C# 中的字典與雜湊表
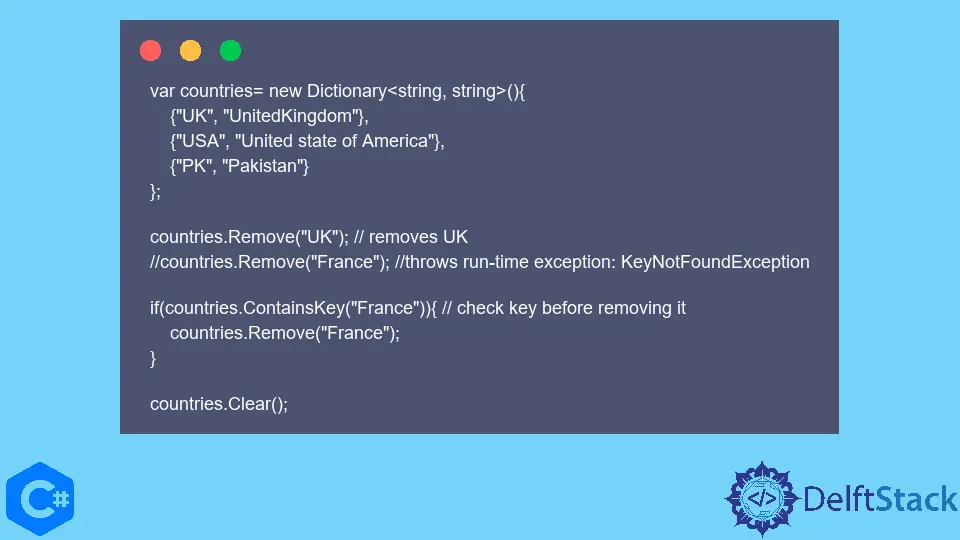
本指南將討論 C# 中 Dictionary
和 Hashtable
之間的區別。
要了解這些集合中哪個更好和更受歡迎,我們需要了解它們的基本原理。那麼,讓我們來看看與這兩個集合相關的語法、特徵、功能和方法。
在使用 C# 時,你應該更喜歡哪一個?哪個更好?
所有這些問題都將在下面得到解答。讓我們潛入吧!
C#
中的字典
Dictionary
是一個通用集合。它將資料儲存在鍵值對中,並且這個集合沒有特定的順序。
字典
的語法如下:
Dictionary<TKey, TValue>
我們將資料值連同它們的鍵一起傳遞。讓我們來看看字典
的特點。
C#
中字典的特徵
- 它儲存鍵值對。
- 它的名稱空間是
System.Collections.Generic
。 Dictionary
中的鍵不能為空並且應該是唯一的。- 但是,值可以為空值和重複值。
- 我們可以通過其對應的鍵訪問資料值,例如,
myDictionary[key]
。 - 所有元素都被認為是
KeyValuePair<TKey, TValue>
。
在 C#
中建立字典
你可以通過傳遞值的型別及其可以儲存的相應鍵在 C# 中建立一個字典
。檢視以下程式碼,瞭解我們如何建立 Dictionary
。
IDictionary<int, string> rollno_names = new Dictionary<int, string>();
rollno_names.Add(1, "Ali"); // adding a key/value using the Add() method
rollno_names.Add(2, "Haider");
rollno_names.Add(3, "Saad");
// The following throws runtime exception: key already added.
// rollno_names.Add(3, "Rafay");
foreach (KeyValuePair<int, string> i in rollno_names)
Console.WriteLine("Key: {0}, Value: {1}", i.Key, i.Value);
// creating a dictionary using collection-initializer syntax
var countries = new Dictionary<string, string>() {
{ "UK", "United Kingdom" }, { "USA", "United States of America" }, { "PK", "Pakistan" }
};
foreach (var j in countries) Console.WriteLine("Key: {0}, Value: {1}", j.Key, j.Value);
在上面的程式碼中,rollno_names
是一個 Dictionary
,其中 int 為鍵資料型別,字串為值資料型別,如下所示:
Dictionary<int, string>
這個特殊的 Dictionary
可以儲存 int 鍵和字串值。第二個字典
是由集合初始化器建立的國家/地區。
它的鍵和值都是字串資料型別。如果你嘗試複製鍵值並將其設定為 null,你將獲得執行時異常。
在 C#
中訪問字典元素
你可以在索引器的幫助下訪問 Dictionary
元素。你需要指定每個鍵來訪問其對應的值。
ElementAt()
是另一種獲取鍵值對的方法。看看下面的程式碼。
var countries = new Dictionary<string, string>() {
{ "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" }
};
Console.WriteLine(countries["UK"]); // prints value of UK key
Console.WriteLine(countries["USA"]); // prints value of USA key
// Console.WriteLine(countries["France"]); // runtime exception: key does not exist
// use ContainsKey() to check for the unknown key
if (countries.ContainsKey("France")) {
Console.WriteLine(countries["France"]);
}
// use TryGetValue() to get a value of the unknown key
string result;
if (countries.TryGetValue("France", out result)) {
Console.WriteLine(result);
}
// use ElementAt() to retrieve the key-value pair using the index
for (int i = 0; i < countries.Count; i++) {
Console.WriteLine("Key: {0}, Value: {1}", countries.ElementAt(i).Key,
countries.ElementAt(i).Value);
}
在上面的程式碼中,你可以看到沒有包含 France
的鍵值對。所以,上面的程式碼會給出一個執行時異常。
在 C#
中更新字典元素
通過在索引器中指定鍵,可以更改/更新鍵的值。如果在 Dictionary
中沒有找到鍵,它將丟擲 KeyNotFoundException
異常;因此,在訪問任何未知鍵之前使用 ContainsKey()
函式。
看看下面的程式碼。
var countries = new Dictionary<string, string>() { { "UK", "London, Manchester, Birmingham" },
{ "USA", "Chicago, New York, Washington" },
{ "PK", "Pakistan" } };
countries["UK"] = "Europe"; // update value of UK key
countries["USA"] = "America"; // update value of USA key
// countries["France"] = "Western Europe"; //throws run-time exception: KeyNotFoundException
if (countries.ContainsKey("France")) {
countries["France"] = "Western Europe";
}
在 C#
中刪除字典元素
使用 Remove()
方法刪除 Dictionary
中已經存在的鍵值對。使用 Clear()
方法刪除所有 Dictionary
元素。
var countries = new Dictionary<string, string>() {
{ "UK", "UnitedKingdom" }, { "USA", "United state of America" }, { "PK", "Pakistan" }
};
countries.Remove("UK"); // removes UK
// countries.Remove("France"); //throws run-time exception: KeyNotFoundException
if (countries.ContainsKey("France")) { // check key before removing it
countries.Remove("France");
}
countries.Clear();
C#
中的雜湊表
與 Dictionary
不同,Hashtable
是一個非泛型集合。它還儲存鍵值對。
通過計算每個鍵的雜湊碼並將其儲存在單獨的內部儲存桶中,它通過在訪問值時匹配所提供鍵的雜湊碼來改進查詢。看一下 Hashtable
的一些特徵。
C#
中 Hashtable 的特徵
- 鍵值對儲存在
Hashtables
中。 - 它屬於名稱空間
SystemCollection
。 - 實現了
IDictionary
介面。 - 鍵不能為空,並且必須是不同的。
- 值可能重複或為空。
- 可以通過向索引器提供相關鍵來獲取值,如
Hashtable[key]
。 DictionaryEntry
物件用於儲存元素。
在 C#
中建立一個雜湊表
檢視以下不言自明的程式碼以瞭解 Hashtable
的建立。
`Hashtable` rollno_names = new `Hashtable`();
rollno_names.Add(1, "Ali"); // adding a key/value using the Add() method
rollno_names.Add(2, "Haider");
rollno_names.Add(3, "Saad");
// The following throws runtime exception: key already added.
// rollno_names.Add(3, "Rafay");
foreach (DictionaryEntry i in rollno_names)
Console.WriteLine("Key: {0}, Value: {1}", i.Key, i.Value);
// creating a `Hashtable` using collection-initializer syntax
var countries = new `Hashtable`() {
{ "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" }
};
foreach (DictionaryEntry j in countries) Console.WriteLine("Key: {0}, Value: {1}", j.Key, j.Value);
我們可以像 Dictionary
一樣建立 Hashtable
。唯一的區別是它是非泛型的,因此我們不必指定鍵的資料型別及其對應的值。
在 C#
的雜湊表中新增字典
我們可以建立 Dictionary
的物件,並且通過在建立 Hashtable
時簡單地傳遞該物件,我們可以在 Hashtable
中新增 Dictionary
鍵值對。
更新 C#
中的雜湊表
通過在索引器中輸入鍵,你可以從 Hashtable
檢索現有鍵的值。由於 Hashtable
是一個非泛型集合(意味著鍵和值都沒有資料型別),因此在從中獲取值時需要將其型別轉換為字串。
看看下面的程式碼。
// creating a Hashtable using collection-initializer syntax
var countries = new Hashtable() {
{ { "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" } };
string countrynameUK = (string)countries["UK"]; // cast to string
string countrynameUSA = (string)countries["USA"]; // cast to string
Console.WriteLine(countrynameUK);
Console.WriteLine(countrynameUSA);
countries["UK"] = "Euorpe"; // update value of UK key
countries["USA"] = "America"; // update value of USA key
if (!countries.ContainsKey("France")) {
countries["France"] = "Western Euorpe";
}
從 C#
中的雜湊表中刪除元素
Hashtable
的給定鍵值對使用 Remove()
方法刪除。如果提供的鍵不存在於 Hashtable
中,則丟擲 KeyNotfoundException
異常;因此,在刪除金鑰之前,請使用 ContainsKey()
方法檢視它是否已經存在。
使用 Clear()
函式一次刪除每個專案。
var countries = new Hashtable() {
{ "UK", "UnitedKingdom" }, { "USA", "United State of America" }, { "PK", "Pakistan" }
};
countries.Remove("UK"); // removes UK
// countries.Remove("France"); //throws run-time exception: KeyNotFoundException
if (countries.ContainsKey("France")) { // check key before removing it
countries.Remove("France");
}
countries.Clear(); // removes all elements
C#
中的字典與雜湊表
Dictionary |
Hashtable |
---|---|
如果我們試圖發現一個不存在的鍵,它要麼返回要麼丟擲異常。 | 如果我們試圖發現一個不存在的鍵,它會返回 null。 |
因為沒有裝箱和拆箱,它比 Hashtable 快。 |
由於需要裝箱和拆箱,它需要比字典 更長的時間。 |
執行緒安全僅適用於公共靜態成員。 | Hashtable 的成員都是執行緒安全的, |
由於 Dictionary 是泛型型別,任何資料型別都可以與它一起使用(建立它時,我們必須指定鍵和值的資料型別)。 |
不是泛型型別。 |
具有強型別鍵和值的 Hashtable 實現稱為 Dictionary 。 |
由於 Hashtables 是靈活型別的資料結構,因此可以新增任何鍵和值型別。 |
基於這些事實,我們可以說 Dictionary
優於 Hashtable
。因為你可以將任何隨機項插入 Dictionary<TKey, TValue>
並且你不必強制轉換取出的值,所以你可以獲得型別安全。
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn