How to Concatenate Strings in Arduino
- Strings in Arduino
-
Concatenate Strings in Arduino Using the
concat()
Function -
Concatenate Strings in Arduino Using the Append Operator (
+
) -
Concatenate Strings in Arduino Using
c_str()
and the Append Operator (+
) - Conclusion
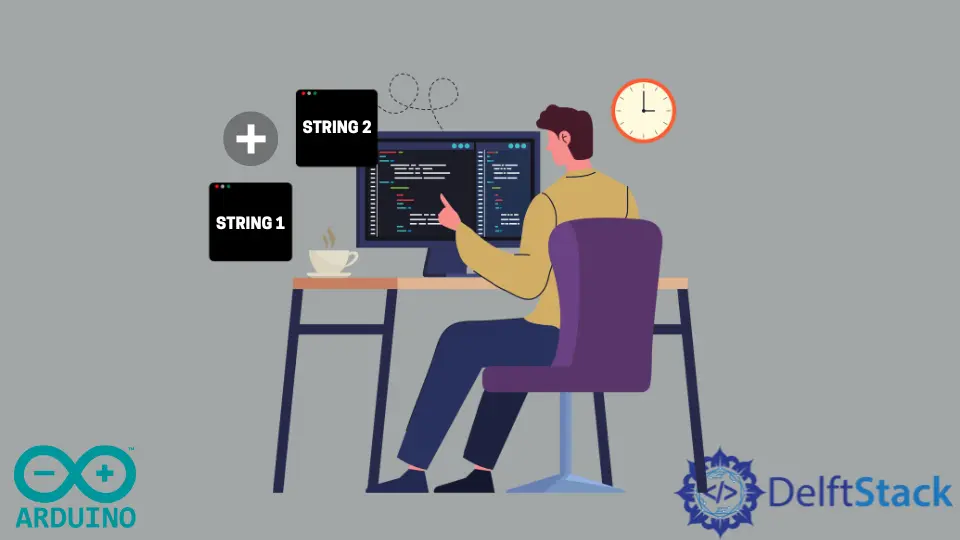
Arduino, the open-source electronics platform, is widely used for creating interactive projects and prototypes. When working with Arduino, manipulating strings is a common requirement, and concatenating strings is a fundamental operation.
In this article, we’ll explore the basics of string concatenation in Arduino and provide examples to help you integrate this essential skill into your projects.
Strings in Arduino
In Arduino programming, strings are sequences of characters. Each character in a string is represented by its ASCII value, and strings are terminated with a null character ('\0'
). The String
class is used to manipulate strings conveniently.
To concatenate strings means to combine two or more strings into a single string. This can be particularly useful when you want to create custom messages, display information, or communicate with external devices.
Concatenate Strings in Arduino Using the concat()
Function
The concat()
function provides a convenient way to append one string to another in Arduino. This method is especially useful when you want to build a string gradually, adding different components one at a time.
Its basic syntax is as follows:
MyString.concat(MyParameter);
Here, MyString
is a string
object containing the initial string, and MyParameter
is the string or value to be appended. The concat()
function modifies the original string, and its return value is true
if the concatenation is successful and false
otherwise.
Let’s now dive into a practical example demonstrating the usage of the concat()
function:
void setup() {
String s1 = "Hello";
String s2 = " Arduino";
// Concatenate s2 to s1 using the concat() function
s1.concat(s2);
Serial.begin(9600);
Serial.println(s1);
}
void loop() {}
In this example, we start by declaring two string variables, s1
and s2
, initialized with the strings Hello
and Arduino
, respectively. The concat()
function is then used on s1
to append the content of s2
to it.
This modified string is then printed to the serial monitor using Serial.println()
.
The setup()
function is where the concatenation takes place, and the loop()
function remains empty, as we do not need any continuous execution for this particular example.
Output:
Hello Arduino
This output showcases the successful concatenation of the two strings.
Concatenate Strings in Arduino Using the Append Operator (+
)
Concatenating strings in Arduino can also be achieved using the append operator +
. This is the most straightforward method to concatenate strings in Arduino.
The append operator +
in Arduino allows for the concatenation of strings or variables of various data types. The basic syntax is as follows:
MyString = Parameter1 + Parameter2 + ... + ParameterN;
Here, MyString
is a string
object used to store the resulting concatenation, and Parameter1
through ParameterN
represents the values or strings to be appended. The append operator automatically converts different data types to strings before concatenating them.
Let’s explore a practical example that demonstrates the application of the append operator +
:
void setup() {
String s1 = "Hello";
String s2 = " Arduino";
int n = 10;
// Concatenate strings and an integer using the append operator
String s3 = s1 + " ,,," + s2 + " " + n;
Serial.begin(9600);
Serial.println(s3);
}
void loop() {}
In this example, we start by declaring two string variables, s1
and s2
, initialized with the strings Hello
and Arduino
, respectively. Additionally, an integer variable n
is declared and assigned the value 10.
The append operator +
is then employed to concatenate these variables and strings into a new string s3
.
The setup()
function handles the concatenation and serial output, while the loop()
function remains empty since we do not require continuous execution.
Output:
Hello ,,, Arduino 10
This output illustrates the successful concatenation of strings and an integer using the append operator +
.
Concatenate Strings in Arduino Using c_str()
and the Append Operator (+
)
In certain scenarios, you may encounter the need to concatenate strings in Arduino while also incorporating C-style strings (char
arrays). This advanced approach involves using the c_str()
function to convert String
objects to C-style strings, allowing for seamless integration with the append operator +
.
To concatenate strings using c_str()
and the append operator, you first convert the String
objects to C-style strings using c_str()
, then utilize the append operator to concatenate them.
The basic syntax is as follows:
MyString = String(Parameter1.c_str()) + String(Parameter2.c_str()) + ... +
String(ParameterN.c_str());
Here, MyString
is the String
object used to store the result, and Parameter1
, Parameter2
, and so forth are the original String
objects to be converted to C-style strings.
Here’s an example demonstrating the usage of c_str()
with the append operator (+
):
void setup() {
String s1 = "Hello";
String s2 = " Arduino";
// Convert the String objects to C-style strings and concatenate them
String result = String(s1.c_str()) + String(s2.c_str());
Serial.begin(9600);
Serial.println(result);
}
void loop() {}
In this example, we start by declaring two String variables, s1
and s2
, initialized with the same strings Hello
and Arduino
. The c_str()
function is then applied to each String
object, converting them into C-style strings.
The append operator is subsequently used to concatenate these C-style strings, and the result is stored in the variable result
. Finally, the concatenated string is printed to the serial monitor using Serial.println()
.
Output:
Hello Arduino
This output illustrates the successful concatenation of strings using the c_str()
function and the append operator in Arduino.
When working with strings in Arduino, it’s essential to be mindful of memory usage. String manipulation can lead to memory fragmentation and, in some cases, program crashes.
Avoid excessive use of dynamic memory allocation and consider using character arrays (char[]
) for more memory-efficient string operations, especially in resource-constrained environments.
Conclusion
Concatenating strings in Arduino is a fundamental skill that allows you to create dynamic and informative messages for your projects. Whether you’re displaying data on an LCD screen or communicating with external devices, mastering string concatenation will enhance your ability to create versatile and engaging Arduino applications.
Experiment with the examples provided in this article, and incorporate this knowledge into your future projects to unlock the full potential of the Arduino platform.