How to Read String in Arduino Serial Port
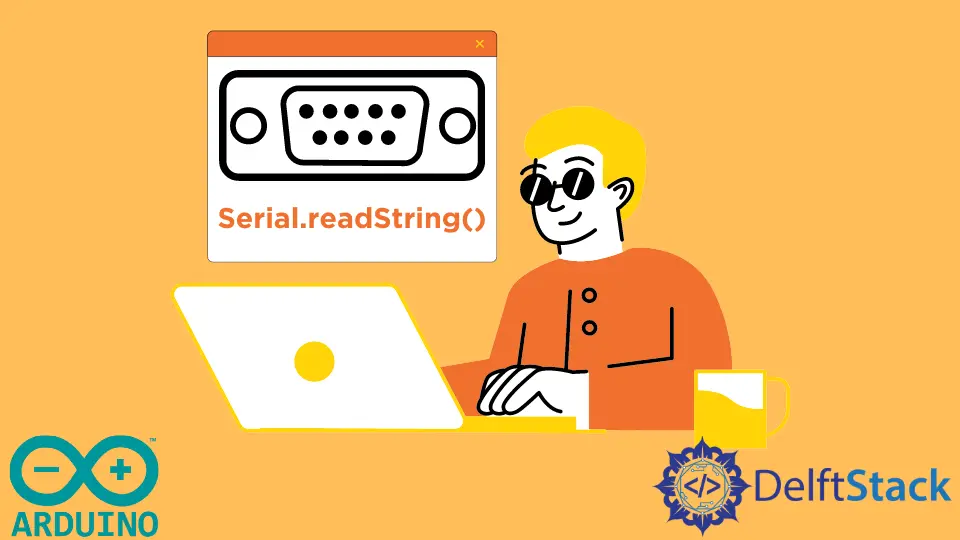
Reading strings from the Arduino serial port is an essential skill for anyone delving into the world of Arduino programming. Whether you’re building a simple project or a complex application, being able to read data from the serial port can open up a plethora of possibilities.
In this article, we’ll explore two primary methods for reading strings in Arduino: using the Serial.readString()
function and the Serial.readStringUntil()
function. These functions are straightforward yet powerful, allowing you to capture user input or data from sensors effectively. Let’s dive into how to implement these methods for reading strings from the Arduino serial port.
Using Serial.readString()
The Serial.readString()
function is a convenient way to read a string from the serial buffer until a timeout occurs. It captures all incoming characters and returns them as a single string. This method is particularly useful when you want to read a complete line of text or a series of characters without worrying about delimiters.
Here’s a simple example that demonstrates how to use Serial.readString()
:
void setup() {
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
String inputString = Serial.readString();
Serial.print("You entered: ");
Serial.println(inputString);
}
}
In this code, we start by initializing the serial communication at a baud rate of 9600. The loop()
function continuously checks if there are any bytes available to read. If there are, it reads the entire string until a timeout occurs, storing it in the inputString
variable. Finally, it prints the received string back to the serial monitor.
Output:
You entered: Hello Arduino
The Serial.readString()
function is ideal for situations where you expect a complete message without needing to specify a delimiter. However, keep in mind that it waits for a timeout, which can sometimes lead to delays in your program if the input is not received quickly. This method is perfect for reading user inputs, such as commands or configuration settings.
Using Serial.readStringUntil()
The Serial.readStringUntil()
function offers a more tailored approach to reading strings from the serial port. With this method, you can specify a delimiter that marks the end of the string. This is particularly useful when you want to read data that is formatted with specific characters, such as commas or line breaks.
Here’s how to use Serial.readStringUntil()
:
void setup() {
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
String inputString = Serial.readStringUntil('\n');
Serial.print("You entered: ");
Serial.println(inputString);
}
}
In this example, we again initialize serial communication at a baud rate of 9600. The loop()
function checks for available bytes and reads the string until it encounters a newline character (\n
). This means that the string is captured up to the point where the user presses the Enter key. The received string is then printed back to the serial monitor.
Output:
You entered: Hello Arduino
Using Serial.readStringUntil()
is beneficial when you are reading structured data where a specific end character is used. This method helps eliminate unwanted characters and allows for cleaner data processing. It’s especially handy in applications where you need to parse data from sensors or other devices that send information in a specific format.
Conclusion
Reading strings from the Arduino serial port using Serial.readString()
and Serial.readStringUntil()
is a fundamental skill that can enhance your projects significantly. Each method has its advantages, depending on your specific needs. Whether you choose to read a complete string until a timeout or capture data until a specific delimiter, these functions provide a robust way to handle serial communication. Experiment with both methods to determine which one best suits your application, and you’ll find that working with Arduino becomes even more enjoyable and productive.
FAQ
-
What is the difference between Serial.readString() and Serial.readStringUntil()?
Serial.readString() reads until a timeout, while Serial.readStringUntil() reads until a specified delimiter. -
Can I use other delimiters with Serial.readStringUntil()?
Yes, you can specify any character as a delimiter, such as commas or semicolons. -
What baud rate should I use for serial communication?
A common baud rate is 9600, but you can choose higher rates for faster communication if both sender and receiver support it.
-
How can I clear the serial buffer?
You can clear the serial buffer using the Serial.flush() function, which waits for the transmission of outgoing serial data to complete. -
Is there a limit to the length of the string I can read?
Yes, the maximum length of the string is limited by the available memory on your Arduino board.
Related Article - Arduino String
- Arduino strcmp Function
- Arduino Strcpy Function
- How to Concatenate Strings in Arduino
- How to Parse a String in Arduino
- How to Split String in Arduino
- How to Compare Strings in Arduino