在 C# 中更新字典值
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp Dictionary
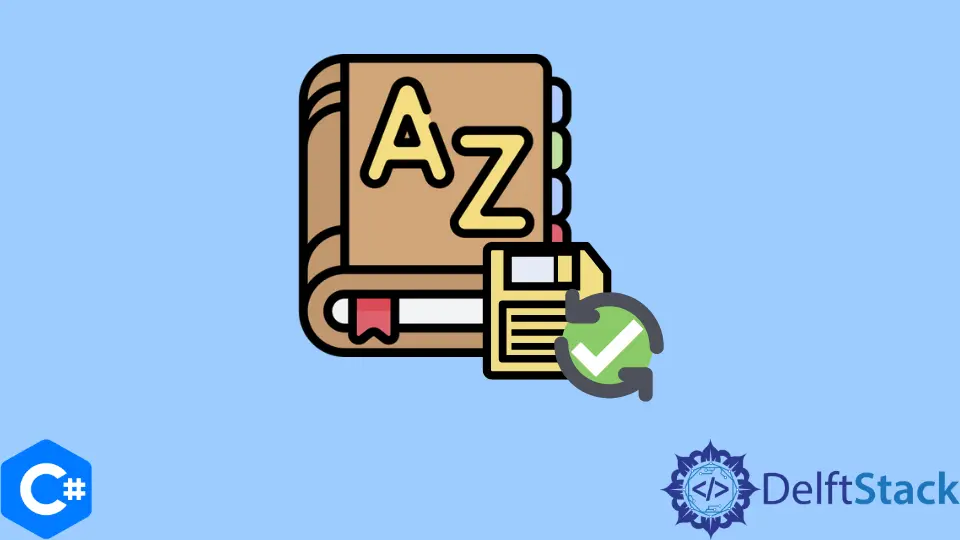
本教程将演示如何更新 C# 字典中的现有值。
dictionary
是一种集合类型,与只能通过索引或值本身访问值的数组或列表不同,字典使用键和值对来存储其数据。
Dictionary<TKey, TValue>() dict = new Dictionary<TKey, TValue>();
字典中的键和值可以定义为使用任何类型,但必须在整个字典中保持一致。
字典中的键必须具有以下特征:
- 键不能为空
- 键必须是唯一的;不允许重复
- 键必须与初始化时定义的类型相同
要引用字典中的值,你必须使用它的键作为索引。从那里,你可以编辑现有值。
例子:
using System;
using System.Collections.Generic;
namespace UpdateDictionary_Example {
class Program {
static void Main(string[] args) {
// Initializing the dictionary with a STRING key and a DOUBLE value
Dictionary<string, double> dict = new Dictionary<string, double>();
// Creating a new dictionary key with the initial value
dict["Apple"] = 1.99;
Console.WriteLine("An apple costs: " + dict["Apple"].ToString());
// Editing the value of the item with the key value "Apple"
dict["Apple"] = 2.99;
Console.WriteLine("An apple now costs: " + dict["Apple"].ToString());
}
}
}
在上面的示例中,字典首先被初始化,将其键定义为字符串,将其值定义为双精度。你可以观察到,由于字典在更新其值之前首先检查键是否存在,因此将新记录添加到字典和更新记录的语法是相同的。字典的 Apple
成本与其初始成本和更新成本一起打印。
输出:
An apple costs: 1.99
An apple now costs: 2.99
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe