How to Convert a Dictionary to JSON String in C#
- the Basic Structure of a JSON String
-
Use
JsonConvert.SerializeObject()
to Convert aDictionary
to JSON in C# -
Use a Custom Made Function to Convert a
Dictionary
to JSON in C# -
Use JavaScript
Serializer
in C# for.NET
Webapps - Conclusion
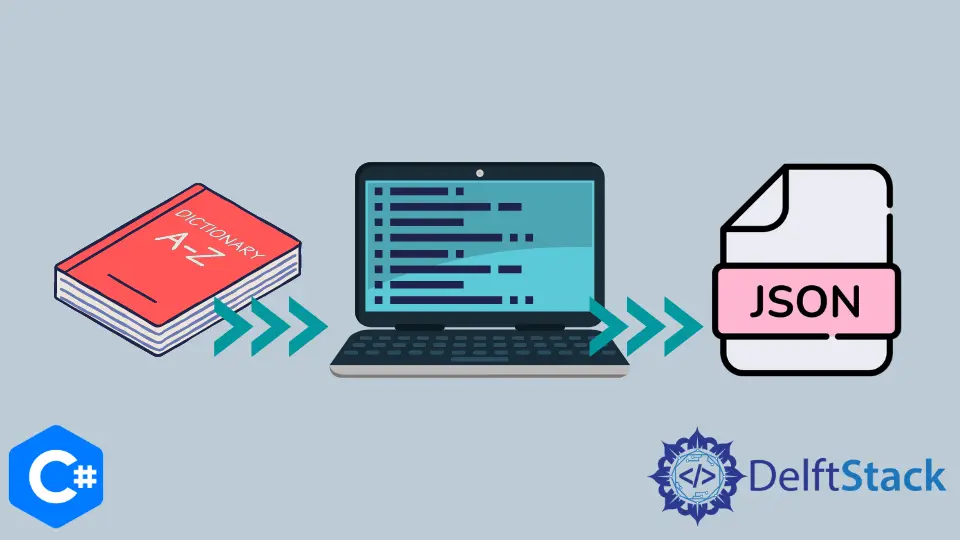
This article delves into the nuances of converting a Dictionary
to JSON in C#, an operation pivotal for data serialization and transmission across different systems and platforms. We will explore the process using various methods, including the JsonSerializer.Serialize
method and alternative approaches for scenarios where external libraries or specific serialization needs come into play.
This tailored guide for both beginners and experienced developers aims to provide comprehensive insights and practical code examples to proficiently handle this common yet crucial task in C# programming.
A Dictionary
in C# is a collection that stores key-value pairs. It allows quick retrieval of values based on their unique keys.
To use a Dictionary
, you must first include the System.Collections.Generic
namespace, which provides generic collection classes.
using System.Collections.Generic;
Once the namespace is imported, you can declare and initialize a Dictionary
within any method, typically within the Main
function for simple console applications. It’s important to note that the IDictionary<TKey, TValue>
interface can be used as a type for more generic code, but for instantiation, you use the concrete Dictionary<TKey, TValue>
class.
IDictionary<int, double> cgpas = new IDictionary<int, dictionary>();
Let’s go ahead now and see how we can convert a Dictionary
to JSON
.
the Basic Structure of a JSON String
JSON stands for JavaScript Object Notation
. It uses a sort of structure where values are mapped to a key.
So, if we want to store a set of employees in a store, let’s say we can proceed as follows.
{"employees" : {{"id" : 1 , "name" : "John"}, {"id" : 2, "name" : "Kevin"}}
Now, the above-given example may be a bit complex. But let’s break it down.
We are defining JSON first because you can get an insight into its structure and be better at breaking down how the conversions stated later on occur.
So we kick off with "Employees"
, which is the KEY
. And then, we see a curly bracket followed by 2
more curly brackets (close and open).
A curly bracket enclosure can also be called a set. It contains keys and values because we have only defined 2
different employees, JOHN
and KEVIN
; hence only two sets are defined.
These are enclosed in a bigger set, the value of the KEY EMPLOYEES
. Hence, if we now tend to call the EMPLOYEES
tag, we will receive this set as the value.
Use JsonConvert.SerializeObject()
to Convert a Dictionary
to JSON in C#
The primary purpose of converting a dictionary to JSON is to serialize the dictionary into a string format that can be easily transferred over networks, saved into files, or used in APIs. This is particularly useful in web services, APIs, and any client-server communication where C# backends need to send data to frontends or other services in a universally understandable format.
In C#, converting data structures such as dictionaries to JSON can be elegantly handled using the JsonConvert.SerializeObject
method from the Newtonsoft.Json
library, also known as Json.NET
.
The SerializeObject
method is highly versatile and powerful, making it a popular choice for C# developers dealing with JSON data.
Example:
using System;
using System.Collections.Generic;
using Newtonsoft.Json;
class Program {
static void Main() {
// Creating a dictionary
Dictionary<string, int> fruits =
new Dictionary<string, int> { { "Apple", 10 }, { "Banana", 5 }, { "Orange", 8 } };
// Converting the dictionary to a JSON string
string json = JsonConvert.SerializeObject(fruits, Formatting.Indented);
// Printing the result
Console.WriteLine(json);
}
}
In this code example, we start by creating a Dictionary<string, int>
named fruits
, where the keys represent fruit names and the values denote their quantities. As developers, we then leverage the power of JsonConvert.SerializeObject
to seamlessly convert this dictionary into a JSON string.
The method is straightforward; it takes our fruits
dictionary as an argument and transforms it into a JSON representation. The optional parameter Formatting.Indented
is employed to format the resulting JSON with indentation, enhancing its human readability during debugging or when presenting the data.
This formatting is particularly useful in our development workflow. Lastly, by utilizing the Console.WriteLine
statement, we print the JSON string to the console, allowing us to inspect the serialized data.
Output:
Use a Custom Made Function to Convert a Dictionary
to JSON in C#
In scenarios where using external libraries like Newtonsoft.Json
is not feasible, C# provides built-in methods such as string.Format
and string.Join
, which can be effectively used to convert a dictionary to a JSON string. This approach is particularly useful in situations where you want to avoid external dependencies or need a lightweight method for serialization.
This method is ideal for simple serialization tasks where you don’t require the full capabilities of a comprehensive JSON library. It’s commonly used in applications where the data structure is straightforward, and the overhead of an external library is unjustifiable.
Example:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
var dictionary = new Dictionary<string, string> {
{ "Name", "Alice" }, { "Age", "30" }, { "Country", "Wonderland" }
};
string json = DictionaryToJson(dictionary);
Console.WriteLine(json);
}
static string DictionaryToJson(Dictionary<string, string> dict) {
var keyValuePairs = dict.Select(kvp => string.Format("\"{0}\":\"{1}\"", kvp.Key, kvp.Value));
return "{" + string.Join(",", keyValuePairs) + "}";
}
}
In our implementation, we begin by creating a dictionary containing simple key-value pairs. In the DictionaryToJson
function, we use LINQ’s Select
method to format each key-value pair in the dictionary into a JSON property using string.Format
.
This method ensures each key and value is correctly enclosed in quotes. We then use string.Join
to concatenate these formatted strings, using a comma as the separator.
This results in a single string that represents the JSON object. By combining these methods, we create a concise and efficient way to serialize a dictionary to JSON without relying on external libraries.
Output:
Use JavaScript Serializer
in C# for .NET
Webapps
Suppose you are using web applications that use C# as the core. In that case, you are better off following a LIST
structure to define the objects and then using the JavaScriptSerializer()
function to convert it to a string.
The JsonSerializer.Serialize
method is part of the System.Text.Json
namespace in .NET
, introduced as a high-performance, low-allocating JSON serialization library.
Example:
using System;
using System.Collections.Generic;
using System.Text.Json;
class Program {
static void Main() {
// Create a dictionary
var personDictionary = new Dictionary<string, string> {
{ "Name", "John Doe" }, { "Age", "30" }, { "Country", "USA" }
};
// Convert dictionary to JSON
string jsonResult = JsonSerializer.Serialize(personDictionary);
// Print the JSON result
Console.WriteLine(jsonResult);
}
}
In this code snippet, we start by creating a dictionary named personDictionary
with string keys and values.
This dictionary holds simple data about a person. We then use the JsonSerializer.Serialize
method to convert this dictionary into a JSON string.
The serialization process iterates over the dictionary entries, converting each key-value pair into a JSON field.
We chose JsonSerializer
for its efficiency and compliance with modern JSON standards. It’s especially suited for applications targeting .NET
Core or later versions.
Moreover, JsonSerializer
supports custom serialization options, though they are not utilized in this basic example.
Output:
Conclusion
In conclusion, the conversion of a Dictionary
to JSON in C# is a fundamental skill in modern software development, essential for data exchange in web services, APIs, and numerous other applications. Throughout this article, we have explored various methods to achieve this, from using the built-in JsonSerializer.Serialize
method to employing external libraries like Newtonsoft.Json
, and even crafting custom serialization functions for specific requirements.
Each method offers unique advantages, whether it be the simplicity and direct support of JsonSerializer
, the extensive features of Newtonsoft.Json
, or the control and independence of custom functions. By understanding these techniques, developers can choose the most suitable approach for their specific context, ensuring efficient and effective data serialization in their C# applications. This knowledge not only enhances a developer’s toolkit but also contributes to building more robust, flexible, and interoperable software systems.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub