在 Java 中将字符串转换为 LocalDate
-
使用 Java 中的
parse()
方法将字符串转换为LocalDate
-
使用 Java 中的
parse()
和ofPattern()
方法将字符串转换为LocalDate
- 在 Java 中转换有月份的字符串日期为字符串名称
- 将具有日和月的字符串日期转换为 Java 中的字符串名称
-
在 Java 中将有时间的字符串日期转换为
LocalDate
- 结论
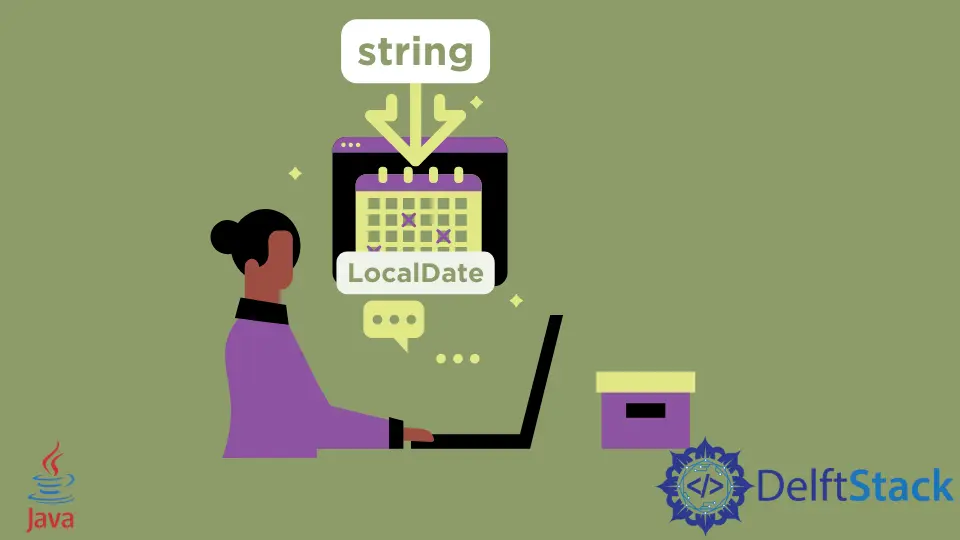
本教程通过 Java 示例介绍了 String 到 LocalDate
的转换。
LocalDate
是 Java 中的一个类,它帮助我们表示本地日期。表示日期的格式是 yyyy-mm-dd
。
有时,我们可能希望将 String 转换为 LocalDate
以使用 LocalDate
类提供的功能。在本教程中,我们将看到将 String 转换为 LocalDate
的各种方法。
日期可以用多种格式表示;我们需要创建一个格式化程序实例。我们可以使用 DateTimeFormatter
类创建格式化程序。
然后我们将格式化程序实例传递给 LocalDate.parse()
方法。如果传递的字符串不可解析,则 LocalDate.parse()
方法将抛出 DateTimeParseException
。
请注意,解析等于将 String 转换为日期,而格式化则意味着将日期转换为 Java 中的 String。
使用 Java 中的 parse()
方法将字符串转换为 LocalDate
如果日期是 ISO 本地日期格式(yyyy-mm-dd
),我们不需要设置格式。我们可以直接将 String 解析为返回本地日期的 parse()
方法。
请参见下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "2021-12-21";
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date);
System.out.println(local_date);
}
}
输出:
2021-12-21
使用 Java 中的 parse()
和 ofPattern()
方法将字符串转换为 LocalDate
如果 String 日期不是 ISO 格式,我们需要使用 DateTimeFormatter.ofPattern()
方法转换其格式,然后通过传递格式类型作为参数调用 parse()
方法。
请参见下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21/12/2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
输出:
2021-12-21
在 Java 中转换有月份的字符串日期为字符串名称
如果字符串日期有一个月份名称,如 Jan
、Feb
、Mar
等,那么我们需要使用带有 MMM
参数的 ofPattern()
方法,该方法仅在没有错误的情况下工作如果默认语言环境是 Locale.English
或 Locale.US
。
换句话说,语言环境应该理解编写月份以解析字符串的语言。区域设置是表示特定地理、政治或文化区域的对象。
看看下面的代码。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-Dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
输出:
2021-12-21
请注意,上述格式区分大小写。如果我们写 dec
而不是 Dec
,我们会得到一个异常。
请参见下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
输出:
Exception in thread "main" java.time.format.DateTimeParseException: Text '21-dec-2021' could not be parsed at index 3
at java.base/java.time.format.DateTimeFormatter.parseResolved0(DateTimeFormatter.java:2052)
at java.base/java.time.format.DateTimeFormatter.parse(DateTimeFormatter.java:1954)
at java.base/java.time.LocalDate.parse(LocalDate.java:430)
at SimpleTesting.main(SimpleTesting.java:8)
现在让我们将默认语言环境更改为另一个不懂英语的国家,例如中国。我们通过使用 Locale.setDefault()
方法并将所需的语言环境作为参数传递,在本例中为 Locale.CHINESE
