在 C# 中檢查字典鍵是否存在
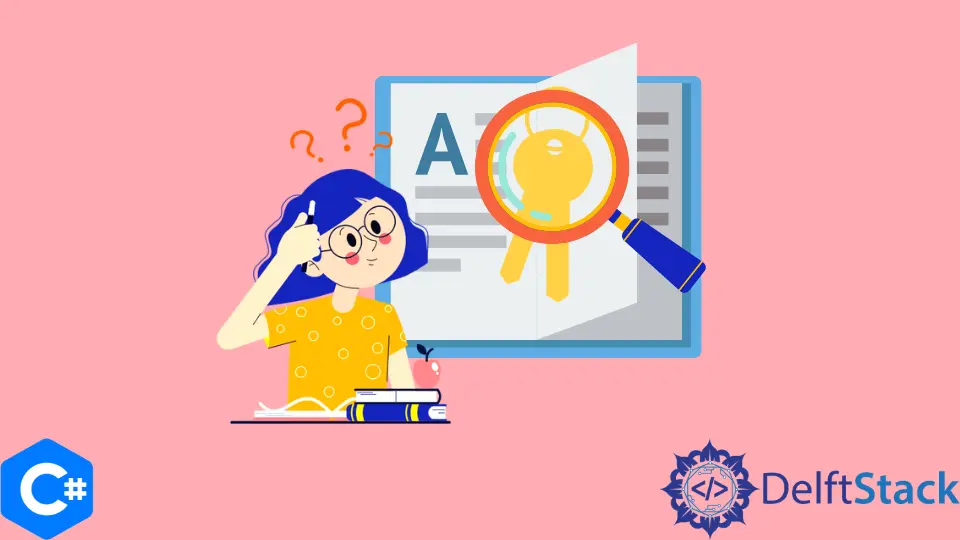
Dictionary
傾向於對映鍵和值。它包含特定值對映到的特定鍵。不允許有重複的鍵,這是字典
的全部目標。
今天我們將研究如何檢查一個鍵是否已經存在於一個字典
中。
在 C#
中使用 try-catch
檢查是否存在字典鍵
讓我們首先建立一個名為 students
的字典,將每個特定的學生 ID 對映到 CGPA:
Dictionary<int, double> students = new Dictionary<int, double>();
students[101] = 3.22;
students[102] = 3.44;
students[103] = 2.98;
因為 CGPA 應該是雙精度格式,所以我們將 ID 和 CGPA 分別定義為 <TKEY, TVALUE>
為 <int, double>
。
假設我們想在 students
中查詢學生 102
是否存在條目。確實如此,但我們需要一個程式碼來直接告訴我們。
為此,我們可以使用 try-catch
異常,如下所示:
try {
Console.WriteLine(students[102].ToString());
} catch (Exception e) {
Console.WriteLine("No such key present");
}
輸出:
3.44
但是如果我們現在嘗試對學生 104
做同樣的事情,會發生以下情況:
輸出:
No such key present
為什麼?好吧,ToString()
方法僅適用於非空值。因為學生 104
為 null 並且在 students
中沒有條目,所以會發生異常。
而不是丟擲異常,我們傾向於捕獲它並在控制檯中顯示訊息 No such key present
。
因此,這是檢查金鑰是否存在的有效方法。但是我們可以讓它更簡單嗎?
在 C#
中使用 ContainsKey()
檢查是否存在字典鍵
觀察以下程式碼:
if (!students.ContainsKey(104)) {
Console.WriteLine("No such key present");
}
這將出現錯誤,因為不存在這樣的鍵。
輸出:
No such key present
在 C#
中使用 TryGetValue()
檢查是否存在字典鍵
如果我們執行以下操作:
double getval = 0.0;
if (!students.TryGetValue(104, out getval)) {
Console.WriteLine("No such key present");
}
這往往會返回如下輸出:
No such key present
TryGetValue()
傾向於獲取鍵的值(如果存在)。該值在第二個引數中獲得。
因此,它使用 out
關鍵字傳送。獲取該值的變數在作用域之前宣告,即 getval
。
所有這些不同方法的完整程式碼已包含在下面:
using System;
using System.Collections.Generic;
using System.Text;
namespace jinlku_console {
class coder {
static void Main(String[] args) {
Dictionary<int, double> students = new Dictionary<int, double>();
students[101] = 3.22;
students[102] = 3.44;
students[103] = 2.98;
// TRY CATCH BLOCK
try {
Console.WriteLine(students[104].ToString());
} catch (Exception e) {
Console.WriteLine("No such key present");
}
// CONTAINS KEY METHOD
if (!students.ContainsKey(104)) {
Console.WriteLine("No such key present");
}
// TRYGETVALUE
double getval = 0.0;
if (!students.TryGetValue(104, out getval)) {
Console.WriteLine("No such key present");
}
}
}
}
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub