How to Convert an Array to String in Java
-
Convert an Array to a String Using the
Arrays.toString()
Method in Java -
Convert an Array to a String Using the
String.join()
Method in Java -
Convert an Array to a String Using the
Arrays.stream()
Method in Java
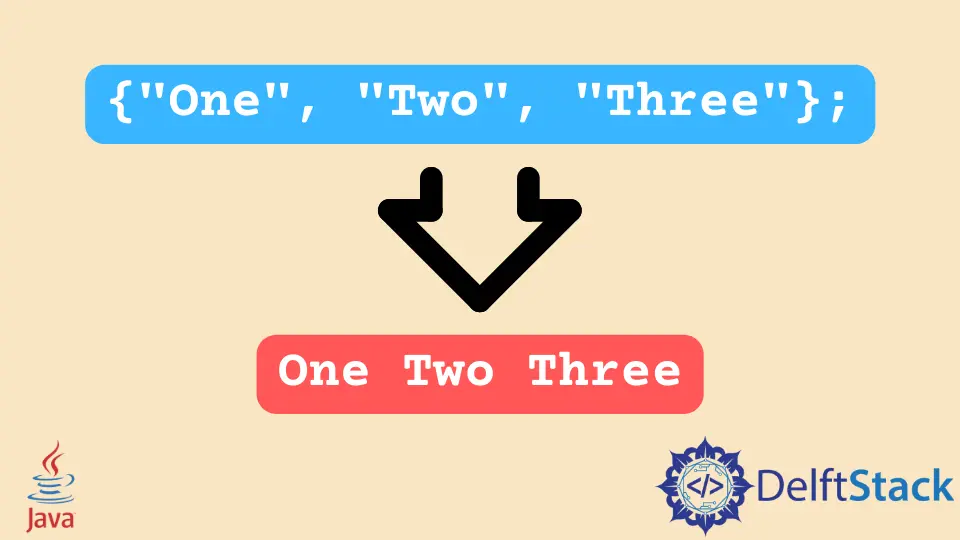
In this tutorial, we will see how to convert an array to a string using various ways in Java. An array is made up of elements of the same data type, while a string is just a collection of characters. In the following examples, we will go through three methods to convert an array to a string.
Convert an Array to a String Using the Arrays.toString()
Method in Java
Arrays
is a class that contains various static methods that can manipulate arrays. One of the useful functions of Arrays
is toString()
, which takes in an array of various data types like int
and char
and returns a string representation of the array.
In the example, we create an array arrayOfInts
of int
type and fill it with a few items. To convert arrayOfInts
to a string, we use Arrays.toString()
and pass it as an argument that returns a string arrayToString
that we print in the output.
import java.util.Arrays;
public class ArrayToString {
public static void main(String[] args) {
int[] arrayOfInts = {1, 3, 9, 11, 13};
String arrayToString = Arrays.toString(arrayOfInts);
System.out.println(arrayToString);
}
}
Output:
[1, 3, 9, 11, 13]
Convert an Array to a String Using the String.join()
Method in Java
The join()
method was added in the String
class with the release of JDK 8. This function returns a string that is concatenated with the specified delimiter. join()
takes in the delimiter and the elements as arguments.
In the code, we have an array of the String
type. We call the String.join()
method and pass the whitespace as the delimiter and also the array whose elements will be joined with the whitespace.
The output shows all the items of the array separated by whitespaces.
public class ArrayToString {
public static void main(String[] args) {
String[] arrayOfStrings = {"One", "Two", "Three", "four", "Five"};
String arrayToString = String.join(" ", arrayOfStrings);
System.out.println(arrayToString);
}
}
Output:
One Two Three four Five
Convert an Array to a String Using the Arrays.stream()
Method in Java
In this example, we use the Stream API introduced in JDK 8. Arrays.stream()
takes in an array. The collect()
method returns the result after executing the specified operation on every element of the array. Here, we perform the Collectors.joining()
operation on the array elements that collects items and joins them to return as a whole string.
import java.util.Arrays;
import java.util.stream.Collectors;
public class ArrayToString {
public static void main(String[] args) {
String[] arrayOfStrings = {"One", "Two", "Three", "four", "Five"};
String arrayToString = Arrays.stream(arrayOfStrings).collect(Collectors.joining());
System.out.println(arrayToString);
}
}
Output:
OneTwoThreefourFive
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java