How to Update Dictionary Value in C#
-
Updating Dictionary Values in C# Using
Indexer
-
Updating Dictionary Values in C# Using the
TryGetValue
-
Updating Dictionary Values in C# Using the
ContainsKey
-
Updating Dictionary Values in C# Using
ContainsKey
andIncrement
-
Updating Dictionary Values in C# Using
TryAdd
- Conclusion
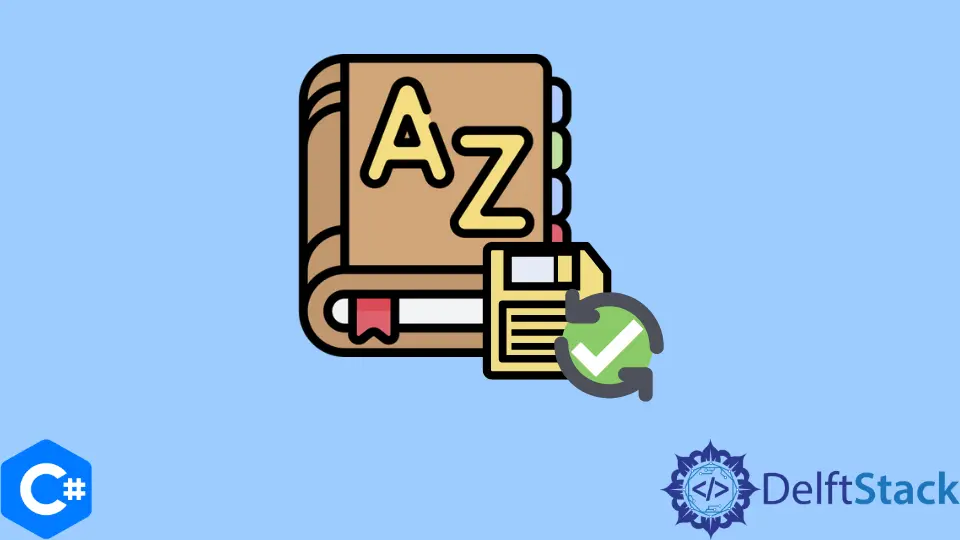
In C# programming, dictionaries serve as indispensable tools for efficiently managing key-value pairs. The ability to dynamically update values within a dictionary is a common requirement in various scenarios.
However, scenarios often arise where the need to update the value of a specific key within a dictionary dynamically becomes apparent. This tutorial will demonstrate how to update an existing value in a C# dictionary.
Updating Dictionary Values in C# Using Indexer
A dictionary is a type of collection that, unlike an array or a list whose values can only be accessed by the index or the value itself, a dictionary uses key and value pairs to store its data.
The keys and values in a dictionary can be defined as using any type but must be consistent throughout the entire dictionary. To reference the value within the dictionary, you must use its key as the index.
Consider a practical example where the task at hand involves maintaining a record of student scores using a dictionary. Initially, scores are assigned to students, and later, there’s a requirement to update a student’s score based on new information.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a dictionary to store student scores
Dictionary<string, int> studentScores = new Dictionary<string, int>();
// Adding initial scores
studentScores["John"] = 85;
studentScores["Alice"] = 92;
studentScores["Bob"] = 78;
// Updating John's score using the Indexer
studentScores["John"] = 90;
// Displaying the updated scores
foreach (var kvp in studentScores) Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
A dictionary named studentScores
is created to store the scores of different students. Initially, scores are assigned to three students: John
, Alice
, and Bob
.
The pivotal moment occurs when we decide to update John’s score. Instead of removing the existing entry and adding a new one, the Indexer method allows us to directly modify John’s score in a single line: studentScores["John"] = 90
.
This line replaces John’s previous score of 85
with the updated score of 90
.
In order to see the impact of this update, a foreach
loop is used to iterate through the dictionary and display the student names along with their updated scores.
In this loop, kvp
stands for key-value pair
, and each student’s name (kvp.Key
) is printed along with their updated score (kvp.Value
).
Output:
John: 90
Alice: 92
Bob: 78
Updating Dictionary Values in C# Using the TryGetValue
The TryGetValue
method is often associated with dictionary or map-like data structures.
The purpose of this method is to attempt to retrieve a value associated with a specified key from the dictionary without causing an exception if the key is not present. Instead of throwing an exception, it returns a boolean value indicating whether the retrieval was successful.
Let’s explore this concept with a practical example. Imagine you have a dictionary representing the scores of students, and you need to update a student’s score based on new information.
The TryGetValue
method proves to be a valuable tool in handling scenarios where the key might not exist in the dictionary.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a dictionary to store student scores
Dictionary<string, int> studentScores = new Dictionary<string, int>();
// Adding initial scores
studentScores["John"] = 85;
studentScores["Alice"] = 92;
studentScores["Bob"] = 78;
// Updating John's score using the TryGetValue method
UpdateStudentScore(studentScores, "John", 90);
// Displaying the updated scores
foreach (var kvp in studentScores) {
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
static void UpdateStudentScore(Dictionary<string, int> scores, string studentName, int newScore) {
// Try to get the existing score for the student
if (scores.TryGetValue(studentName, out int currentScore)) {
// Update the score if the student is found
scores[studentName] = newScore;
} else {
// Handle the case when the student is not found
Console.WriteLine($"Student '{studentName}' not found in the dictionary.");
}
}
}
A dictionary named studentScores
is created to store the scores of different students. Initially, scores are assigned to three students: John
, Alice
, and Bob
.
The pivotal moment occurs when we decide to update John’s score. Instead of removing the existing entry and adding a new one, the Indexer method allows us to directly modify John’s score in a single line: studentScores["John"] = 90
.
This line replaces John’s previous score of 85
with the updated score of 90
.
In order to see the impact of this update, a foreach
loop is used to iterate through the dictionary and display the student names along with their updated scores.
In this loop, kvp
stands for key-value pair
, and each student’s name (kvp.Key
) is printed along with their updated score (kvp.Value
).
Output:
John: 90
Alice: 92
Bob: 78
Updating Dictionary Values in C# Using the ContainsKey
When it comes to updating values dynamically, the ContainsKey
method serves as a valuable tool. This method allows developers to check whether a dictionary contains a specific key before attempting to update its associated value, providing a robust and error-resistant approach.
Let’s explore this concept through a practical example. Imagine you are tasked with maintaining a record of student scores using a dictionary, and you need to update a student’s score based on new information.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a dictionary to store student scores
Dictionary<string, int> studentScores = new Dictionary<string, int>();
// Adding initial scores
studentScores["John"] = 85;
studentScores["Alice"] = 92;
studentScores["Bob"] = 78;
// Updating John's score using the ContainsKey method
UpdateStudentScore(studentScores, "John", 90);
// Displaying the updated scores
foreach (var kvp in studentScores) {
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
static void UpdateStudentScore(Dictionary<string, int> scores, string studentName, int newScore) {
// Check if the dictionary contains the specified student name
if (scores.ContainsKey(studentName)) {
// Update the student's score if the key exists
scores[studentName] = newScore;
} else {
// Handle the case when the key is not found
Console.WriteLine($"Student '{studentName}' not found in the dictionary.");
}
}
}
We have a dictionary named studentScores
that initially contains scores for three students: John
, Alice
, and Bob
.
The crucial part of the code comes when we need to update John’s score using the UpdateStudentScore
method, which utilizes the ContainsKey
method UpdateStudentScore(studentScores, "John", 90);
.
Now, let’s delve into the UpdateStudentScore
method. This method takes the dictionary, the student’s name, and the new score as parameters.
The first step involves checking if the dictionary contains the specified student name using the ContainsKey
method. If the key exists, the method proceeds to update the student’s score; otherwise, it gracefully handles the case when the key is not found.
Output:
John: 90
Alice: 92
Bob: 78
Updating Dictionary Values in C# Using ContainsKey
and Increment
When it comes to updating values dynamically, combining the ContainsKey
method with the increment operation proves to be a powerful technique. This approach allows you to check whether a dictionary contains a specific key and, if so, increment its associated value.
Consider a scenario where you are tasked with tracking the number of occurrences of items in a dictionary. You want to update the count for a particular item, and if the item is not present in the dictionary, you want to add it with an initial count of 1.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a dictionary to store item counts
Dictionary<string, int> itemCounts = new Dictionary<string, int>();
// Incrementing counts for items
UpdateItemCount(itemCounts, "Apple");
UpdateItemCount(itemCounts, "Banana");
UpdateItemCount(itemCounts, "Apple");
UpdateItemCount(itemCounts, "Orange");
// Displaying the updated item counts
foreach (var kvp in itemCounts) {
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
static void UpdateItemCount(Dictionary<string, int> counts, string itemName) {
// Check if the dictionary contains the specified item
if (counts.ContainsKey(itemName)) {
// If the item exists, increment its count
counts[itemName]++;
} else {
// If the item does not exist, add it with an initial count of 1
counts[itemName] = 1;
}
}
}
We have a dictionary named itemCounts
that initially represents the counts of different items. We increment the counts for Apple
, Banana
, Apple
again, and Orange
using the UpdateItemCount
method.
The UpdateItemCount
method takes the dictionary and the item name as parameters. It begins by checking if the dictionary contains the specified item using the ContainsKey
method.
If the item is found, the method increments its count using the increment operation (counts[itemName]++
).
If the item is not found, it adds the item to the dictionary with an initial count of 1.
Output:
Apple: 2
Banana: 1
Orange: 1
Updating Dictionary Values in C# Using TryAdd
The TryAdd
method is not a built-in method for all dictionaries in C#. However, it is commonly associated with concurrent dictionaries in the System.Collections.Concurrent
namespace, which provides thread-safe operations for concurrent programming scenarios.
In the context of concurrent dictionaries, the TryAdd
method attempts to add a new key-value pair to the dictionary, but it does so in a way that avoids race conditions when multiple threads are accessing the dictionary simultaneously.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a dictionary to store item quantities
Dictionary<string, int> itemQuantities = new Dictionary<string, int>();
// Updating item quantities using TryAdd
UpdateItemQuantity(itemQuantities, "Apple", 3);
UpdateItemQuantity(itemQuantities, "Banana", 2);
UpdateItemQuantity(itemQuantities, "Apple", 2);
UpdateItemQuantity(itemQuantities, "Orange", 5);
// Displaying the updated item quantities
foreach (var kvp in itemQuantities) {
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
static void UpdateItemQuantity(Dictionary<string, int> quantities, string itemName,
int quantity) {
// Try to add the item with its quantity
if (quantities.TryAdd(itemName, quantity)) {
// If the item was added (key did not exist), no further action is needed
} else {
// If the item already existed, update its quantity
quantities[itemName] += quantity;
}
}
}
We have a dictionary named itemQuantities
that initially represents the quantities of different items. We update the quantities for Apple
(adding it with a quantity of 3), Banana
(adding it with a quantity of 2), Apple
again (updating its quantity to 2), and Orange
(adding it with a quantity of 5) using the UpdateItemQuantity
method.
The UpdateItemQuantity
method takes the dictionary, the item name, and the quantity as parameters. It begins by attempting to add the item to the dictionary using the TryAdd
method.
If the item is added successfully (meaning the key did not exist), no further action is needed. If the item already exists, the method updates its quantity by adding the specified quantity.
Output:
Apple: 5
Banana: 2
Orange: 5
Conclusion
In the diverse landscape of C# programming, this tutorial has explored various techniques for updating dictionary values, each method offering unique advantages. From the simplicity of the Indexer
method to the safety of TryGetValue
, the robustness of ContainsKey
, and the streamlined efficiency of TryAdd
, developers have a rich toolkit to handle dynamic updates effectively.
Whether managing student scores or item quantities, these methods contribute to clean and readable code, enhancing the maintainability of applications. By understanding and employing these approaches judiciously, developers can navigate the intricacies of dictionary updates, ensuring reliability and efficiency in their C# projects.