How to Get Day of Week in Java
Sheeraz Gul
Feb 02, 2024
Java
Java Date
- Java Get Day of Week
-
Get the Day of the Week Using Java 7
Calendar
Class - Get the Day of the Week Using Java 8 Date API
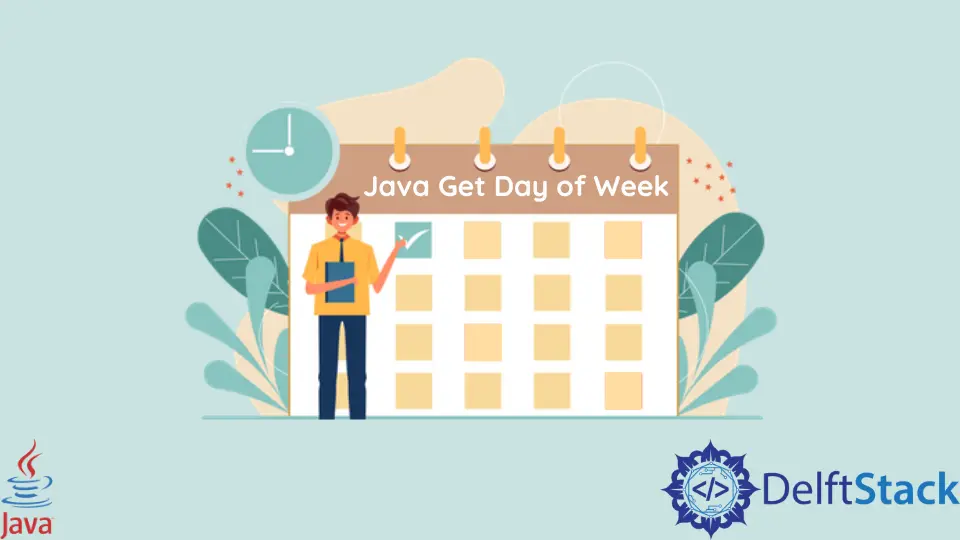
This tutorial demonstrates how to get the day of the Week in Java.
Java Get Day of Week
Sometimes there is a requirement to get the day of a week while working with UI. This can be achieved in Java with the following two methods:
- From Java 7, the legacy
Calendar
class can be used, which defines the constants from SUNDAY (1) to SATURDAY (7). We can get the day using the methodcalendar.get(Calendar.DAY_OF_WEEK)
on the instance of the calendar. - From Java 8, the legacy API Date can be used to get the day of a week from Monday (1) to SUNDAY (7). The method
LocalDate.getDayOfWeek()
can be used to get the day of the week.
Get the Day of the Week Using Java 7 Calendar
Class
The Java 7 Calendar
class is used to get the day of the week. For that, we need to use java.util.Date
and java.util.Calendar
.
Follow the steps below:
-
Create an instance of the
Calendar
class. -
Set the date in calendar using the
setTime(new Date())
method, which will set the current date. -
Now use
get(Calendar.DAY_OF_WEEK)
on the calendar instance to get the day’s number of the week. -
We need to use the
SimpleDateFormat()
Class to get the name of the day.
Let’s implement the above example in Java:
package delftstack;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class Example {
public static void main(String[] args) {
Calendar Demo_Calendar = Calendar.getInstance();
Demo_Calendar.setTime(new Date());
int Day_Number = Demo_Calendar.get(Calendar.DAY_OF_WEEK);
DateFormat Date_Formatter = new SimpleDateFormat("EEEE");
String Day_Name = Date_Formatter.format(Demo_Calendar.getTime());
System.out.println("The current day of the Week in number is :: " + Day_Number);
System.out.println("The current day of the Week in Text is :: " + Day_Name);
}
}