Java Date vs. LocalDate
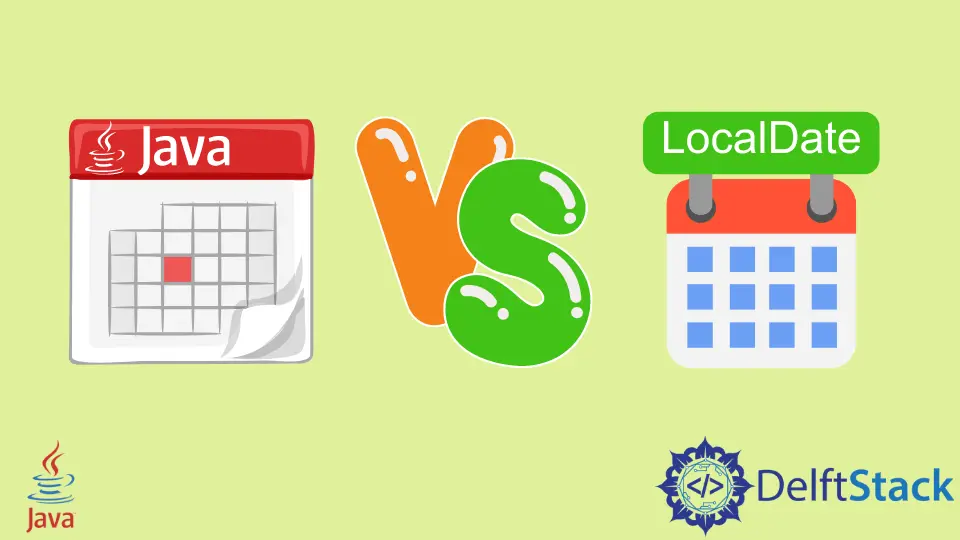
Handling dates and times is an essential part of many applications. Java provides several classes and APIs to work with dates and times, and two commonly used classes for this purpose are Date
and LocalDate
.
While both are used to represent dates, they have significant differences in terms of functionality, usage, and compatibility with modern Java best practices. This tutorial demonstrates the difference between Date
and LocalDate
in Java.
Java Date
Class
The java.util.Date
class has been part of the Java platform since the early versions. It represents an instant in time, typically in milliseconds since the epoch (January 1, 1970, 00:00:00 GMT).
However, Date
also contains time information down to milliseconds, making it a precise representation of both date and time.
Here’s a simple example of creating a Date
instance:
import java.util.Date;
public class DateExample {
public static void main(String[] args) {
Date currentDate = new Date();
System.out.println("Current date and time: " + currentDate);
}
}
Output:
Current date and time: Fri Sept 29 03:28:47 GMT 2023
First, it imports the Date
class from the java.util
package. Inside the main
method, an instance of the Date
class is created and assigned to the variable currentDate
using Date currentDate = new Date();
.
This instance represents the current date and time at the moment of creation. The System.out.println
statement is then used to output a message along with the current date and time, achieved by concatenating the string "Current date and time: "
with the currentDate
variable.
Finally, the program prints this message to the standard output, providing the current date and time when executed.
However, it is important to note that the Date
class has several drawbacks:
- The
Date
class is mutable, meaning its value can be changed after instantiation. This can lead to unexpected behavior if not handled carefully. - The
Date
class doesn’t handle time zones well. It represents the date and time in UTC (Coordinated Universal Time) and relies on the system’s default time zone for display purposes. Date
includes millisecond precision, which may be unnecessary for applications that only need date information.
