How to Convert a String to an Int in Java
-
Convert a
String
to anint
UsingparseInt()
in Java -
Convert
String
to anint
UsingApache
in Java -
Convert
String
to anint
Usingdecode()
in Java -
Convert
String
to anint
UsingvalueOf()
in Java -
Convert
String
to anint
UsingparseUnsignedInt()
in Java -
Convert
String
After Removing Non-Numeric Chars UsingreplaceAll()
in Java
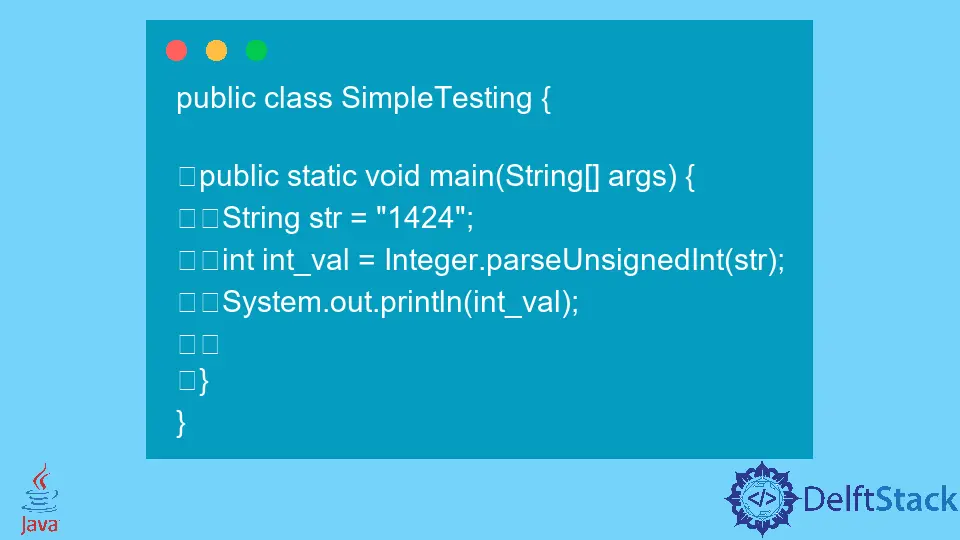
This tutorial introduces how to convert a String
to an int
in Java and lists some example codes to understand it.
Convert a String
to an int
Using parseInt()
in Java
Both String
and int
are data types in Java. The String
is used to store textual information while int
is used to store numerical data.
Here, we use the parseInt()
method of the Integer
class that returns an integer. Since Java does implicit auto-boxing, then we can store it into int primitive type. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1234";
int int_val = Integer.parseInt(str);
System.out.println(int_val);
}
}
Output:
1234
Convert String
to an int
Using Apache
in Java
If you are working with the Apache
library, you can use the toInt()
method of NumberUtils
class that returns an int value. If the passed string contains any non-numeric char, this method does not throw any exception, but returns 0
. It is safe to use toInt()
rather than parseInt()
which throws NumberFormatException
. See the example below.
import org.apache.commons.lang3.math.NumberUtils;
public class SimpleTesting {
public static void main(String[] args) {
String str = "1234";
int int_val = NumberUtils.toInt(str);
System.out.println(int_val);
str = "1234x"; // non-numeric string
int_val = NumberUtils.toInt(str);
System.out.println(int_val);
}
}
Output:
1234
0
Convert String
to an int
Using decode()
in Java
We can use the decode()
method of the Integer
class to get int value from a string. It returns Integer
value, but if you want to get int
(primitive) value then use intValue()
with decode()
method. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1424";
int int_val = Integer.decode(str);
System.out.println(int_val);
// int primitive
int_val = Integer.decode(str).intValue();
System.out.println(int_val);
}
}
Output:
1424
1424
Convert String
to an int
Using valueOf()
in Java
We can use the valueOf()
method to get an int value of a string after conversion.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1424";
int int_val = Integer.valueOf(str);
System.out.println(int_val);
}
}
1424
1424
Convert String
to an int
Using parseUnsignedInt()
in Java
If we want to convert a string that does not include a sign, use the parseUnsignedInt()
method to get int value. It works fine with unsigned string value and throws an exception if the value is signed.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1424";
int int_val = Integer.parseUnsignedInt(str);
System.out.println(int_val);
}
}
Output:
1424
Convert String
After Removing Non-Numeric Chars Using replaceAll()
in Java
If the string contains non-numeric chars, use the replaceAll()
method of String
class with regex
to remove all the non-numeric chars and then use the parseInt()
method of Integer
class to get its equivalent integer value. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "1424x";
str = str.replaceAll("[^\\d]", "");
int int_val = Integer.parseInt(str);
System.out.println(int_val);
}
}
Output:
1424
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java