在 Java 中將字串轉換為 LocalDate
-
使用 Java 中的
parse()
方法將字串轉換為LocalDate
-
使用 Java 中的
parse()
和ofPattern()
方法將字串轉換為LocalDate
- 在 Java 中轉換有月份的字串日期為字串名稱
- 將具有日和月的字串日期轉換為 Java 中的字串名稱
-
在 Java 中將有時間的字串日期轉換為
LocalDate
- まとめ
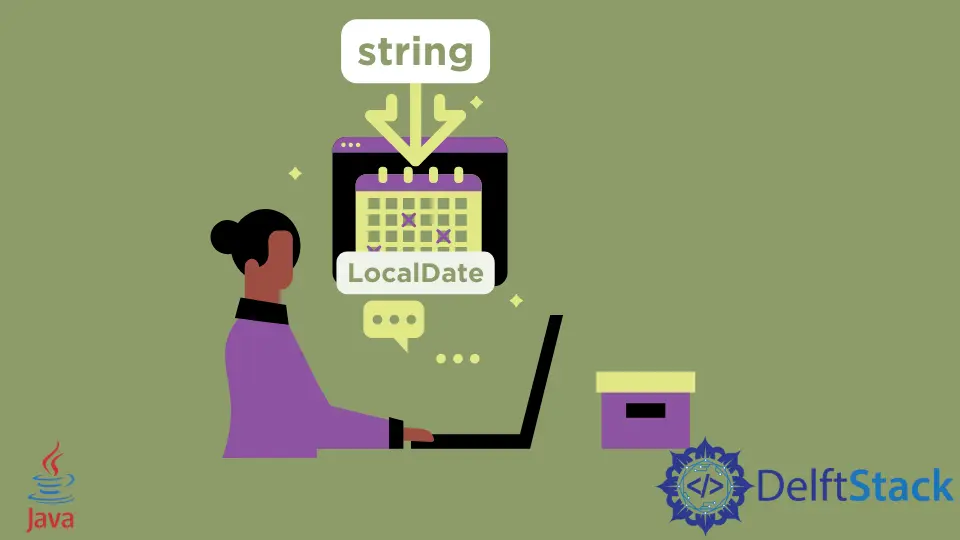
本教程通過 Java 示例介紹了 String 到 LocalDate
的轉換。
LocalDate
是 Java 中的一個類,它幫助我們表示本地日期。表示日期的格式是 yyyy-mm-dd
。
有時,我們可能希望將 String 轉換為 LocalDate
以使用 LocalDate
類提供的功能。在本教程中,我們將看到將 String 轉換為 LocalDate
的各種方法。
日期可以用多種格式表示;我們需要建立一個格式化程式例項。我們可以使用 DateTimeFormatter
類建立格式化程式。
然後我們將格式化程式例項傳遞給 LocalDate.parse()
方法。如果傳遞的字串不可解析,則 LocalDate.parse()
方法將丟擲 DateTimeParseException
。
請注意,解析等於將 String 轉換為日期,而格式化則意味著將日期轉換為 Java 中的 String。
使用 Java 中的 parse()
方法將字串轉換為 LocalDate
如果日期是 ISO 本地日期格式(yyyy-mm-dd
),我們不需要設定格式。我們可以直接將 String 解析為返回本地日期的 parse()
方法。
請參見下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "2021-12-21";
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date);
System.out.println(local_date);
}
}
輸出:
2021-12-21
使用 Java 中的 parse()
和 ofPattern()
方法將字串轉換為 LocalDate
如果 String 日期不是 ISO 格式,我們需要使用 DateTimeFormatter.ofPattern()
方法轉換其格式,然後通過傳遞格式型別作為引數呼叫 parse()
方法。
請參見下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21/12/2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
輸出:
2021-12-21
在 Java 中轉換有月份的字串日期為字串名稱
如果字串日期有一個月份名稱,如 Jan
、Feb
、Mar
等,那麼我們需要使用帶有 MMM
引數的 ofPattern()
方法,該方法僅在沒有錯誤的情況下工作如果預設語言環境是 Locale.English
或 Locale.US
。
換句話說,語言環境應該理解編寫月份以解析字串的語言。區域設定是表示特定地理、政治或文化區域的物件。
看看下面的程式碼。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-Dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
輸出:
2021-12-21
請注意,上述格式區分大小寫。如果我們寫 dec
而不是 Dec
,我們會得到一個異常。
請參見下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
輸出:
Exception in thread "main" java.time.format.DateTimeParseException: Text '21-dec-2021' could not be parsed at index 3
at java.base/java.time.format.DateTimeFormatter.parseResolved0(DateTimeFormatter.java:2052)
at java.base/java.time.format.DateTimeFormatter.parse(DateTimeFormatter.java:1954)
at java.base/java.time.LocalDate.parse(LocalDate.java:430)
at SimpleTesting.main(SimpleTesting.java:8)
現在讓我們將預設語言環境更改為另一個不懂英語的國家,例如中國。我們通過使用 Locale.setDefault()
方法並將所需的語言環境作為引數傳遞,在本例中為 Locale.CHINESE
。
看看下面的程式碼。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
Locale.setDefault(Locale.CHINESE); // setting locale as chinese
String JE_date = "21-Dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
輸出:
Exception in thread "main" java.time.format.DateTimeParseException: Text '21-Dec-2021' could not be parsed at index 3
at java.base/java.time.format.DateTimeFormatter.parseResolved0(DateTimeFormatter.java:2052)
at java.base/java.time.format.DateTimeFormatter.parse(DateTimeFormatter.java:1954)
at java.base/java.time.LocalDate.parse(LocalDate.java:430)
at SimpleTesting.main(SimpleTesting.java:10)
在上面的程式碼中,我們得到一個錯誤,因為中文語言環境不理解英文,因此它無法理解月份的名稱。假設我們的字串是法語:21-mai-2021
,然後要將其轉換為日期,我們需要將 Locale.FRANCE
作為引數傳遞給 ofPattern()
方法。
請參見下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-mai-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy", Locale.FRANCE);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
輸出:
2021-05-21
因此,為避免異常,當日期包含英文單詞時,將 Locale.US
作為引數傳遞。
將具有日和月的字串日期轉換為 Java 中的字串名稱
字串日期可以包含日期和月份名稱作為英文縮寫,例如 Tue, Dec 21, 2021
。要將此日期轉換為本地日期,就像前面的情況一樣,我們可以使用 ofPattern()
方法輕鬆轉換此格式。
請參見下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "Tue, Dec 21 2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("E, MMM d yyyy", Locale.US);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
輸出:
2021-12-21
請注意,我們已將 Locale.US
作為引數傳遞給 ofPattern()
方法。如果預設語言環境不是英語,這是為了防止異常。
在 Java 中將有時間的字串日期轉換為 LocalDate
字串日期也可能與日期有時間,例如 Tuesday, Dec 21, 2021, 10:30:15 PM
。要轉換這個日期,我們需要先在 ofPattern()
方法中設定格式,然後使用 parse()
方法獲取本地日期。
請參見下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "Tuesday, Dec 21, 2021 10:30:15 PM";
DateTimeFormatter JEFormatter =
DateTimeFormatter.ofPattern("EEEE, MMM d, yyyy hh:mm:ss a", Locale.US);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
輸出:
2021-12-21
まとめ
本教程討論了在 Java 中將 String 轉換為 LocalDate
。除了上面討論的格式之外,還可以使用其他幾種格式來獲取日期。