How to Split String to List in C#
-
Split String to List Using the
String.Split()
Method in C# -
Split String to List Using the
Regex.Split()
Method in C# -
Split String to List Using
String.Split()
WithStringSplitOptions
in C# -
Split String to List Using
String.Split()
With Multiple Delimiters in C# -
Split String to List Using
String.Split()
With Character Array in C# -
Split String to List Using
String.Split()
WithStringSplitOptions
andTrim()
- Conclusion
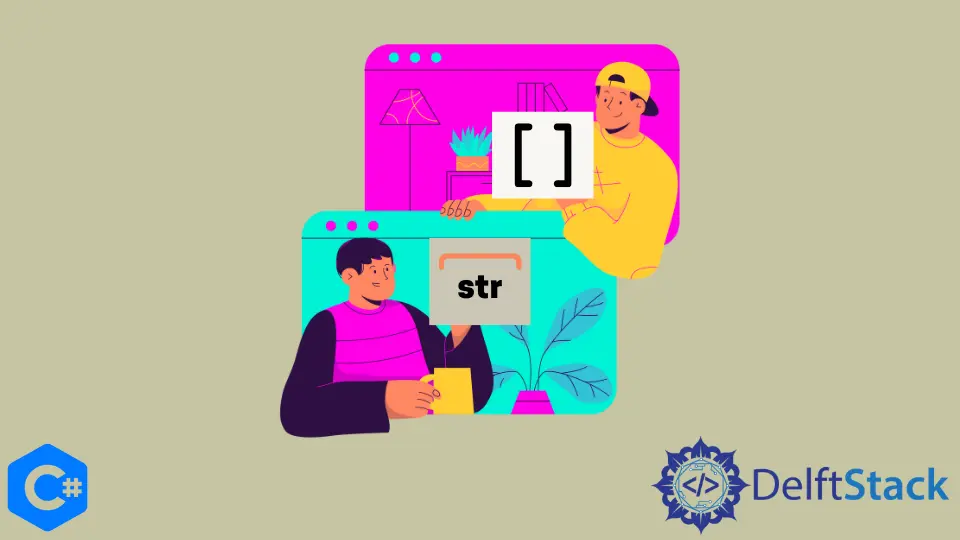
String manipulation is a crucial part of C# programming. Frequently, we’ll encounter situations where we need to divide a string into smaller components, and there are multiple ways to accomplish this.
In this tutorial, we’ll delve into various techniques for breaking down a string into a collection of smaller strings in C#. We’ll not only explain how each method functions but also provide insights into when and why we might use them.
Split String to List Using the String.Split()
Method in C#
The String.Split()
method in C# is a widely used method for splitting a string into an array of substrings based on a specified delimiter. It’s part of the System.String
class and provides a straightforward way to break down a string into its constituent parts.
Here’s the syntax of the String.Split()
method:
string[] Split(params char[] separator);
string[] Split(char[] separator, StringSplitOptions options);
string[] Split(char[] separator, int count, StringSplitOptions options);
Parameters:
separator
: An array of characters that serves as the delimiter for splitting the string. The method splits the string wherever it encounters any of the specified characters.count
: An optional parameter that limits the number of substrings produced by the split operation. If specified, the method stops splitting aftercount - 1
substrings have been created.options
: An enumeration of typeStringSplitOptions
that allows us to control how empty entries are treated during the split operation. It can have one of two values:StringSplitOptions.None
: This is the default behavior and includes empty entries in the resulting array.StringSplitOptions.RemoveEmptyEntries
: This option excludes empty entries from the resulting array.
The String.Split()
method returns an array of strings containing the substrings obtained by splitting the original string. After obtaining the array of strings, we can convert it into a list by using the ToList()
function of Linq in C#.
See the example code below:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
string input = "this,needs,to,split";
List<string> result = input.Split(',').ToList();
// Printing the elements in the list
foreach (string item in result) {
Console.WriteLine(item);
}
}
}
Output:
this
needs
to
split
As we can see, the ToList()
method successfully converted an array of strings, which is the result of the Split()
method, into a List<string>
.
Split String to List Using the Regex.Split()
Method in C#
We can also use the Regex.Split()
method in C# to split a string into an array of substrings based on a specified regular expression pattern.
This method in C# has the following syntax:
public static string[] Split(string input, string pattern);
Parameters:
input
: This is the input string that we want to split into substrings.pattern
: This is a string that represents the regular expression pattern used to determine where theinput
string should be split. This means theinput
string will be split at locations where this pattern is matched.
The method returns an array of strings, with each element in the array representing one of the resulting substrings from the split operation. The substrings do not include the matching delimiter.
Similar to the first method, the array of strings returned by the Regex.Split()
method is converted into a list using the ToList()
function.
Here’s an example:
using System;
using System.Collections.Generic;
using System.Text.RegularExpressions;
using System.Linq;
class Program {
static void Main() {
string input = "this,needs,to,split";
List<string> result = Regex.Split(input, ",").ToList();
foreach (string item in result) {
Console.WriteLine(item);
}
}
}
Output:
this
needs
to
split
In this example, we used a regular expression to split the input
string at each comma (,
). The result is the same as in the first method.
Split String to List Using String.Split()
With StringSplitOptions
in C#
The String.Split()
method can also be customized by using the StringSplitOptions
enumeration. This approach splits a string based on a delimiter and removes any empty substrings from the result using the StringSplitOptions.RemoveEmptyEntries
option.
It is particularly useful when we have an input string with consecutive delimiters, or we want to exclude empty elements from the list.
Here’s an example:
using System;
class Program {
static void Main() {
string input = "this,,needs,to,split";
string[] result = input.Split(new[] { ',' }, StringSplitOptions.RemoveEmptyEntries);
foreach (string item in result) {
Console.WriteLine(item);
}
}
}
Output:
this
needs
to
split
In this case, the StringSplitOptions.RemoveEmptyEntries
option removes the empty entries, resulting in the result
array containing only this
, needs
, to
, and split
.
Make sure you have a .NET
environment properly configured, and this code will perform as intended.
Split String to List Using String.Split()
With Multiple Delimiters in C#
We can also split a string with multiple delimiters by providing an array of delimiters to the String.Split()
method. This can be useful when our input string uses different separators.
Here’s how it works:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
string input = "this;needs,to,split";
List<string> resultList = new List<string>(input.Split(new[] { ',', ';' }));
foreach (string item in resultList) {
Console.WriteLine(item);
}
}
}
Output:
this
needs
to
split
In this example, the input string is split at both commas (,
) and semicolons (;
), resulting in an array with five elements.
Split String to List Using String.Split()
With Character Array in C#
Another way to split a string is by using an array of characters as delimiters. This method allows us to specify multiple characters as splitting points.
Below is an example code:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
string input = "this,needs,to,split";
char[] delimiters = { ',' };
List<string> resultList = new List<string>(input.Split(delimiters));
foreach (string item in resultList) {
Console.WriteLine(item);
}
}
}
Output:
this
needs
to
split
In this case, we specified a character array with a single comma (,
) as the delimiter. The result is the same as in the previous methods above.
Split String to List Using String.Split()
With StringSplitOptions
and Trim()
Sometimes, we may want to remove leading and trailing whitespace from the substrings after splitting. We can achieve this by using the StringSplitOptions.RemoveEmptyEntries
option in combination with the Trim()
method.
This method splits a string based on a delimiter, removes empty substrings, and trims leading and trailing spaces from each element. It’s useful when we want to clean up the individual elements in the resulting list, especially when dealing with input that may have extra spaces or padding.
Here’s an example:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
string input = " this , needs , to , split ";
List<string> resultList = input.Split(new[] { ',' }, StringSplitOptions.RemoveEmptyEntries)
.Select(s => s.Trim())
.ToList();
foreach (string item in resultList) {
Console.WriteLine(item);
}
}
}
Output:
this
needs
to
split
In this example, we split the input string at commas (,
), remove empty entries, and then use Trim()
to remove spaces from the resulting substrings.
Conclusion
In C#, there are multiple methods available for splitting a string into a list of strings, each with its own advantages and use cases. The choice of method depends on the specific requirements of our task, such as the delimiter used, handling of empty entries, and the need for regular expressions. By understanding these methods, we can efficiently manipulate and process strings in our C# applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#