Let’s have another example to better understand the Date
class in Java:
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.TimeZone;
public class Example {
public static void main(String[] args) {
Instant Date_Instant = new Date().toInstant();
LocalDateTime Demo_Date =
LocalDateTime.ofInstant(Date_Instant, ZoneId.of(ZoneId.SHORT_IDS.get("PST")));
System.out.println("The Current Date is: " + Demo_Date);
Instant Calendar_Instant = Calendar.getInstance().toInstant();
System.out.println(Calendar_Instant);
ZoneId Default_TimeZone = TimeZone.getDefault().toZoneId();
System.out.println(Default_TimeZone);
ZonedDateTime Gregorian_Calendar_DateTime = new GregorianCalendar().toZonedDateTime();
System.out.println(Gregorian_Calendar_DateTime);
Date Date_Demo = Date.from(Instant.now());
System.out.println(Date_Demo);
TimeZone Time_Zone = TimeZone.getTimeZone(Default_TimeZone);
System.out.println(Time_Zone);
GregorianCalendar gc = GregorianCalendar.from(Gregorian_Calendar_DateTime);
System.out.println(gc);
}
}
Output:
The Current Date is: 2022-09-13T00:40:09.373
2022-09-13T07:40:09.490Z
Asia/Karachi
2022-09-13T12:40:09.545+05:00[Asia/Karachi]
Tue Sep 13 12:40:09 PKT 2022
sun.util.calendar.ZoneInfo[id="Asia/Karachi",offset=18000000,dstSavings=0,useDaylight=false,transitions=12,lastRule=null]
java.util.GregorianCalendar[time=1663054809545,areFieldsSet=true,areAllFieldsSet=true,lenient=true,zone=sun.util.calendar.ZoneInfo[id="Asia/Karachi",offset=18000000,dstSavings=0,useDaylight=false,transitions=12,lastRule=null],firstDayOfWeek=2,minimalDaysInFirstWeek=4,ERA=1,YEAR=2022,MONTH=8,WEEK_OF_YEAR=37,WEEK_OF_MONTH=3,DAY_OF_MONTH=13,DAY_OF_YEAR=256,DAY_OF_WEEK=3,DAY_OF_WEEK_IN_MONTH=2,AM_PM=1,HOUR=0,HOUR_OF_DAY=12,MINUTE=40,SECOND=9,MILLISECOND=545,ZONE_OFFSET=18000000,DST_OFFSET=0]
Let’s break down the provided Java code above:
Instant Date_Instant = new Date().toInstant();
LocalDateTime Demo_Date =
LocalDateTime.ofInstant(Date_Instant, ZoneId.of(ZoneId.SHORT_IDS.get("PST")));
System.out.println("The Current Date is: " + Demo_Date);
The program starts by creating an Instant
from the current Date using the toInstant()
method. This Instant
represents a point on the timeline.
Next, the Instant
is converted to a LocalDateTime
, which is a date and time representation without a specific time zone, using the ofInstant()
method, specifying the ZoneId
for Pacific Standard Time (PST). Finally, the resulting LocalDateTime
is printed to the console.
Instant Calendar_Instant = Calendar.getInstance().toInstant();
System.out.println(Calendar_Instant);
Here, the program obtains the current date and time as an Instant
from the default Calendar
instance using the toInstant()
method. The Instant
is then printed to the console.
ZoneId Default_TimeZone = TimeZone.getDefault().toZoneId();
System.out.println(Default_TimeZone);
This step involves converting the default TimeZone
to a ZoneId
using the toZoneId()
method. ZoneId
is part of the modern java.time
package and represents a time zone. The resulting ZoneId
is then printed.
ZonedDateTime Gregorian_Calendar_DateTime = new GregorianCalendar().toZonedDateTime();
System.out.println(Gregorian_Calendar_DateTime);
A ZonedDateTime
instance is created from the current date and time using the toZonedDateTime()
method on a GregorianCalendar
. ZonedDateTime
represents a date, time, and time zone. The resulting ZonedDateTime
is printed.
Date Date_Demo = Date.from(Instant.now());
System.out.println(Date_Demo);
In this step, a Date
instance is created from the current Instant
using the Date.from()
method. This is an example of bridging between the modern java.time
classes and the legacy java.util.Date
class.
TimeZone Time_Zone = TimeZone.getTimeZone(Default_TimeZone);
System.out.println(Time_Zone);
The default ZoneId
is converted to a TimeZone
, a legacy class, using TimeZone.getTimeZone()
. This is another example of interoperability between the modern and legacy date and time classes.
GregorianCalendar gc = GregorianCalendar.from(Gregorian_Calendar_DateTime);
System.out.println(gc);
Here, a GregorianCalendar
instance is created from a ZonedDateTime
instance using the GregorianCalendar.from()
method. GregorianCalendar
is a legacy class, and this operation demonstrates how to convert to legacy date and time representations when needed.
Java LocalDate
Class
On the other hand, the java.time.LocalDate
class was introduced in Java 8 as part of the java.time
package. It represents a date without any time or timezone information.
This makes it suitable for applications that only need to work with dates. Creating a LocalDate
instance is straightforward:
import java.time.LocalDate;
public class LocalDateExample {
public static void main(String[] args) {
LocalDate currentDate = LocalDate.now();
System.out.println("Current date: " + currentDate);
}
}
Output:
Current date: 2023-09-29
First, the code imports the LocalDate
class from the java.time
package.
Inside the main
method, an instance of the LocalDate
class is created using LocalDate.now()
, which represents the current date without the time component. This instance is assigned to the variable currentDate
.
Next, the program uses the System.out.println
statement to output a message that includes the current date. The message is created by concatenating the string "Current date: "
with the currentDate
variable.
Finally, when the program is executed, it prints this message to the standard output, providing the current date in the format Year-Month-Day
. The LocalDate.now()
function ensures that the program obtains the current date dynamically each time it is run.
The key advantages of using LocalDate
are:
LocalDate
is immutable, ensuring that once created, its value cannot be changed. This promotes better code stability and avoids unexpected modifications.- As
LocalDate
does not store time or timezone information, it’s not affected by changes in time zones, making it more reliable for date-based operations. LocalDate
is designed specifically for representing dates without the complexity of time information, making the code more readable and easier to maintain.
Let’s try another example for LocalDate
in Java.
import java.time.LocalDate;
import java.time.Month;
import java.time.ZoneId;
public class Example {
public static void main(String[] args) {
LocalDate Current_LocalDate = LocalDate.now();
System.out.println("The Current Date is: " + Current_LocalDate);
LocalDate FirstLocalDate_2022 = LocalDate.of(2022, Month.JANUARY, 1);
System.out.println("The Specific Date with inputs: " + FirstLocalDate_2022);
LocalDate LocalDate_Karachi = LocalDate.now(ZoneId.of("Asia/Karachi"));
System.out.println("The Current Date in Karachi is: " + LocalDate_Karachi);
LocalDate LocalDate_FromBase = LocalDate.ofEpochDay(300);
System.out.println("300th day from base date: " + LocalDate_FromBase);
LocalDate HundredDay_2022 = LocalDate.ofYearDay(2022, 100);
System.out.println("100th day of 2022: " + HundredDay_2022);
}
}
The code above uses LocalDate
to get the current date, specific date, date from a particular timezone, and the date on a particular day of the year. See output:
The Current Date is: 2022-09-13
The Specific Date with inputs: 2022-01-01
The Current Date in Karachi is: 2022-09-13
300th day from base date: 1970-10-28
100th day of 2022: 2022-04-10
Now let’s break down the provided Java code into a detailed explanation, step by step:
LocalDate Current_LocalDate = LocalDate.now();
System.out.println("The Current Date is: " + Current_LocalDate);
The code utilizes LocalDate.now()
to obtain the current date in the default time zone. The current date is then printed to the console for display.
LocalDate FirstLocalDate_2022 = LocalDate.of(2022, Month.JANUARY, 1);
System.out.println("The Specific Date with inputs: " + FirstLocalDate_2022);
LocalDate.of()
is employed to create a specific date (January 1, 2022, in this case) by providing the year, month (represented by Month.JANUARY
), and day as arguments. The specific date is assigned to a variable and then printed to the console.
LocalDate LocalDate_Karachi = LocalDate.now(ZoneId.of("Asia/Karachi"));
System.out.println("The Current Date in Karachi is: " + LocalDate_Karachi);
In this part, an attempt is made to create a LocalDate
instance using LocalDate.now()
with an invalid input - providing a time zone using ZoneId.of()
. Since LocalDate
does not accept a time zone as a parameter, this results in a compilation error.
LocalDate LocalDate_FromBase = LocalDate.ofEpochDay(300);
System.out.println("300th day from base date: " + LocalDate_FromBase);
The code showcases how to create a LocalDate
instance from the base date (1970-01-01) by providing the number of days since that base date (300 days in this case). The calculated date is then printed to the console.
LocalDate HundredDay_2022 = LocalDate.ofYearDay(2022, 100);
System.out.println("100th day of 2022: " + HundredDay_2022);
Here, LocalDate.ofYearDay()
is utilized to create a LocalDate
for a specific day of a given year (the 100th day of 2022 in this case).
Java Date
vs. LocalDate
Here’s a table summarizing the important differences between Date
and LocalDate
in Java:
Aspect | java.util.Date |
java.time.LocalDate |
---|---|---|
Package | java.util.Date is the core API for a date in Java from JDK 1.0. |
java.time.LocalDate was introduced in the 1.8 version of Java. |
Mutability | Date is mutable, allowing modifications to its value after instantiation. |
LocalDate is immutable; once created, its value cannot be changed. |
Timezone Handling | Represents an instant in time, including both date and time components. It is influenced by the system’s default timezone when displayed or manipulated. | Represents a date without any time or timezone information. Does not store any timezone-related data, making it independent of time zones. |
Precision | Provides millisecond precision, including time information down to milliseconds. | Provides date precision, focusing only on the date component in ISO format (yyyy-MM-dd ). |
Usage | Suitable for scenarios where both date and time are needed, such as scheduling events. | Ideal for applications dealing with date-related operations, where time and timezone information is unnecessary, like birthdays and paydays. |
Legacy/Modern | A legacy class that has been part of Java since early versions. | A modern class introduced in Java 8 as part of the java.time package. |
When deciding between Date
and LocalDate
, consider the requirements of your application. If your application needs to handle both date and time or work with legacy code that uses Date
, then Date
may still be necessary.
However, for modern Java applications that primarily require date-related operations and clarity in handling dates, LocalDate
is often the better choice due to its immutability, timezone independence, and simplicity.
In summary, Date
is suitable for scenarios requiring both date and time, while LocalDate
is ideal for applications focusing solely on date-related operations. As Java evolves, the trend is moving towards using the java.time
package, including LocalDate
, for improved date and time handling.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook