How to Convert String to LocalDate in Java
-
Convert String to
LocalDate
Using theparse()
Method in Java -
Convert String to
LocalDate
Using theparse()
andofPattern()
Method in Java - Convert String Date That Has a Month as String Name in Java
- Convert String Date That Has Day and Month as String Name in Java
-
Convert String Date That Has Time to
LocalDate
in Java - Conclusion
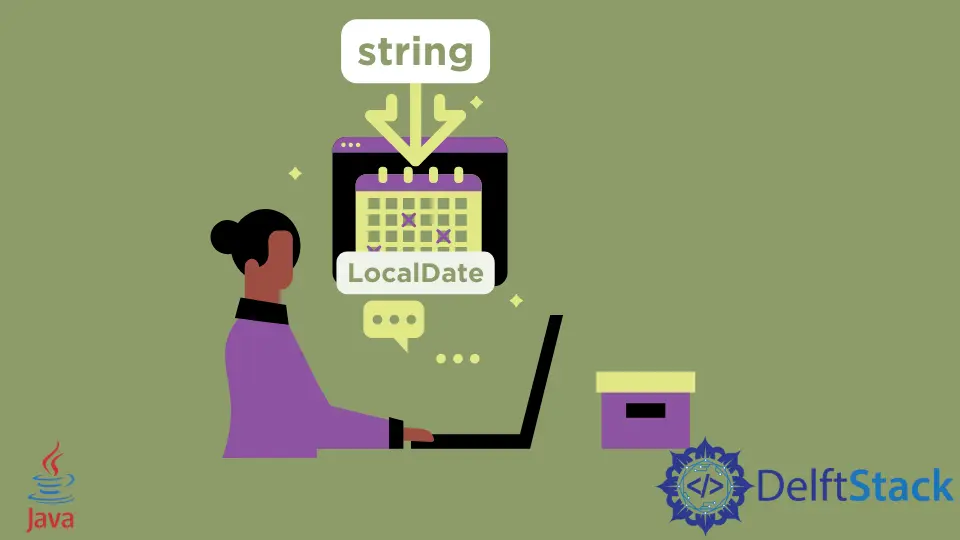
This tutorial introduces the conversion of String to LocalDate
with examples in Java.
LocalDate
is a class in Java, which helps us represent the local date. The format in which the date is represented is yyyy-mm-dd
.
Sometimes, we might want to convert a String into LocalDate
to use the functions provided by the LocalDate
class. In this tutorial, we will see various ways by which we can convert String to LocalDate
.
The date can be represented in many formats; we need to create a formatter instance. We can create a formatter by using the DateTimeFormatter
class.
We then pass the formatter instance into the LocalDate.parse()
method. The LocalDate.parse()
method throws DateTimeParseException
if string passed is not parsable.
Note that parsing equals converting String to date, and formatting means converting the date to String in Java.
Convert String to LocalDate
Using the parse()
Method in Java
If the date is in ISO Local Date format (yyyy-mm-dd
), we don’t need to set the format. We can directly parse the String to the parse()
method that returns the local date.
See the example below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "2021-12-21";
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date);
System.out.println(local_date);
}
}
Output:
2021-12-21
Convert String to LocalDate
Using the parse()
and ofPattern()
Method in Java
If the String date is not in ISO format, we need to convert its format by using the DateTimeFormatter.ofPattern()
method, and then call the parse()
method by passing the format type as the argument.
See the example below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21/12/2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
Output:
2021-12-21
Convert String Date That Has a Month as String Name in Java
If the String date has a month name in letters like Jan
, Feb
, Mar
, etc., then we need to use the ofPattern()
method with the MMM
argument, which works without error only if the default locale is either Locale.English
or Locale.US
.
In other words, the locale should understand the language in which the month is written to parse the String. A locale is an object which represents a specific geographical, political, or cultural region.
Look at the code below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-Dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
Output:
2021-12-21
Note that the above format is case-sensitive. We will get an exception if we write dec
instead of Dec
.
See the example below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
Output:
Exception in thread "main" java.time.format.DateTimeParseException: Text '21-dec-2021' could not be parsed at index 3
at java.base/java.time.format.DateTimeFormatter.parseResolved0(DateTimeFormatter.java:2052)
at java.base/java.time.format.DateTimeFormatter.parse(DateTimeFormatter.java:1954)
at java.base/java.time.LocalDate.parse(LocalDate.java:430)
at SimpleTesting.main(SimpleTesting.java:8)
Let us now change the default locale to another country that doesn’t understand English, like China. We do this by using the Locale.setDefault()
method and passing the required locale as an argument, in this case, Locale.CHINESE
.
Look at the code below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
Locale.setDefault(Locale.CHINESE); // setting locale as chinese
String JE_date = "21-Dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
Output:
Exception in thread "main" java.time.format.DateTimeParseException: Text '21-Dec-2021' could not be parsed at index 3
at java.base/java.time.format.DateTimeFormatter.parseResolved0(DateTimeFormatter.java:2052)
at java.base/java.time.format.DateTimeFormatter.parse(DateTimeFormatter.java:1954)
at java.base/java.time.LocalDate.parse(LocalDate.java:430)
at SimpleTesting.main(SimpleTesting.java:10)
In the above code, we get an error because the Chinese locale doesn’t understand English, and therefore it cannot understand the month’s name. Suppose that our String is in French: 21-mai-2021
, then to convert this into a date, we need to pass Locale.FRANCE
as an argument in the ofPattern()
method.
See the example below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-mai-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy", Locale.FRANCE);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
Output:
2021-05-21
So, to avoid exceptions, pass Locale.US
as an argument when the date contains English words.
Convert String Date That Has Day and Month as String Name in Java
String date may contain day and month name as English abbreviation such as Tue, Dec 21, 2021
. To convert this date to a local date, just like the previous case, we can easily convert this format by using the ofPattern()
method.
See the example below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "Tue, Dec 21 2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("E, MMM d yyyy", Locale.US);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
Output:
2021-12-21
Notice, we have passed Locale.US
as an argument in the ofPattern()
method. It is to prevent an exception if the default locale isn’t English.
Convert String Date That Has Time to LocalDate
in Java
String date may also have time with the date like Tuesday, Dec 21, 2021, 10:30:15 PM
. To convert this date, we need to set the format first in the ofPattern()
method and then use the parse()
method to get the local date.
See the example below.
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "Tuesday, Dec 21, 2021 10:30:15 PM";
DateTimeFormatter JEFormatter =
DateTimeFormatter.ofPattern("EEEE, MMM d, yyyy hh:mm:ss a", Locale.US);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
Output:
2021-12-21
Conclusion
This tutorial discussed converting String to LocalDate
in Java. Apart from the above-discussed formats, several other formats can be used to get the date.
Related Article - Java Date
- How to Find the Day of the Week Using Zellers Congruence in Java
- How to Get Day of Week in Java
- Java Date vs. LocalDate
- How to Set Time Zone of a java.util.Date
- Calendar Date in YYYY-MM-DD Format in Java