How to Convert Dictionary to List Collection in C#
- Understanding Dictionaries and Lists in C#
-
Use the
ToList()
Method to Convert a Dictionary to a List in C# -
Use
KeyValuePair<TKey, TValue>
to Convert Dictionary to List in C# - Conclusion
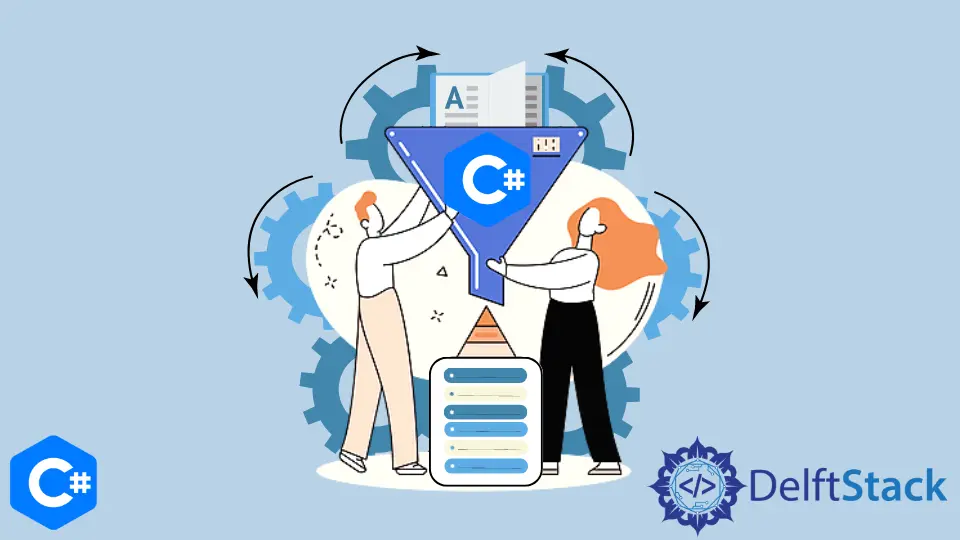
Converting a dictionary to a list enables developers to transform data structures for easier manipulation and processing. Dictionaries store data as key-value pairs, while lists are ordered collections.
Converting from a dictionary to a list allows for straightforward access to individual elements and provides flexibility in data handling. This article will teach you how to convert a dictionary to a list collection in C#.
Understanding Dictionaries and Lists in C#
Before we dive into the conversion process, it’s essential to understand the fundamental differences between dictionaries and lists in C#.
Dictionaries
Dictionaries in C# are a collection of key-value pairs. Each element in a dictionary consists of a unique key and a corresponding value.
This allows for quick retrieval of values based on their associated keys. Dictionaries are often used in scenarios where rapid lookup of data is required.
Example:
Dictionary<string, int> myDictionary = new Dictionary<string, int>();
myDictionary.Add("One", 1);
myDictionary.Add("Two", 2);
myDictionary.Add("Three", 3);
This code creates a Dictionary<string, int>
named myDictionary
and populates it with three key-value pairs. This dictionary associates strings (keys) with integers (values), allowing for efficient retrieval of values based on their corresponding keys.
Lists
On the other hand, lists are ordered collections of elements, allowing duplicates and allowing for indexed access. Unlike dictionaries, lists do not use keys for retrieval, and elements are accessed by their position in the list.
Example:
List<int> myList = new List<int>() { 1, 2, 3, 4, 5 };
This code creates a List<int>
named myList
and initializes it with five integers. This list allows for ordered storage and retrieval of integers, and it supports operations like indexing and iteration.
Use the ToList()
Method to Convert a Dictionary to a List in C#
The ToList()
method is part of LINQ (Language Integrated Query) in C#. It is an extension method that operates on any IEnumerable<T>
type, which includes dictionaries. The purpose of ToList()
is to convert an enumerable type into a List<T>
.
Syntax:
List<T> list = source.ToList();
Here, source
is an IEnumerable<T>
type, which can be a dictionary. The method returns a List<T>
containing the elements of the source.
In C#, a dictionary can be converted into a list using the ToList()
method as a part of the System.Linq
extensions.
A dictionary cannot be converted to a List<string>
directly because the return type of dictionary is KeyCollection
. Meanwhile, a list is similar to an ArrayList
, the only difference being that a list is generic and has unique properties.
To convert dictionary values into a list collection, we can convert this generic list by its built-in ToList()
method. Both lists and dictionaries store data collections and are similar; both are random access data structures of the .NET
framework.
The dictionary is based on a hash table, an efficient algorithm for looking up things. On the other hand, a list goes element by element until it finds the result from the beginning to the result each time.
It’s easy to convert a dictionary into a list collection in C# using the System.Collections.Generic
and System.Linq
namespaces. Call ToList()
on a dictionary collection, yielding a list of KeyValuePair
instances.
Example:
// add `System.Collections.Generic` and `System.Linq` namespaces
using System;
using System.Collections.Generic;
using System.Linq;
class ConDtoLCol {
static void Main() {
// create a Dictionary
Dictionary<string, int> dict = new Dictionary<string, int>();
dict["cat"] = 1;
dict["dog"] = 4;
dict["mouse"] = 2;
dict["rabbit"] = -1;
// call ToList to convert the `dict` Dictionary to List
List<KeyValuePair<string, int>> list = dict.ToList();
// loop over the List to show successful conversion
foreach (KeyValuePair<string, int> pair in list) {
Console.WriteLine(pair.Key);
Console.WriteLine(" {0}", pair.Value);
}
}
}
This code demonstrates how to convert a Dictionary<string, int>
to a List<KeyValuePair<string, int>>
.
First, the necessary namespaces System.Collections.Generic
and System.Linq
are imported. Then, a class named ConDtoLCol
is defined.
Inside the Main
method, a Dictionary<string, int>
called dict
is created and populated with key-value pairs. The keys are strings, and the values are integers.
Next, the ToList()
method is used to convert the dictionary to a list of key-value pairs. This resulting list is then looped through, and each key and its corresponding value are printed to the console.
Output:
cat
1
dog
4
mouse
2
rabbit
-1
If you want to convert the keys of a dictionary to a list collection in C#, you can use:
List<string> listNumber = dicNumber.Keys.ToList();
Furthermore, to convert the values of a dictionary to a list collection in C#, you can use:
List<string> listNumber = dicNumber.Values.ToList();
The .NET
framework dictionary represents a collection of keys and values.
The primary purpose of dictionary keys is to allow users to get a generic list (collection) of the dictionary values. Furthermore, you can use the values
property to get that generic list of dictionary values.
Use KeyValuePair<TKey, TValue>
to Convert Dictionary to List in C#
In C#, the Dictionary<TKey, TValue>
class is a powerful data structure that allows us to store key-value pairs in a collection.
However, there are scenarios where we might need to convert a dictionary into a list of key-value pairs for various purposes, such as sorting, filtering, or performing specific operations. This is where the KeyValuePair<TKey, TValue>
class comes into play.
KeyValuePair<TKey, TValue>
is a struct defined in the System.Collections.Generic
namespace. It represents a single key-value pair and provides properties for accessing both the key and value.
Syntax:
struct KeyValuePair<TKey, TValue> {
public TKey Key { get; }
public TValue Value { get; }
public KeyValuePair(TKey key, TValue value);
}
Key
: This property represents the key in the key-value pair. It’s of typeTKey
.Value
: This property represents the value in the key-value pair. It’s of typeTValue
.KeyValuePair(TKey key, TValue value)
: This is the constructor that initializes a new instance of theKeyValuePair<TKey, TValue>
structure with the specified key and value.
This method involves creating a new list and passing the dictionary as an argument during initialization. The list is automatically populated with KeyValuePair<TKey, TValue>
elements.
Example code:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Create a sample dictionary
Dictionary<int, string> sampleDictionary =
new Dictionary<int, string>() { { 1, "Apple" }, { 2, "Banana" }, { 3, "Cherry" } };
// Convert the dictionary to a list using KeyValuePair<TKey, TValue>
List<KeyValuePair<int, string>> listUsingKeyValuePair =
new List<KeyValuePair<int, string>>(sampleDictionary);
// Display the elements in the list
Console.WriteLine("List elements using KeyValuePair<TKey, TValue>:");
foreach (var kvp in listUsingKeyValuePair) {
Console.WriteLine($"Key: {kvp.Key}, Value: {kvp.Value}");
}
}
}
This code demonstrates how to convert a dictionary into a list of KeyValuePair<int, string>
. In the Main
method, a sample dictionary sampleDictionary
is created with integer keys and string values.
Next, a list called listUsingKeyValuePair
is created by passing the sampleDictionary
as an argument to the List<KeyValuePair<int, string>>
constructor. This operation effectively converts the dictionary into a list of key-value pairs.
The code then proceeds to iterate through the listUsingKeyValuePair
using a foreach
loop. For each key-value pair (kvp
) in the list, it prints out the key and value using Console.WriteLine
.
Output:
List elements using KeyValuePair<TKey, TValue>:
Key: 1, Value: Apple
Key: 2, Value: Banana
Key: 3, Value: Cherry
This example showcases a useful conversion technique that can be employed when you need to work with dictionaries and lists interchangeably, utilizing the KeyValuePair<TKey, TValue>
structure.
Conclusion
In summary, this article guides on converting dictionaries to lists in C#. It highlights the distinctions between dictionaries (key-value pairs) and lists (ordered collections).
It introduces the ToList()
method, showcasing its syntax and usage. Additionally, it emphasizes using KeyValuePair<TKey, TValue>
to convert dictionaries to lists.
The article provides practical examples for both methods, empowering developers to efficiently manipulate data structures in C#.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHubRelated Article - Csharp Dictionary
- Best Way to Iterate Over a Dictionary in C#
- How to Sort a Dictionary by Its Keys in C#
- How to Check for the Existence of a Dictionary Key in C#
- Dictionary vs Hashtable in C#
- How to Convert a Dictionary to JSON String in C#