。
看看下面的代码。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
Locale.setDefault(Locale.CHINESE); // setting locale as chinese
String JE_date = "21-Dec-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy");
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
输出:
Exception in thread "main" java.time.format.DateTimeParseException: Text '21-Dec-2021' could not be parsed at index 3
at java.base/java.time.format.DateTimeFormatter.parseResolved0(DateTimeFormatter.java:2052)
at java.base/java.time.format.DateTimeFormatter.parse(DateTimeFormatter.java:1954)
at java.base/java.time.LocalDate.parse(LocalDate.java:430)
at SimpleTesting.main(SimpleTesting.java:10)
在上面的代码中,我们得到一个错误,因为中文语言环境不理解英文,因此它无法理解月份的名称。假设我们的字符串是法语:21-mai-2021
,然后要将其转换为日期,我们需要将 Locale.FRANCE
作为参数传递给 ofPattern()
方法。
请参见下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "21-mai-2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("dd-MMM-yyyy", Locale.FRANCE);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
输出:
2021-05-21
因此,为避免异常,当日期包含英文单词时,将 Locale.US
作为参数传递。
将具有日和月的字符串日期转换为 Java 中的字符串名称
字符串日期可以包含日期和月份名称作为英文缩写,例如 Tue, Dec 21, 2021
。要将此日期转换为本地日期,就像前面的情况一样,我们可以使用 ofPattern()
方法轻松转换此格式。
请参见下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "Tue, Dec 21 2021";
DateTimeFormatter JEFormatter = DateTimeFormatter.ofPattern("E, MMM d yyyy", Locale.US);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
输出:
2021-12-21
请注意,我们已将 Locale.US
作为参数传递给 ofPattern()
方法。如果默认语言环境不是英语,这是为了防止异常。
在 Java 中将有时间的字符串日期转换为 LocalDate
字符串日期也可能与日期有时间,例如 Tuesday, Dec 21, 2021, 10:30:15 PM
。要转换这个日期,我们需要先在 ofPattern()
方法中设置格式,然后使用 parse()
方法获取本地日期。
请参见下面的示例。
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Locale;
public class SimpleTesting {
public static void main(String args[]) {
String JE_date = "Tuesday, Dec 21, 2021 10:30:15 PM";
DateTimeFormatter JEFormatter =
DateTimeFormatter.ofPattern("EEEE, MMM d, yyyy hh:mm:ss a", Locale.US);
// parsing the string to convert it into date
LocalDate local_date = LocalDate.parse(JE_date, JEFormatter);
System.out.println(local_date);
}
}
输出:
2021-12-21
结论
本教程讨论了在 Java 中将 String 转换为 LocalDate
。除了上面讨论的格式之外,还可以使用其他几种格式来获取日期。