Calendar Date in YYYY-MM-DD Format in Java
-
Convert Date to
YYYY-MM-DD
Format in Java -
Format the Date to
YYYY-MM-DD
Format in Java -
Use the
SimpleDateFormat
Class in Java - Conclusion
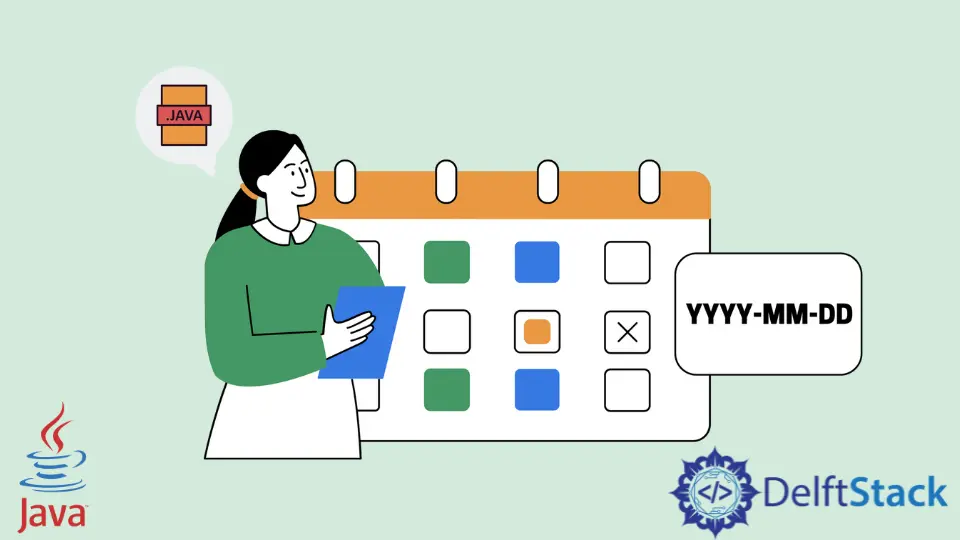
The Java Date
encapsulates the current time and date. The date class does that with the help of two constructors - the Date()
and Date(long millisec)
constructors.
We use the Date()
constructor to initialize the object with the current time and date. On the other hand, the Date(long millisec)
constructor takes the number of milliseconds since midnight, January 1, 1970
, as an argument.
But the output we get is not in the yyyy-MM-dd
format. This article will show how we can produce the output in this format.
We use the date()
method in Java to get the current date and time. To do it, we use a Date object with the toString()
method. Look at the example below to understand it.
import java.util.Date;
public class DateExample {
public static void main(String args[]) {
// Create a date object
Date date_of_today = new Date();
// Use toString() to print the date
System.out.println(date_of_today.toString());
}
}
Output:
Mon Jan 10 09:58:43 UTC 2022
This example uses the date_of_today
to instantiate the DDate object and the toString()
method to print the date. Note that the output we get contains the day, month, date, and year and the time in hours:minutes:seconds
format.
This poses a problem when we work with two or more dates. To remove the name of the day and time from this output, we can use the printf()
method in two ways. The first one uses the symbols %
and $
.
Look at the example below.
import java.util.Date;
public class DateExample {
public static void main(String args[]) {
// Create a new object
Date date_of_today = new Date();
// Display the date
System.out.printf("%1$s %2$tB %2$td, %2$tY", "Date:", date_of_today);
}
}
Output:
Date: January 10, 2022
The second way is to make use of the <
flag. Look at the same example again.
import java.util.Date;
public class DateExample {
public static void main(String args[]) {
// Create a new object
Date date_of_today = new Date();
// display the date
System.out.printf("%s %tB %<te, %<tY", "Date:", date_of_today);
}
}
Output:
Date: January 10, 2022
Note that in these examples, the time is omitted. But we still don’t get the output in the yyyy-MM-dd
format. Let us see how we can deal with this issue.
Convert Date to YYYY-MM-DD
Format in Java
Java has a java.time
package. Instead of using the date class to work with the date and time, we can use the java.time
package. Let us look at an example first.
// import the java.time package
import java.time.LocalDate;
public class DemoOfDate {
public static void main(String[] args) {
// create an object for date
LocalDate date_of_today = LocalDate.now();
// Display the date
System.out.println(date_of_today);
}
}
Output:
2022-01-10
We use the java.time
package and the LocalDate
class in this example. This time we get the output in yyyy-MM-dd
format. The java.time
package has four main classes.
LocalDate
- It gives a date in year, month, day, oryyyy-MM-dd
format as the output.LocalTime
- It gives the output in time and nanoseconds asHH-mm-ss-ns
.LocalDateTime
- It gives the output in both the date and time asyyyy-MM-dd-HH-mm-ss-ns
.DateTimeFormatter
- This acts as a formatter for date-time objects and is used to display and parse them.
Note that we are using the LocalDate
class in the above example. Moreover, we make use of the now()
method. The now()
method in java is a method of the class LocalTime
. It gets the current time in the default timezone from a system clock.
Syntax:
public static LocalTime now()
We do not pass any parameter in the now()
method. One more way is to get the output in yyyy-MM-dd
format. Let us look at that too. Here is the link to the documentation.
Format the Date to YYYY-MM-DD
Format in Java
To change the calendar date to the yyyy-MM-dd
format, we can also use the concept of formatting. It makes working with the date and time much more flexible.
To do this, we use the ofPattern()
method present in the java.time
package. The various ways we can get the output using the ofPattern()
method are as follows.
yyyy-MM-dd
- This gives the output as2022-01-25
dd/MM/yyyy
- This gives the output as25/01/2022
dd-MMM-yyyy
- This gives the output as25/01/2022
E, MMM dd yyyy
- This gives the output asTue, Jan 25 2022
Let us look at an example.
// import the LocalDateTime class
import java.time.LocalDateTime;
// import the DateTimeFormatter class
import java.time.format.DateTimeFormatter;
public class DemoOfDate {
public static void main(String[] args) {
LocalDateTime date_of_today = LocalDateTime.now();
System.out.println("Output before formatting: " + date_of_today);
DateTimeFormatter format_date_of_today = DateTimeFormatter.ofPattern("yyyy-MM-dd");
String formattedDate = date_of_today.format(format_date_of_today);
System.out.println("Output after formatting: " + formattedDate);
}
}
Output:
Output before formatting: 2022-01-01T10:46:21.449669400
Output after formatting: 2022-01-01
Note that we can get the output in the yyyy-MM-dd
format by using formatting. We can also move around the values of all these formats.
For example, when we pass the string yyyy-MM-dd
in the ofPattern()
method, we can change that string to yyyy-dd-MM
or dd-MM-yyyy
or in any other way as per the need. This applies to all the formats provided by the ofPattern()
method.
We use one more method here - the format()
method. The format()
method formats a string by the given locale, arguments, and format in Java.
If we do not specify the locale, the format()
method calls the Locale.getDefault()
method to get the default locale. The format()
method works just like the printf()
function.
Use the SimpleDateFormat
Class in Java
To get the output in yyyy-MM-dd
format, we can use the SimpleDateFormat
class. It provides methods for formatting (changing the date to string) and parsing (changing string to date) the date and time in Java.
By inheriting it, it works with the java.text.DateFormat
class. Let us look at an example.
import java.text.SimpleDateFormat;
import java.util.Date;
public class SimpleDateFormatDemo {
public static void main(String[] args) {
Date date_of_today = new Date();
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
String stringDate = format.format(date_of_today);
System.out.println(stringDate);
}
}
Output:
2022-01-01
To know more about the SimpleDateFormat
class in Java, refer to this documentation.
Conclusion
This article discussed how we could change the calendar date to the yyyy-MM-dd
format in Java. These include the use of the java.time
package, formatting using the toPattern()
method and the SimpleDateFormat
class. The various methods are the toString()
and now()
methods.
We can get the output in yyyy-MM-dd
format and use it when more than a single date is included.
Related Article - Java Date
- How to Find the Day of the Week Using Zellers Congruence in Java
- How to Get Day of Week in Java
- Java Date vs. LocalDate
- How to Set Time Zone of a java.util.Date
- How to Convert String to LocalDate in Java