How to Convert String to Int Array in Java
-
Convert String to Int Array Using the
replaceAll()
Method - Convert String to Int Array Using Java 8 Stream Library
- Convert String to Int Array Using StringTokenizer and Functions
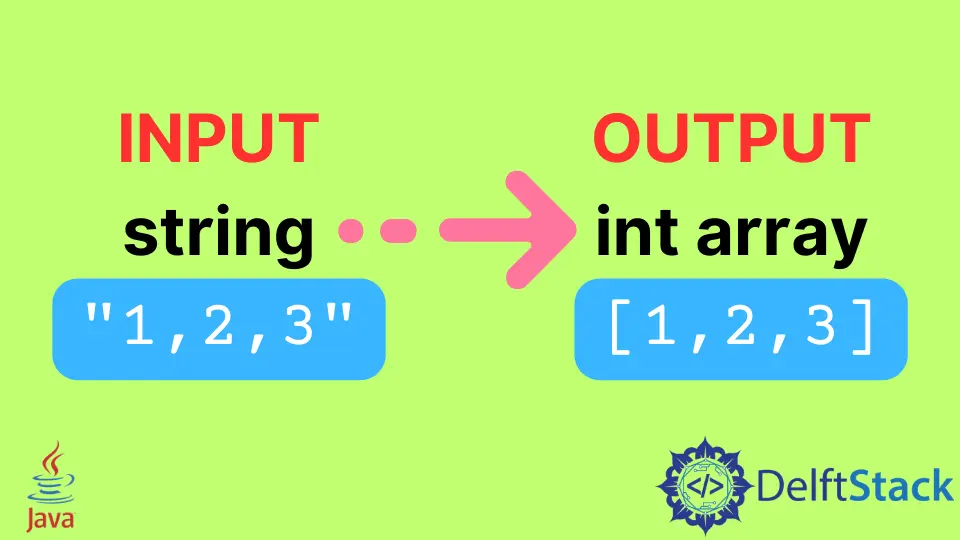
In the Java language, there can be several approaches to think over the problem statement.
Let us first break the problem statement into two parts.
- Convert a simple String to the String array.
- Convert a String array to an int array.
Here is the diagrammatic representation of the above two steps:
Convert String to Int Array Using the replaceAll()
Method
We can use the replaceAll()
method of the String
class that takes two arguments, regex
and replacement
values. This method will replace the given regex with the given replacement value. And at the end of the chain functions, the split()
method is used. This method splits the String based on the character given.
We should use the Integer.parseInt()
function when the values are split and collected in a String array. Since the parseInt()
method throws NumberFormatException
, so the statement should be in one try...catch
block to catch the possibly exception.
Below is the sample program to demonstrate the conversion of strings to int array:
package stringToIntArray;
import java.util.Arrays;
public class ConvertStringToIntArray {
public static void main(String... args) {
String testString = "[1,2,356,678,3378]";
String[] separatedStrings = testString.replaceAll("\\[", "").replaceAll("]", "").split(",");
int[] intArray = new int[separatedStrings.length];
for (int i = 0; i < separatedStrings.length; i++) {
try {
intArray[i] = Integer.parseInt(separatedStrings[i]);
} catch (Exception e) {
System.out.println("Unable to parse string to int: " + e.getMessage());
}
}
System.out.println(Arrays.toString(intArray));
}
}
Output:
[1,2,356,678,3378]
If testString
has value as [1,2,356,678,3378,f]
, it will throw an exception, and the output will look like this.
Unable to parse string to int: For input string: "f"
[1, 2, 356, 678, 3378, 0]
Convert String to Int Array Using Java 8 Stream Library
In the below program, first, we have taken the Arrays.stream()
function. To provide a stream to this, we have used the substring()
method of the String
class, which takes the first and last index parameters. Over the returned String split()
function is applied, which splits the given String based on a delimiter.
Over the stream map()
function is applied. This function takes any function as an argument and returns the stream. We have given the trim()
function of String class which eliminated the leading and succeeding whitespaces. parseInt
transforms the given String received to int. toArray()
dumps converted int elements to an Array.
package stringToIntArray;
import java.util.Arrays;
public class StringToIntUsingJava
8Stream {
public static void main(String[] args) {
String str = "[1, 2, 3, 4, 5]";
int[] arr = Arrays.stream(str.substring(1, str.length() - 1).split(","))
.map(String::trim)
.mapToInt(Integer::parseInt)
.toArray();
System.out.println(Arrays.toString(arr));
}
}
Output:
[1, 2, 3, 4, 5]
Convert String to Int Array Using StringTokenizer and Functions
Instantiate StringTokenizer object using its constructor StringTokenizer()
. The constructor takes the input String and the delimiter to form a tokenized String. Simultaneously create an array of String and int with size as tokens in the tokenized String.
The countTokens()
method counts the tokens, which internally skips the delimiter. hasMoreTokens()
checks if there are any tokens present in tokenizer String. nextToken()
retrieves the next token from the String. This method throws NoSuchElementException
if no-tokens are present in the String.
Iterate to convert the String tokens to int and collect in an int array.
package stringToIntArray;
import java.util.StringTokenizer;
public class StringToIntUsingStringTokenizer {
public static void main(String[] args) {
String testString = "[1,2,3,4]";
StringTokenizer stk = new StringTokenizer(testString, "[,]");
String[] strings = new String[stk.countTokens()];
int[] integerArray = new int[stk.countTokens()];
int i = 0;
while (stk.hasMoreTokens()) {
strings[i] = stk.nextToken();
integerArray[i] = Integer.parseInt(strings[i]);
i++;
}
for (int j = 0; j < integerArray.length; j++)
System.out.println("number[" + j + "]=" + integerArray[j]);
}
}
Output:
number[0]=1
number[1]=2
number[2]=3
number[3]=4
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java