: Pacific Standard Time
Example 6: Set my current time zone to GMT
.
package delftstack.calendar.com.util;
import java.util.GregorianCalendar;
import java.util.TimeZone;
public class Example6 {
public static void main(String[] args) {
// Create a new cal class
GregorianCalendar setnewtimezone = (GregorianCalendar) GregorianCalendar.getInstance();
// Convert to another time zone
setnewtimezone.setTimeZone(TimeZone.getTimeZone("GMT"));
System.out.println("Set my current time zone to GMT");
setnewtimezone.getTimeZone().getDisplayName();
System.out.println("Now print it to confirm updated time zone: "
+ setnewtimezone.getTimeZone().getDisplayName());
}
}
Output:
Set my current time zone to GMT
Now print it to confirm the updated time zone: Greenwich Mean Time
Example 7: Show date using Java calendar
class.
package delftstack.calendar.com.util;
import java.util.Calendar;
public class ShowDateWithJavaCalendarClass {
public static void main(String[] args) {
// Make an obj of Cal Class
Calendar ModCalendarclass = Calendar.getInstance();
System.out.println(
"A simple program to show date using java calendar class: " + ModCalendarclass.getTime());
} // function
} // class
Example 8: Show date using Java GregorianCalendar
class.
package delftstack.calendar.com.util;
import java.util.GregorianCalendar;
public class ShowDateWithJavaGreogorianCalendarClass {
public static void main(String[] args) {
// use gregorian calendar class
GregorianCalendar ModGg = new GregorianCalendar();
// display date with grogerian
System.out.print(
"A simple program to show date using java gregorian calendar class: " + ModGg.getTime());
}
}
Use Constructor on GregorianCalendar
Class in Java
Example 9: Show date using Java GregorianCalendar
class.
package delftstack.calendar.com.util;
import java.util.Calendar;
import java.util.GregorianCalendar;
import java.util.Locale;
// creating GregorianCalendarExample1 to define default constructor
public class CustomizeCalUsingDefaultConstructor {
// main() method start
public static void main(String args[]) {
// Make an array that contains months
String setmonths[] = {
"Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"};
// Array for the AM, PM
String ampm[] = {"AM", "PM"};
// Create GregorianCalendar and use it as constructor
GregorianCalendar ModGg = new GregorianCalendar();
// Print date, time, others
System.out.println("Current Date: " + ModGg.get(Calendar.DATE));
System.out.println("Current Month: " + setmonths[ModGg.get(Calendar.MONTH)]);
System.out.println("Current Year: " + ModGg.get(Calendar.YEAR));
System.out.println("Current Time: " + ModGg.get(Calendar.HOUR) + ":"
+ ModGg.get(Calendar.MINUTE) + " " + ModGg.get(Calendar.SECOND) + " "
+ ampm[ModGg.get(Calendar.AM_PM)]);
System.out.println("Region: " + ModGg.getTimeZone().getDisplayName());
System.out.println("Region: " + Locale.getDefault().getDisplayName());
}
}
Output:
Current Date: 28
Current Month: Mar
Current Year: 2022
Current Time: 5:7 15 AM
Region: Pacific Standard Time
Region: English (United States)
Summary
You can edit the default parameters of the class GregorianCalendar
in Java or the class calendar
in any way you wish. Implementing time, date, zone, locale, and other adjustments is relatively simple for newbies.
We hope you now understand how to use the Gregorian calendar in Java. Please continue to adjust and practice what you have learned thus far.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn