C# 中遍歷字典的最佳方法
Puneet Dobhal
2023年10月12日
Csharp
Csharp Dictionary
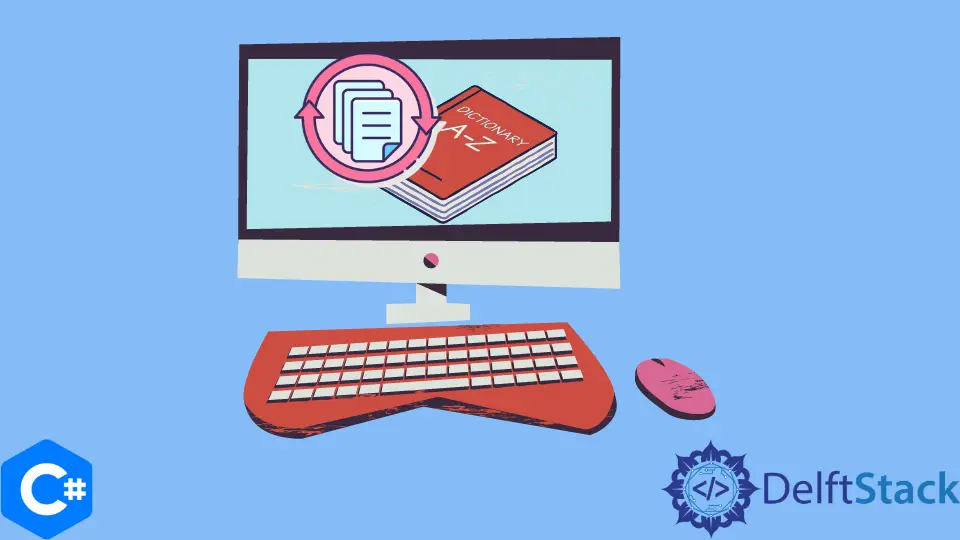
C# 中的字典是鍵值對的集合。它有點類似於英語字典,其中 key
代表一個單詞,而 value
是其含義。在本文中,我們將研究迭代字典的不同方法。
出於表示目的,我們將在整篇文章中用以下 Dictionary
物件的定義。
Dictionary<int, string> sample_Dict = new Dictionary<int, string>();
現在,讓我們看一下可用於遍歷此字典的一些方法。
使用 for
迴圈迭代 C# 中的字典
for 迴圈方法簡單明瞭,我們使用索引依次遍歷字典。
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
for (int index = 0; index < sample_Dict.Count; index++)
System.Console.WriteLine(index + ":" + sample_Dict[index]);
}
}
輸出:
0:value_1
1:value_2
2:value_3
你會注意到,在定義 Dictionary
物件時,我們還指定了初始化列表。指定初始化程式列表時,請確保列表中的每個元素都具有唯一鍵,否則,將導致執行時異常。
使用 foreach
迴圈遍歷 C# 中的字典
foreach
迴圈是我們可以選擇的另一種方法。
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
foreach (var entry in sample_Dict) System.Console.WriteLine(entry.Key + ":" + entry.Value);
}
}
輸出:
0:value_1
1:value_2
2:value_3
可以將上述方法視為 foreach
迴圈的惰性實現。我們還可以使用 KeyValuePair<TKey,TValue>
結構來實現 foreach
方法。
using System;
using System.Collections.Generic;
public class Sample {
public static void Main() {
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
foreach (var entry in sample_Dict) System.Console.WriteLine(entry.Key + ":" + entry.Value);
}
}
輸出:
0:value_1
1:value_2
2:value_3
使用 ParallelEnumerable.ForAll
方法迭代 C# 中的字典
當你遍歷大型字典時,此方法會派上用場,因為它為字典中的每個鍵值對合並了多執行緒處理。
using System;
using System.Linq;
using System.Collections.Generic;
public class Sample {
public static void Main() {
/*User Code Here*/
Dictionary<int, string> sample_Dict =
new Dictionary<int, string>() { { 0, "value_1" }, { 1, "value_2" }, { 2, "value_3" } };
sample_Dict.AsParallel().ForAll(entry => Console.WriteLine(entry.Key + ":" + entry.Value));
}
}
輸出:
0:value_1
1:value_2
2:value_3
在分析上述最佳方法時,我們需要考慮某些引數,其結果將是偶然的。迴圈方法簡潔有效,但是在迭代大型字典時,就執行時複雜性而言,它變得不切實際。
這是 ParallelEnumerable.ForAll
方法出現的地方,因為它允許併發執行節省關鍵時間,這可能是設計某些應用程式時的重要因素。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe