Dictionary vs Hashtable in C#
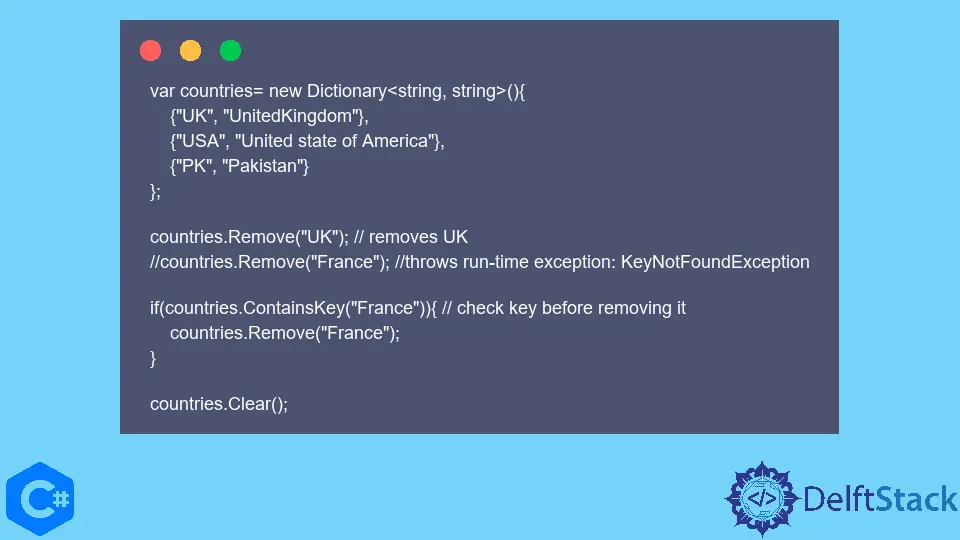
This guide will discuss the differences between Dictionary
and Hashtable
in C#.
To understand which of these collections is better and preferred, we need to understand their fundamentals. So, let’s go through the syntax, characteristics, functionalities, and methods associated with these two collections.
Which one should you prefer when working on C#? Which is better?
All these questions will be answered below. Let’s dive in!
Dictionary in C#
The Dictionary
is a generic collection. It stores data in key-value pairs, and this collection has no particular order.
The syntax of the Dictionary
is as follows:
Dictionary<TKey, TValue>
We pass the data values along with their keys. Let’s go through the characteristics of the Dictionary
.
Characteristics of the Dictionary in C#
- It stores key-value pairs.
- Its namespace is
System.Collections.Generic
. - The keys in the
Dictionary
must not be null and should be unique. - However, values can be null and duplicated.
- We can access the data value through its corresponding key, e.g.,
myDictionary[key]
. - All the elements are considered
KeyValuePair<TKey, TValue>
.
Create a Dictionary in C#
You can create a Dictionary
in C# by passing the type of values and their corresponding keys that it can store. Look at the following code to see how we create a Dictionary
.
IDictionary<int, string> rollno_names = new Dictionary<int, string>();
rollno_names.Add(1, "Ali"); // adding a key/value using the Add() method
rollno_names.Add(2, "Haider");
rollno_names.Add(3, "Saad");
// The following throws runtime exception: key already added.
// rollno_names.Add(3, "Rafay");
foreach (KeyValuePair<int, string> i in rollno_names)
Console.WriteLine("Key: {0}, Value: {1}", i.Key, i.Value);
// creating a dictionary using collection-initializer syntax
var countries = new Dictionary<string, string>() {
{ "UK", "United Kingdom" }, { "USA", "United States of America" }, { "PK", "Pakistan" }
};
foreach (var j in countries) Console.WriteLine("Key: {0}, Value: {1}", j.Key, j.Value);
In the code above, rollno_names
is a Dictionary
with int as key data type and string as value data type as follows:
Dictionary<int, string>
This particular Dictionary
can store int keys and string values. The second Dictionary
is of countries made by the collection-initializer.
Both of its keys and values are of string data types. If you try to duplicate the key-value and make it null, you will get a runtime exception.
Access a Dictionary Element in C#
You can access a Dictionary
element with the help of its indexer. You need to specify each key to access its corresponding value.
ElementAt()
is another approach to getting the key-value pair. Take a look at the following code.
var countries = new Dictionary<string, string>() {
{ "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" }
};
Console.WriteLine(countries["UK"]); // prints value of UK key
Console.WriteLine(countries["USA"]); // prints value of USA key
// Console.WriteLine(countries["France"]); // runtime exception: key does not exist
// use ContainsKey() to check for the unknown key
if (countries.ContainsKey("France")) {
Console.WriteLine(countries["France"]);
}
// use TryGetValue() to get a value of the unknown key
string result;
if (countries.TryGetValue("France", out result)) {
Console.WriteLine(result);
}
// use ElementAt() to retrieve the key-value pair using the index
for (int i = 0; i < countries.Count; i++) {
Console.WriteLine("Key: {0}, Value: {1}", countries.ElementAt(i).Key,
countries.ElementAt(i).Value);
}
In the above code, you can see that there is no key-value pair containing France
. So, the above code will give a runtime exception.
Update a Dictionary Element in C#
By specifying a key in the indexer, one can change/update the value of a key. If a key is not found in the Dictionary
, it will throw the KeyNotFoundException
exception; thus, use the ContainsKey()
function before accessing any unknown keys.
Take a look at the following code.
var countries = new Dictionary<string, string>() { { "UK", "London, Manchester, Birmingham" },
{ "USA", "Chicago, New York, Washington" },
{ "PK", "Pakistan" } };
countries["UK"] = "Europe"; // update value of UK key
countries["USA"] = "America"; // update value of USA key
// countries["France"] = "Western Europe"; //throws run-time exception: KeyNotFoundException
if (countries.ContainsKey("France")) {
countries["France"] = "Western Europe";
}
Remove a Dictionary Element in C#
A key-value pair already existing in a Dictionary
is removed using the Remove()
method. All Dictionary
elements are removed using the Clear()
method.
var countries = new Dictionary<string, string>() {
{ "UK", "UnitedKingdom" }, { "USA", "United state of America" }, { "PK", "Pakistan" }
};
countries.Remove("UK"); // removes UK
// countries.Remove("France"); //throws run-time exception: KeyNotFoundException
if (countries.ContainsKey("France")) { // check key before removing it
countries.Remove("France");
}
countries.Clear();
Hashtable in C#
Unlike the Dictionary
, Hashtable
is a non-generic collection. It also stores key-value pairs.
By computing the hash code for each key and storing it in a separate internal bucket, it improves lookups by matching the hash code of the supplied key when accessing values. Take a look at some characteristics of the Hashtable
.
Characteristics of the Hashtable in C#
- Key-value pairs are kept in
Hashtables
. - It belongs to the namespace
SystemCollection
. - The
IDictionary
interface is implemented. - Keys cannot be null and must be distinct.
- Values may be duplicated or null.
- Values can be obtained by providing the indexer with the relevant key, as in
Hashtable[key]
. DictionaryEntry
objects are used to store the elements.
Create a Hashtable in C#
Look at the following self-explanatory code to understand the creation of a Hashtable
.
`Hashtable` rollno_names = new `Hashtable`();
rollno_names.Add(1, "Ali"); // adding a key/value using the Add() method
rollno_names.Add(2, "Haider");
rollno_names.Add(3, "Saad");
// The following throws runtime exception: key already added.
// rollno_names.Add(3, "Rafay");
foreach (DictionaryEntry i in rollno_names)
Console.WriteLine("Key: {0}, Value: {1}", i.Key, i.Value);
// creating a `Hashtable` using collection-initializer syntax
var countries = new `Hashtable`() {
{ "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" }
};
foreach (DictionaryEntry j in countries) Console.WriteLine("Key: {0}, Value: {1}", j.Key, j.Value);
We can create the Hashtable
just like Dictionary
. The only difference is that it’s non-generic, so we don’t have to specify the data types of keys and their corresponding values.
Add Dictionary in the Hashtable in C#
We can create the object of the Dictionary
, and by simply passing that object while creating the Hashtable
, we can add the Dictionary
key-value pairs inside the Hashtable
.
Update the Hashtable in C#
By entering a key into the indexer, you can retrieve the value of an existing key from the Hashtable
. Since the Hashtable
is a non-generic collection (meaning no data types for both keys and values), type casting it to a string is required when getting values from it.
Take a look at the following code.
// creating a Hashtable using collection-initializer syntax
var countries = new Hashtable() {
{ { "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" } };
string countrynameUK = (string)countries["UK"]; // cast to string
string countrynameUSA = (string)countries["USA"]; // cast to string
Console.WriteLine(countrynameUK);
Console.WriteLine(countrynameUSA);
countries["UK"] = "Euorpe"; // update value of UK key
countries["USA"] = "America"; // update value of USA key
if (!countries.ContainsKey("France")) {
countries["France"] = "Western Euorpe";
}
Remove Elements From the Hashtable in C#
The given key-value pairs of a Hashtable
are removed using the Remove()
method. If the provided key is not present in the Hashtable
, it throws the KeyNotfoundException
exception; thus, before removing a key, use the ContainsKey()
method to see if it already exists.
Use the Clear()
function to remove every item at once.
var countries = new Hashtable() {
{ "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" }
};
countries.Remove("UK"); // removes UK
// countries.Remove("France"); //throws run-time exception: KeyNotFoundException
if (countries.ContainsKey("France")) { // check key before removing it
countries.Remove("France");
}
countries.Clear(); // removes all elements
Dictionary vs. Hashtable in C#
Dictionary |
Hashtable |
---|---|
If we attempt to discover a key that doesn’t exist, it either returns or throws an exception. | If we try to discover a key that doesn’t exist, it returns null. |
Because there is no boxing and unboxing, it is quicker than a Hashtable . |
Due to the boxing and unboxing required, it takes longer than a Dictionary . |
Thread safety is only available for public static members. | A Hashtable ’s members are all thread-safe, |
Since the Dictionary is a generic type, any data type can be used with it (When creating it, we must specify the data types for both keys and values). |
Not a generic type. |
Hashtable implementation with strongly typed Keys and Values is called Dictionary . |
Since Hashtables are flexibly typed data structures, any key and value types can be added. |
Based on these facts, we can say that Dictionary
is preferred over the Hashtable
. Because you can insert any random item into Dictionary<TKey, TValue>
and you don’t have to cast the values you take out, you gain type safety.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn