C# 中遍历字典的最佳方法
Puneet Dobhal
2023年10月12日
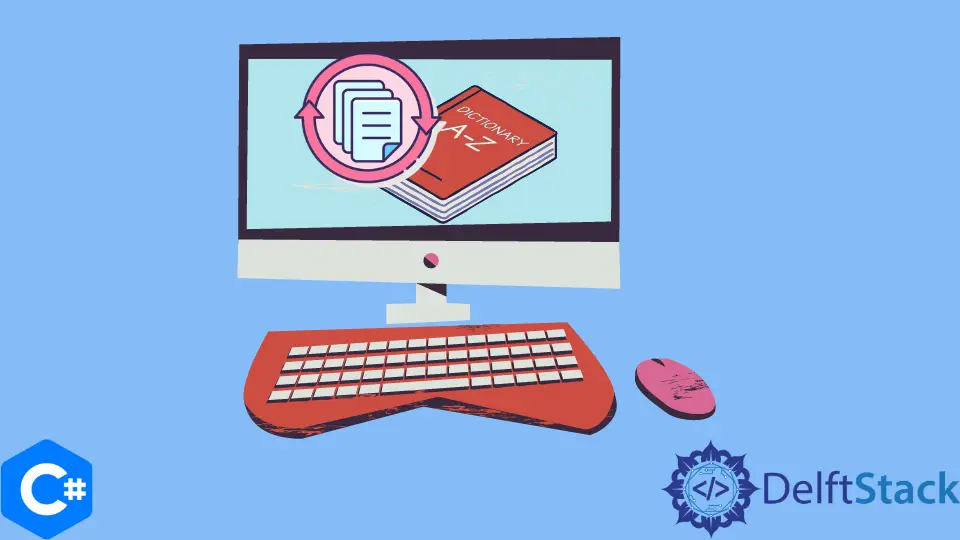
C# 中的字典是键值对的集合。它有点类似于英语字典,其中 key
代表一个单词,而 value
是其含义。在本文中,我们将研究迭代字典的不同方法。
出于表示目的,我们将在整篇文章中用以下 Dictionary
对象的定义。
Dictionary<int, string> sample_Dict = new Dictionary<int, string>();
现在,让我们看一下可用于遍历此字典的一些方法。
使用 for
循环迭代 C# 中的字典
for 循环方法简单明了,我们使用索引依次遍历字典。
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
for (int index = 0; index < sample_Dict.Count; index++)
System.Console.WriteLine(index + ":" + sample_Dict[index]);
}
}
输出:
0:value_1
1:value_2
2:value_3
你会注意到,在定义 Dictionary
对象时,我们还指定了初始化列表。指定初始化程序列表时,请确保列表中的每个元素都具有唯一键,否则,将导致运行时异常。
使用 foreach
循环遍历 C# 中的字典
foreach
循环是我们可以选择的另一种方法。
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
foreach (var entry in sample_Dict) System.Console.WriteLine(entry.Key + ":" + entry.Value);
}
}
输出:
0:value_1
1:value_2
2:value_3
可以将上述方法视为 foreach
循环的惰性实现。我们还可以使用 KeyValuePair<TKey,TValue>
结构来实现 foreach
方法。
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
foreach (var entry in sample_Dict) System.Console.WriteLine(entry.Key + ":" + entry.Value);
}
}
输出:
0:value_1
1:value_2
2:value_3
使用 ParallelEnumerable.ForAll
方法迭代 C# 中的字典
当你遍历大型字典时,此方法会派上用场,因为它为字典中的每个键值对合并了多线程处理。
using System;
using System.Linq;
using System.Collections.Generic;
public class Sample {
public static void Main() {
/*User Code Here*/
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
sample_Dict.AsParallel().ForAll(entry => Console.WriteLine(entry.Key + ":" + entry.Value));
}
}
输出:
0:value_1
1:value_2
2:value_3
在分析上述最佳方法时,我们需要考虑某些参数,其结果将是偶然的。循环方法简洁有效,但是在迭代大型字典时,就运行时复杂性而言,它变得不切实际。
这是 ParallelEnumerable.ForAll
方法出现的地方,因为它允许并发执行节省关键时间,这可能是设计某些应用程序时的重要因素。