How to Check for the Existence of a Dictionary Key in C#
-
Use
try-catch
to Check for the Existence of a Dictionary Key inC#
-
Use
ContainsKey()
to Check for the Existence of a Dictionary Key inC#
-
Use
TryGetValue()
to Check for the Existence of a Dictionary Key inC#
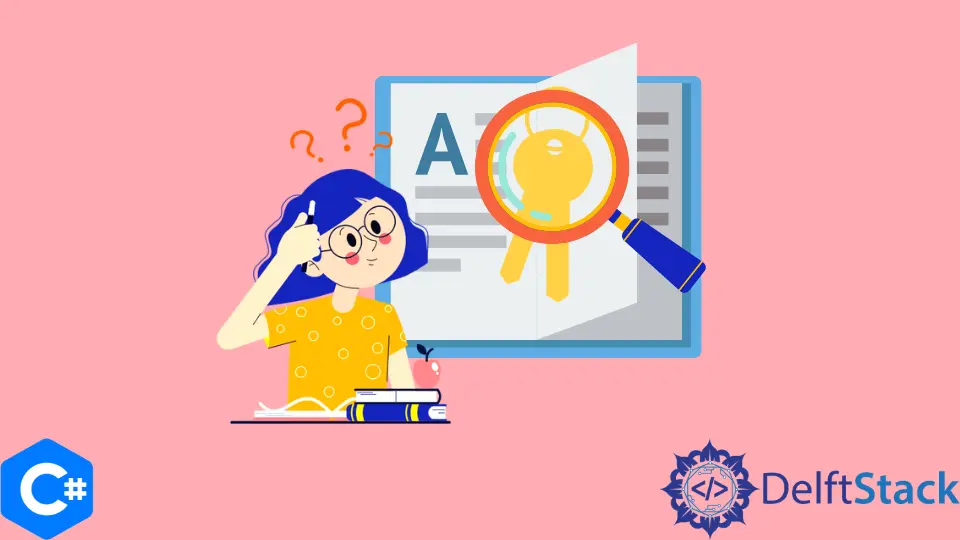
A Dictionary
tends to map keys and values. It contains a specific key to which a particular value is mapped. Duplicate keys are not allowed, and that is the entire goal of a Dictionary
.
Today we will be looking at how we can check if a key already exists in a Dictionary
or not.
Use try-catch
to Check for the Existence of a Dictionary Key in C#
Let’s first start with creating a dictionary called students
that maps each specific student ID to the CGPA:
Dictionary<int, double> students = new Dictionary<int, double>();
students[101] = 3.22;
students[102] = 3.44;
students[103] = 2.98;
Because the CGPAs are supposed to be in double format, we have defined the <TKEY, TVALUE>
as <int, double>
respectively for IDs and CGPAs.
Let’s say we want to find if there exists an entry for the student 102
in students
. It does, but we need a code to tell us straight.
So to do that, we can use a try-catch
exception as follows:
try {
Console.WriteLine(students[102].ToString());
} catch (Exception e) {
Console.WriteLine("No such key present");
}
OUTPUT:
3.44
But if we now try to do the same thing for student 104
, the following happens:
OUTPUT:
No such key present
Why? Well, the ToString()
method only works for values that are not null. Because student 104
is null and has no entry in students
, an exception occurs.
And rather than throwing the exception, we tend to catch it and present the message No such key present
in the console.
So it is an effective way to check whether a key is present. But can we make it simpler?
Use ContainsKey()
to Check for the Existence of a Dictionary Key in C#
Observe the following code:
if (!students.ContainsKey(104)) {
Console.WriteLine("No such key present");
}
This will present an error because no such key is present.
OUTPUT:
No such key present
Use TryGetValue()
to Check for the Existence of a Dictionary Key in C#
If we do the following:
double getval = 0.0;
if (!students.TryGetValue(104, out getval)) {
Console.WriteLine("No such key present");
}
This tends to return the output as follows:
No such key present
The TryGetValue()
tends to obtain a value for a key if it exists. The value is obtained in the second parameter.
Hence it is sent with the out
keyword. And the variable to get this value is declared before the scope, which is getval
.
The full code for all of these different methods has been included below:
using System;
using System.Collections.Generic;
using System.Text;
namespace jinlku_console {
class coder {
static void Main(String[] args) {
Dictionary<int, double> students = new Dictionary<int, double>();
students[101] = 3.22;
students[102] = 3.44;
students[103] = 2.98;
// TRY CATCH BLOCK
try {
Console.WriteLine(students[104].ToString());
} catch (Exception e) {
Console.WriteLine("No such key present");
}
// CONTAINS KEY METHOD
if (!students.ContainsKey(104)) {
Console.WriteLine("No such key present");
}
// TRYGETVALUE
double getval = 0.0;
if (!students.TryGetValue(104, out getval)) {
Console.WriteLine("No such key present");
}
}
}
}
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub