在 C# 中检查字典键是否存在
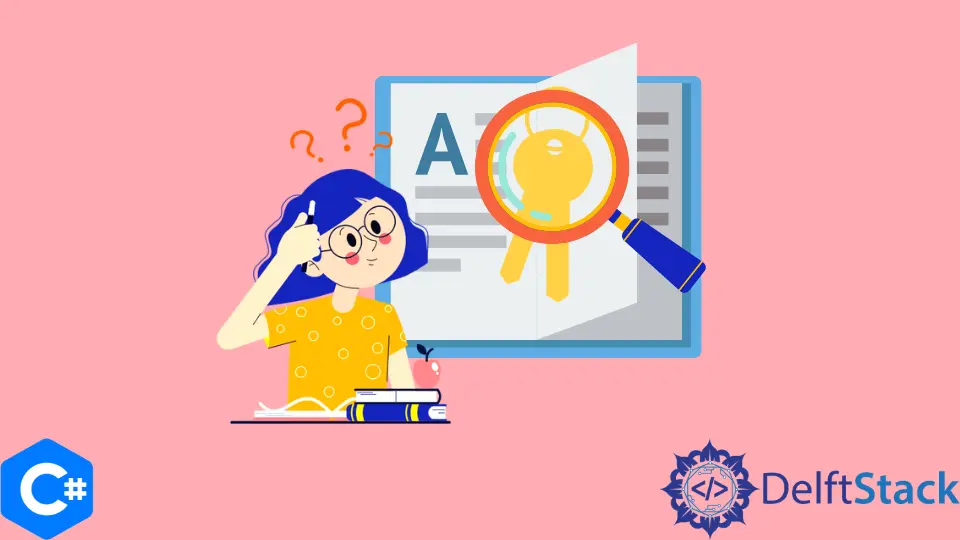
Dictionary
倾向于映射键和值。它包含特定值映射到的特定键。不允许有重复的键,这是字典
的全部目标。
今天我们将研究如何检查一个键是否已经存在于一个字典
中。
在 C#
中使用 try-catch
检查是否存在字典键
让我们首先创建一个名为 students
的字典,将每个特定的学生 ID 映射到 CGPA:
Dictionary<int, double> students = new Dictionary<int, double>();
students[101] = 3.22;
students[102] = 3.44;
students[103] = 2.98;
因为 CGPA 应该是双精度格式,所以我们将 ID 和 CGPA 分别定义为 <TKEY, TVALUE>
为 <int, double>
。
假设我们想在 students
中查找学生 102
是否存在条目。确实如此,但我们需要一个代码来直接告诉我们。
为此,我们可以使用 try-catch
异常,如下所示:
try {
Console.WriteLine(students[102].ToString());
} catch (Exception e) {
Console.WriteLine("No such key present");
}
输出:
3.44
但是如果我们现在尝试对学生 104
做同样的事情,会发生以下情况:
输出:
No such key present
为什么?好吧,ToString()
方法仅适用于非空值。因为学生 104
为 null 并且在 students
中没有条目,所以会发生异常。
而不是抛出异常,我们倾向于捕获它并在控制台中显示消息 No such key present
。
因此,这是检查密钥是否存在的有效方法。但是我们可以让它更简单吗?
在 C#
中使用 ContainsKey()
检查是否存在字典键
观察以下代码:
if (!students.ContainsKey(104)) {
Console.WriteLine("No such key present");
}
这将出现错误,因为不存在这样的键。
输出:
No such key present
在 C#
中使用 TryGetValue()
检查是否存在字典键
如果我们执行以下操作:
double getval = 0.0;
if (!students.TryGetValue(104, out getval)) {
Console.WriteLine("No such key present");
}
这往往会返回如下输出:
No such key present
TryGetValue()
倾向于获取键的值(如果存在)。该值在第二个参数中获得。
因此,它使用 out
关键字发送。获取该值的变量在作用域之前声明,即 getval
。
所有这些不同方法的完整代码已包含在下面:
using System;
using System.Collections.Generic;
using System.Text;
namespace jinlku_console {
class coder {
static void Main(String[] args) {
Dictionary<int, double> students = new Dictionary<int, double>();
students[101] = 3.22;
students[102] = 3.44;
students[103] = 2.98;
// TRY CATCH BLOCK
try {
Console.WriteLine(students[104].ToString());
} catch (Exception e) {
Console.WriteLine("No such key present");
}
// CONTAINS KEY METHOD
if (!students.ContainsKey(104)) {
Console.WriteLine("No such key present");
}
// TRYGETVALUE
double getval = 0.0;
if (!students.TryGetValue(104, out getval)) {
Console.WriteLine("No such key present");
}
}
}
}
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub