Best Way to Iterate Over a Dictionary in C#
-
Use the
for
Loop to Iterate Over a Dictionary inC#
-
Use
foreach
Loop to Iterate Over a Dictionary inC#
-
Use
ParallelEnumerable.ForAll
Method to Iterate a Dictionary inC#
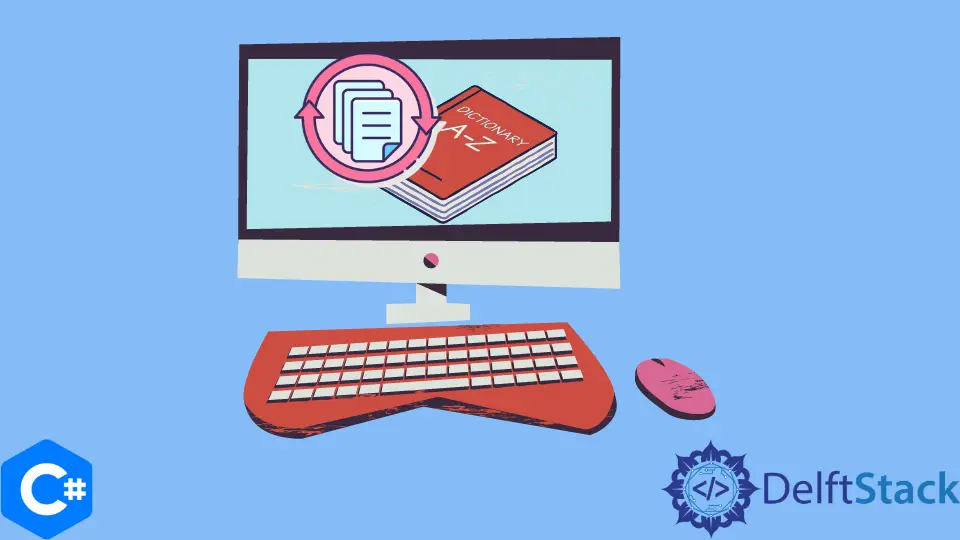
Dictionary in C# is a collection of key-value pairs. It is somewhat similar to the English dictionary where the key
represents a word and value
is its meaning. In this article, we will look at different ways of iterating a dictionary.
For representational purposes, we will consider the following definition of the Dictionary object throughout the article.
Dictionary<int, string> sample_Dict = new Dictionary<int, string>();
Now, Let’s look at some of the methods you can use to traverse this dictionary.
Use the for
Loop to Iterate Over a Dictionary in C#
for
loop method is easy and concise, where we sequentially iterate over the dictionary using an index.
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
for (int index = 0; index < sample_Dict.Count; index++)
System.Console.WriteLine(index + ":" + sample_Dict[index]);
}
}
Output:
0:value_1
1:value_2
2:value_3
As you will notice we have also specified the initializer list while defining the Dictionary object. While specifying the initializer list, make sure that each entry in the list has a unique key, otherwise, it will lead to Run-time
exception.
Use foreach
Loop to Iterate Over a Dictionary in C#
foreach
looping is another alternative that you can opt for.
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
foreach (var entry in sample_Dict) System.Console.WriteLine(entry.Key + ":" + entry.Value);
}
}
Output:
0:value_1
1:value_2
2:value_3
The above method can be considered a lazy implementation of foreach
loop. We can also implement foreach
method using KeyValuePair<TKey,TValue>
structure.
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
foreach (var entry in sample_Dict) System.Console.WriteLine(entry.Key + ":" + entry.Value);
}
}
Output:
0:value_1
1:value_2
2:value_3
Use ParallelEnumerable.ForAll
Method to Iterate a Dictionary in C#
This method comes in handy when you are iterating over large dictionaries, as it incorporates multithreading processing for each key-value pair in the dictionary.
using System;
using System.Linq;
using System.Collections.Generic;
public class Sample {
public static void Main() {
/*User Code Here*/
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
sample_Dict.AsParallel().ForAll(entry => Console.WriteLine(entry.Key + ":" + entry.Value));
}
}
Output:
0:value_1
1:value_2
2:value_3
When analyzing the best method out of the above mentioned, we need to consider certain parameters and the result would be circumstantial. Looping method is concise and effective but when iterating large dictionaries it becomes impractical in terms of run time complexity.
This is where ParallelEnumerable.ForAll
method comes in as it allows concurrent execution saving crucial time, which may be an important factor while designing certain applications.