How to Sort a Dictionary by Its Keys in C#
-
Use the
OrderBy()
LINQ Method to Sort a Dictionary by Its Keys inC#
-
Use the
SortedList<TKey, TValue>
Class to Sort a Dictionary by Its Keys inC#
-
Use the
SortedDictionary<TKey, TValue>
Class to Sort a Dictionary by Its Keys inC#
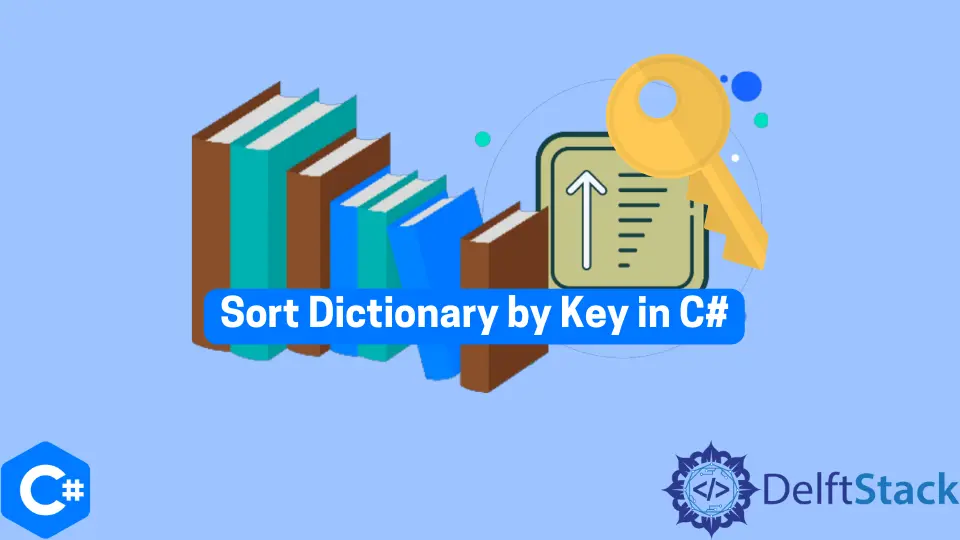
In C#, a dictionary allows near constant time lookups that reflect its implementation as a Hash Table
, which means it is also inherently unsorted. You can separately acquire the elements of a dictionary to sort them as a dictionary has no pre-defined sort method.
The first method is to use the OrderBy()
LINQ method; however, it doesn’t have the best performance O(n*log(n))
as it needs to sort all the entries. If you need repeated access to the dictionary elements by key, use SortedList
or SortedDictionary
because these can help you achieve this purpose without compromising on quality.
You can lose some performance in lookup and insertion times when extracting the list of elements in order in linear time O(n)
. In this tutorial, you will learn three possible methods to sort a dictionary by its keys in C#.
Use the OrderBy()
LINQ Method to Sort a Dictionary by Its Keys in C#
This LINQ method enables programmers to extract an IOrderedEnumerable
of KeyValueParts
from a dictionary to sort it by its keys. As a dictionary cannot store elements in order, the sorting of a dictionary will be O(n*log(n))
.
LINQ methods belong to the System.Linq
namespace and can enable you to easily process every key-value pair for each key in the sorted dictionary. The OrderBy
method is implemented by deferred execution and immediately returns an object that stores all the information required to sort a dictionary.
It performs a stable sort, and if the keys of two elements are equal, the order of the elements is preserved.
Example Code:
using System;
using System.Linq;
using System.Collections.Generic;
public class sortOrderBy {
public static void Main(string[] args) {
var metal = new Dictionary<string, int> {
["Platinum"] = 7,
["Aluminum"] = 4,
["Titanium"] = 3,
};
foreach (var console in metal.OrderBy(x => x.Key)) {
Console.WriteLine(console);
}
}
}
Output:
[Aluminum, 4]
[Platinum, 7]
[Titanium, 3]
An alternative LINQ method to OrderBy
is OrderByDesending
, which does as its name suggests - it reverses the order. As it’s a type of IOrderedEnumerable<TElement>
, the ThenBy
and ThenByDescending
methods also enable you to specify additional sort criteria to sort a dictionary.
Additionally, LINQ Reverse
method is an equivalent of the OrderByDescending
method to sort a dictionary in descending order.
Example Code:
using System;
using System.Linq;
using System.Collections.Generic;
public class sortOrderByDescending {
public static void Main(string[] args) {
var metal = new Dictionary<string, int> {
["Platinum"] = 7,
["Aluminum"] = 4,
["Titanium"] = 3,
};
// LINQ `Reverse` method, which gives the similar output
/*
foreach (var item in fruit.Reverse())
{
Console.WriteLine(console);
}
*/
foreach (var console in metal.OrderByDescending(x => x.Key)) {
Console.WriteLine(console);
}
}
}
Output:
[Titanium, 3]
[Platinum, 7]
[Aluminum, 4]
Use the SortedList<TKey, TValue>
Class to Sort a Dictionary by Its Keys in C#
Its name can become misleading for some as this method or data structure maps keys to its values and can act similar to a dictionary. It uses a Binary Search approach to find values by keys and store them in a particular order to sort a dictionary.
You can use the SortedList<TKey, TValue>
method to sort a dictionary by key in a configurable way in case you pass an IEqualityComparer<T>
into the constructor. Internally, it is implemented using a list effectively, so instead of looking up by hash code, it does a Binary Search.
It is useful doesn’t mean that it’s performance-wise optimized as it can build a large list but can avoid complexity. It belongs to the System.Collections.Generic
namespace and is based on the associated IComparer<T>
implementation.
Example Code:
using System;
using System.Collections.Generic;
public class sortedListExp {
public static void Main(string[] args) {
var metal = new SortedList<string, int> {
["Platinum"] = 7,
["Aluminum"] = 4,
["Titanium"] = 3,
};
foreach (var console in metal) {
Console.WriteLine(console);
}
}
}
Output:
[Aluminum, 4]
[Platinum, 7]
[Titanium, 3]
Use the SortedDictionary<TKey, TValue>
Class to Sort a Dictionary by Its Keys in C#
It represents a dictionary where key-value pairs are sorted on the key, as it’s implemented as a Binary Search tree with a logarithmic time retrieval operation. It initializes a new instance of a dictionary (containing the same mapping as the specified dictionary) and sorts it according to its keys.
It belongs to a Generic class, a binary search tree with O(log n)
retrieval, where n
represents the number of elements in the dictionary. It’s similar to the SortedList<TKey, TValue>
but has faster insertion and removal operations for unsorted data.
As it’s implemented as a Red-Black
tree while a dictionary is implemented as a hash table, using SortedDictionary
can negatively impact the performance. However, if there’s no significant performance difference, using SortedDictionary
can cause less confusion.
Example Code:
using System;
using System.Collections.Generic;
public class sortedDictionaryExp {
public static void Main(string[] args) {
var metal = new SortedDictionary<string, int> {
["Platinum"] = 7,
["Aluminum"] = 4,
["Titanium"] = 3,
};
foreach (var console in metal) {
Console.WriteLine(console);
}
}
}
Output:
[Aluminum, 4]
[Platinum, 7]
[Titanium, 3]
This tutorial contains everything to discuss how to sort a dictionary by its keys, and it might clear up any confusion there might have been around this topic. Additionally, it might have brought some new methods or data structures to your attention to fulfill this task while maintaining the ultimate performance.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub