How to Parse a String in Arduino
-
Parse a String Using the
strtok()
Function in Arduino -
Parse a String Using the
substring()
Function in Arduino
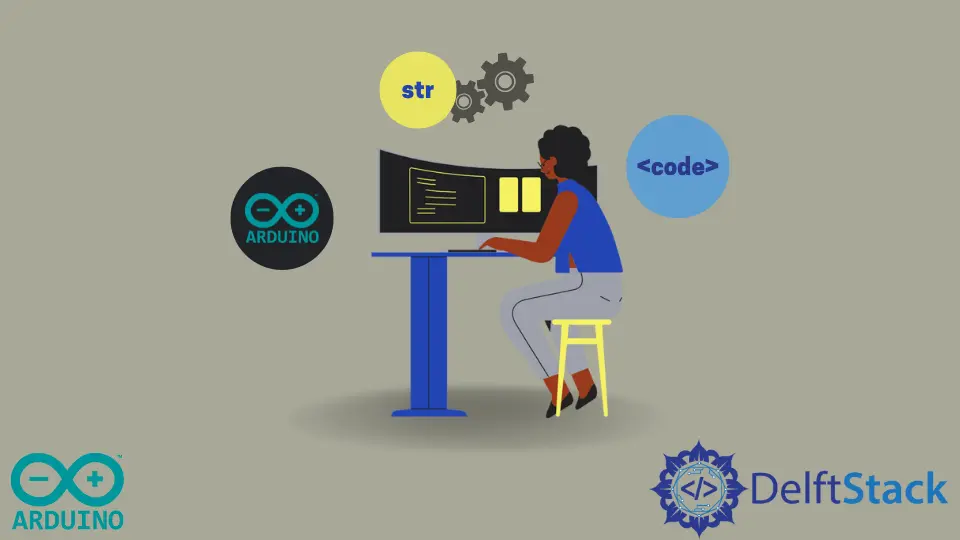
This tutorial will discuss parsing a string using the strtok()
and substring()
functions in Arduino.
Parse a String Using the strtok()
Function in Arduino
A string can contain characters, numbers, and symbols in Arduino. We can use the strtok()
function in Arduino to separate or parse a string.
For example, if we have a string with sub-strings separated by a comma, we want to separate each sub-string using the comma and save each sub-string as a separate string or character array.
Syntax:
char *s = strtok(char *MyS, char *MyD);
In the above code, MyS
is a constant char variable that contains the string that we want to parse, and MyD
is also a constant char variable that contains the delimiter, character, or symbol that we what to use to parse the given string. The strtok()
function will return the first token or the array of characters before the given delimiter.
We have to pass NULL
in place of the given string to get the second string token because the given string excluding the first token will be saved in the strtok()
function.
For example, let’s define a character string and separate it using the delimiter and the strtok()
function.
char *MyS = "this is-delftstack.com-website";
char *MyD = "-";
void setup() {
Serial.begin(9600);
char *s = strtok(MyS, MyD);
Serial.println(s);
char *s1 = strtok(NULL, MyD);
Serial.println(s1);
char *s2 = strtok(NULL, MyD);
Serial.println(s2);
}
void loop() {}
Output:
this is
delftstack.com
website
In the above code, we used the strtok()
function three times to get all the three tokens present in the given string, and each string token will be stored inside a character array. We can also use a loop to get all the available tokens present in the given string so that we don’t have to write the code repeatedly.
We wrote the code inside the setup()
function, which will run only once when we power the Arduino board, but in case of a large string, we can write the code inside the loop()
function to repeat itself. We will get the first token inside the setup()
function, and we will check if the next token is available inside the loop()
function.
If available, we will store it inside an array and repeat the procedure until no more string tokens are left inside the given string.
For example, let’s use the above string and find all the available string tokens using the same delimiter above.
char *MyS = "this is-delftstack.com-website-Welcome";
char *MyD = "-";
char *MyArray[10];
int i = 1;
void setup() {
Serial.begin(9600);
char *s = strtok(MyS, MyD);
Serial.println(s);
MyArray[0] = s;
}
void loop() {
char *s1 = strtok(NULL, MyD);
if (s1 != NULL) {
MyArray[i] = s1;
Serial.println(MyArray[i]);
i = i + 1;
}
}
Output:
this is
delftstack.com
website
Welcome
In the code above, we initialized the array with size 10
, which will be used to store the string tokens. We can initialize it according to the string tokens present in the given string.
We also initialized the index, which will be used to store values inside the array. We will increment it inside the loop()
function.
We used an if
statement to check if the string token is NULL or not. If it’s not NULL, we will store it inside the array and increment the index.
Note that the loop has to repeat to find the next string token. We can place a loop inside the setup()
function if we want to get all the tokens before the loop()
function.
We will have all the string tokens stored inside the array before moving to the loop()
function. The delimiter can be any character, symbol, or number present in the given string, and we can also use space as a delimiter.
Parse a String Using the substring()
Function in Arduino
We can also use the substring()
function in Arduino to separate or parse a string. According to the given index values, the substring()
separates a sub-string from the given string.
For example, if we want to get the first five characters from a string, we can use the substring()
function and the index value 5.
Syntax:
String s = MyS.substring(SIndex, EIndex);
In the above code, s
is the string variable used to store the output of the substring()
function and MyS
is the given string with a string data type. The SIndex
is the starting index, and EIndex
is the ending index for the sub-string.
For example, let’s create a string and find its sub-string in the 0
to 5
index.
String MyS = "this is delftstack.com website Welcome";
void setup() {
Serial.begin(9600);
String s = MyS.substring(0, 5);
Serial.println(s);
}
void loop() {}
Output:
this
In the above code, we used the serial monitor of Arduino to display the result. As you can see from the output, the first five characters or indexes are displayed on the serial monitor window.
We can only see four characters in the output because the fifth character is the space character. If we don’t know the index of a character, we can use the indexOf()
function to get the index of that character.
Syntax of the indexOf()
function:
int MyI = MyS.indexOf(char);
In the above code, the MyI
variable will store the output, which is the index of the given character, and char
is the character whose index we want to find. The MyS
is the string variable that contains the given string.
For example, we have a string of words separated by a space, and we want to get the first word from the string. We can use the indexOf()
function to find the index of the space character and then pass that index inside the substring()
function to get the first word.
String MyS = "this is delftstack.com website Welcome";
void setup() {
Serial.begin(9600);
int MyI = MyS.indexOf(" ");
String s = MyS.substring(0, MyI);
Serial.println(s);
}
void loop() {}
Output:
this
As you can see in the above output, we extracted the first word from the given string. If we want to get all the world from the given string, we must use a loop.
We can also pass a second argument inside the indexOf()
function to set the starting position, which will be used to search for the index of the given character.
For example, in the above example, if we want to get the first two words of the string, we can set the starting index value to 5
, and the function will return the index value of the second delimiter, which we can use to extract the first two words.
We wrote the code inside the setup()
function in the above code, which will only run once. But if we wrote the code inside the loop()
function, the code will repeat itself.
We can write the code inside the loop()
function to find all the sub-strings present in the given string.
For example, let’s find all the substrings present in the above string.
String MyS = "this is delftstack.com website Welcome";
int MyP = 0;
int MyI = 0;
String array[10];
int index = 0;
void setup() { Serial.begin(9600); }
void loop() {
if (MyI >= 0) {
MyI = MyS.indexOf(" ", MyP);
String s = MyS.substring(MyP, MyI);
Serial.println(s);
MyP = MyI + 1;
array[index] = s;
Serial.println(array[index]);
index = index + 1;
}
}
Output:
this
is
delftstack.com
website
Welcome
In the above code, we used the index value of the given delimiter inside the if
statement to check if the end of the given string is reached because the indexOf()
function will return a negative value if the index that we passed inside the indexOf()
function exceeds the length of the given string.
We have initialized two integer variables, MyP
and MyI
, which will be used for previous and current index values.
We will use the MyP
or previous index value to get the MyI
or next index value, and then we will use these values to find the substring. After that, we will change the value of the MyP
or previous index to the current index plus one so that the next time the loop repeats itself; we will get the next word.
We have also initialized a string array of size 10 to store the substrings according to the index value. We will increment the index in the if
statement to save the next substring in the next index value.
Note that the loop must repeat itself to get all the substrings present in the given string. We can employ a loop inside the setup ()
function if we want to get the substrings before starting the loop()
function.
We used space as a delimiter in the above examples, but we can use any character, symbol, and number to parse the given string. The indexOf()
and substring()
functions belong to the string class of Arduino and for more details about the string class, check this link.