How to Convert Char Array to Strings in C#
-
Use the
string()
Method to Convert Char Array to String inC#
-
Use the
string.Join()
Method to Convert Char Array to String inC#
-
Use the
string.Concat()
Method to Convert Char Array to String inC#
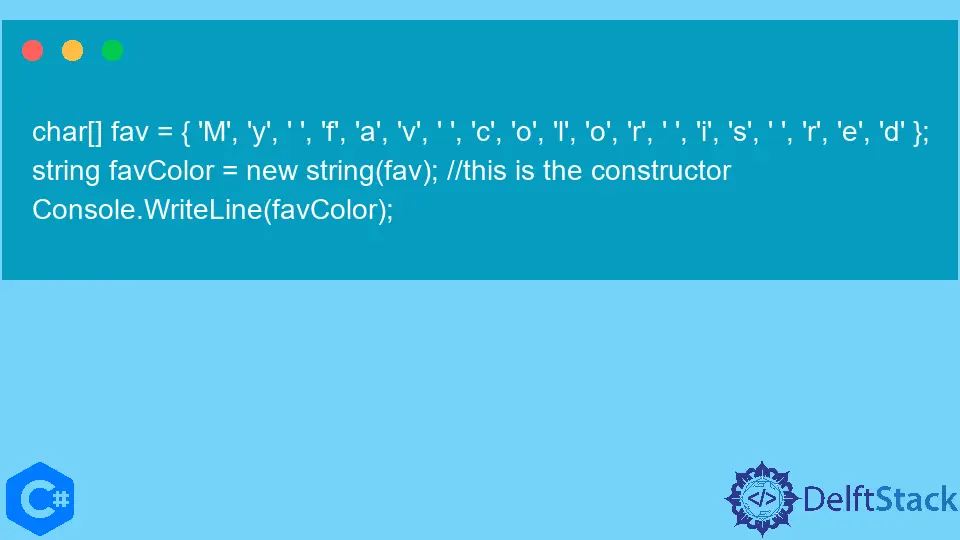
This guide will discuss how to convert a char array to a string in C#.
The values printed in character array
will be as a single character, and there are three ways to make them a string
.
The following are the ways to convert the char array to string in C#
Use the string()
Method to Convert Char Array to String in C#
The string constructor is the first method you can use to convert a char array to a string in C#. The string()
is a class constructor that combines the characters to form a string. It uses a character array as its parameter.
Code:
char[] fav = { 'M', 'y', ' ', 'f', 'a', 'v', ' ', 'c', 'o', 'l',
'o', 'r', ' ', 'i', 's', ' ', 'r', 'e', 'd' };
string favColor = new string(fav); // this is the constructor
Console.WriteLine(favColor);
Output:
My fav color is red
This is one of C #’s most common methods to convert char array to string.
Use the string.Join()
Method to Convert Char Array to String in C#
The string.Join()
is used to join the characters in a string formation. It needs two values as its parameter.
The first is a separator, which uses an empty string
or space. The char
array is used as the second parameter.
Code:
char[] fav = { 'M', 'y', ' ', 'f', 'a', 'v', ' ', 'c', 'o', 'l',
'o', 'r', ' ', 'i', 's', ' ', 'r', 'e', 'd' };
string favColor = string.Join(" ", fav); // this is the use of string.join()
Console.WriteLine(favColor);
Output:
M y f a v c o l o r i s r e d
This method uses a stringBuilder()
class internally, which is more efficient than the other methods.
Use the string.Concat()
Method to Convert Char Array to String in C#
The string.Concat()
method is a combination of the above two methods. Even though it’s a combination, this method is still different from the other two methods mentioned as it needs only one parameter, and the second method, string.Join()
, has stringBuilder()
, but this method works like a string constructor.
Also, this method concats the characters into a string instead of joining them.
Code:
char[] fav = { 'M', 'y', ' ', 'f', 'a', 'v', ' ', 'c', 'o', 'l',
'o', 'r', ' ', 'i', 's', ' ', 'r', 'e', 'd' };
string favColor = string.Concat(fav); // this is the use of string.concat()
Console.WriteLine(favColor);
Output:
My fav color is red
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Csharp Array
- How to Get the Length of an Array in C#
- How to Sort an Array in C#
- How to Sort an Array in Descending Order in C#
- How to Remove Element of an Array in C#
- How to Convert a String to a Byte Array in C#
- How to Adding Values to a C# Array