How to Convert List Into Dictionary in C#
-
Convert List Into Dictionary in
C#
-
Convert List to Dictionary Using the LINQ
ToDictionary()
Method inC#
-
Convert List to Dictionary Using the Non-Linq Method in
C#
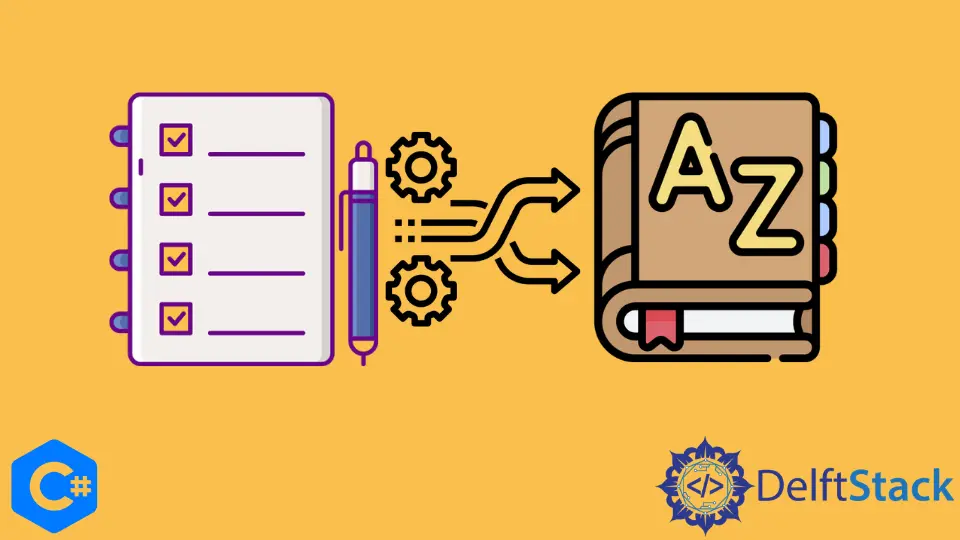
In this article, we will learn how we can convert a list into a dictionary using ToDictionary()
, and we will also get an idea of how we can deal with duplicate keys.
Convert List Into Dictionary in C#
There are many situations where we may have to convert a list into a dictionary. There are a few methods with the help of which we can easily convert a list into a dictionary.
We will discuss these methods in detail with examples below.
Convert List to Dictionary Using the LINQ ToDictionary()
Method in C#
The most effortless way to change a list into a dictionary is through the LINQ ToDictionary()
method, which is shown below.
Example code:
#Csharp
using System.Linq;
var seasonList = GetSeasonsList();
var seasonsById = seasonList.ToDictionary(keySelector: s => S.Id, elementSelector: s => s);
This works by looping within the list and will utilize the key/element selector lambdas, which we have fixed to form the dictionary.
Here, the keySelector
and elementSelector
parameters are mentioned this way to read them easily.
Convert List to Dictionary Using the Non-Linq Method in C#
We can also convert a list to a dictionary in a non-LINQ way using a loop.
Example code:
#Csharp
var seasons = GetSeasonList();
var seasonById = new Dictionary<int, Season>();
foreach (var season in seasons) {
seasonById.Add(season.Id, season);
}
It is advised to use the non-LINQ method because it has improved performance, but we can use either method according to our preferences.
Two types of element selectors can be used in these methods. One is an implicit element selector, and the other is an explicit element selector.
When we use these two statements, the outcome will be the same. Let’s use an implicit element selector, as shown below.
Example code:
#Csharp
var seasonsById = seasonList.ToDictionary(keySelector: s => s.Id, elementSelector: s => s);
Let’s use an explicit element selector, as shown below.
Example code:
#Csharp
var seasonById = seasonList.ToDictionary(keySelector: s => s.Id);
The element selector will robotically use the items in the list as the elements if you have not defined them. This may be what you wish for while marking objects by one of their properties.
Duplicate Keys in C#
The list may have a duplicate key. In this scenario, ToDictionary()
will show an exception that is System.ArgumentException
.
It shows that an item possessing the same key is already on the list when we try to add a duplicate key with the help of the code shown below.
Syntax:
#Csharp
Dictionary.Add(key, value)
We will get the same exception using the code shown above. This is the same exception we get when we try to insert a duplicate key using Dictionary.Add(key, value)
.
By exception, we can avoid duplicate keys in our data.
If we want duplicate keys in our data, we need to group them by the key and select what we want for the value. Let’s see some options as explained below.
an Aggregate Value for the Key in C#
First of all, we will estimate item counts. We can repeat words.
To achieve this, we need to group by the word, and we must set the count as an aggregate.
Let’s go through an example in which we will use this to check for duplicate keys, as shown below.
Example code:
#Csharp
var seasons = new List<string> { "You", "Breaking Bad", "Marvel", "You" };
var seasonCountMap =
seasons.GroupBy(s => s).ToDictionary(keySelector: m => m.Key, elementSelector: m => m.Count());
foreach (var seasonCount in seasonCountMap) {
Console.WriteLine($"{seasonCount.Key}={seasonCount.Value}");
}
Output:
List of Elements for Key in C#
Here, we will catalog some seasons according to their release year. There can be a lot of seasons released each year.
To achieve a dictionary of lists, we can use the following code as shown below.
Example code:
#Csharp
var seasonList = GetSeasonList();
var seasonByYear = seasonList.GroupBy(s => s.YearOfRelease)
.ToDictionary(keySelector: m => m.Key, elementSelector: m => m.ToList());
foreach (var seasonGroup in seasonByYear) {
Console.WriteLine($"{seasonGroup.Key}={string.Join(", ", seasonGroup.Value.Select(v => v.Name))}");
}
First Element With the Key in C#
We have to choose one of the items to describe the group in case of duplicate keys. We can choose the first element in the group as a representative.
Let’s see an example. As shown below, we have listed seasons by year and selected only one season per year.
Example code:
#Csharp
var seasonList = GetSeasonList();
var seasonsByYear = seasonList.GroupBy(s => s.YearOfRelease)
.ToDictionary(keySelector: m => m.Key, elementSelector: m => m.First());
Foreach(var season in seasonsByYear) {
Console.WriteLine($"{season.Key}={season.Value.Name}");
}
Check Duplicates by Looping in C#
You must check if the key is present when using the loop method in place of the LINQ method. By this, you will easily deal with duplicates.
And you have complete freedom to choose how you want to deal with duplicates.
Example code:
#Csharp
using System.IO;
using System;
class Program {
static void Main() {
var seasons = GetSeasonList();
var seasonsByYear = new Dictionary<int, List<Season>>();
foreach (var season in seasons) {
if (!seasonByYear.ContainsKey(season.YearOfRelease)) {
seasonByYear.Add(season.YearOfRelease, new List<Season>());
}
seasonByYear[season.YearOfRelease].Add(season);
}
foreach (var seasonGroup in seasonByYear) {
Console.WriteLine(
$"{seasonGroup.Key}={string.Join(", ", seasonGroup.Value.Select(m =>m.Name))}");
}
}
}
In this example, a list of seasons released every year has been created. When the function notices that the key is not specified yet, it presents it with an empty list and then adds season to it.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Csharp Dictionary
- Best Way to Iterate Over a Dictionary in C#
- How to Sort a Dictionary by Its Keys in C#
- How to Check for the Existence of a Dictionary Key in C#
- Dictionary vs Hashtable in C#
- How to Convert a Dictionary to JSON String in C#
- How to Convert Dictionary to List Collection in C#