How to Compare Strings in Arduino
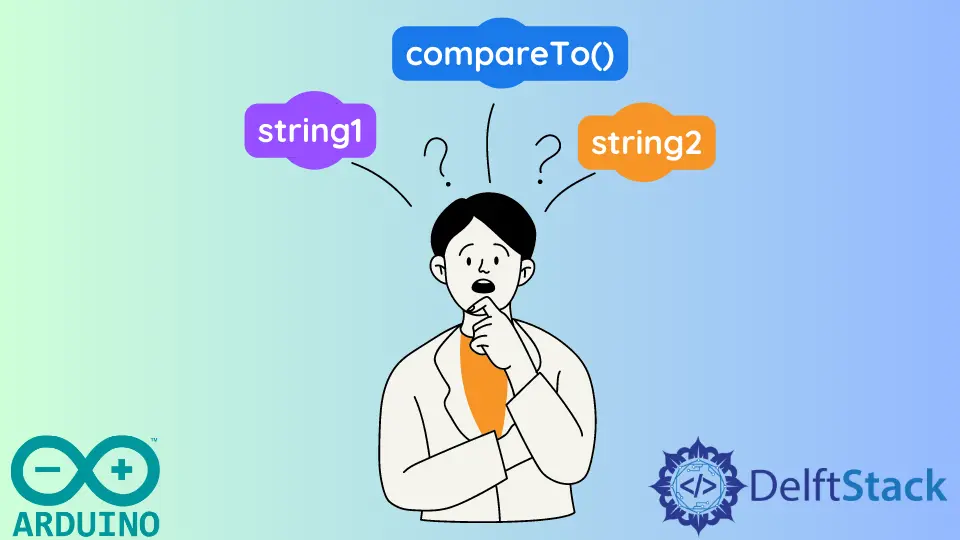
When working with Arduino, string manipulation is a fundamental skill that can significantly enhance your programming capabilities. One common task is comparing two strings, which can be essential for various applications, such as validating user input or controlling the flow of your program based on string values. In Arduino, the compareTo()
function is a handy tool for this purpose.
This article will guide you through the process of comparing strings in Arduino, detailing how to use the compareTo()
function effectively. By the end, you’ll have a solid understanding of string comparison and its practical applications in your Arduino projects.
Understanding the compareTo()
Function
The compareTo()
function in Arduino is a method of the String
class that allows you to compare two strings lexicographically. This means that it compares the strings character by character based on their ASCII values. The function returns an integer: a negative value if the first string is less than the second, zero if they are equal, and a positive value if the first string is greater than the second. This straightforward comparison method is essential for decision-making processes in your code.
Basic Usage of compareTo()
To use the compareTo()
function, you first need to create two string variables. Here’s a simple example to illustrate how this works:
void setup() {
Serial.begin(9600);
String str1 = "Hello";
String str2 = "World";
int result = str1.compareTo(str2);
Serial.println(result);
}
void loop() {
}
Output:
-15
In this example, we initialize two strings, str1
and str2
. We then call compareTo()
on str1
, passing str2
as an argument. The result is a negative number, indicating that “Hello” is lexicographically less than “World”. This method is particularly useful when you want to sort strings or check for specific conditions in your code.
Comparing Strings for Equality
Often, you may want to check if two strings are equal. While compareTo()
can do this, using the equality operator ==
is a more straightforward approach for equality checks. Here’s how you can do it:
void setup() {
Serial.begin(9600);
String str1 = "Arduino";
String str2 = "Arduino";
if (str1 == str2) {
Serial.println("Strings are equal");
} else {
Serial.println("Strings are not equal");
}
}
void loop() {
}
Output:
Strings are equal
In this case, we check if str1
is equal to str2
using the ==
operator. Since both strings contain the same value, the output confirms their equality. This method is quick and efficient for equality checks, while compareTo()
is better suited for determining the relative order of strings.
Case Sensitivity in String Comparisons
Another important aspect of string comparison is case sensitivity. The compareTo()
function is case-sensitive, meaning that “hello” and “Hello” are considered different strings. If you want to compare strings without regard to case, you can convert both strings to the same case before comparison. Here’s an example:
void setup() {
Serial.begin(9600);
String str1 = "Hello";
String str2 = "hello";
if (str1.equalsIgnoreCase(str2)) {
Serial.println("Strings are equal (case insensitive)");
} else {
Serial.println("Strings are not equal");
}
}
void loop() {
}
Output:
Strings are not equal
In this example, we use the equalsIgnoreCase()
method to compare str1
and str2
. Since the method ignores case differences, it would return true if both strings were the same except for case. This is particularly useful when dealing with user input, where case variations are common.
Conclusion
Comparing strings in Arduino is a fundamental skill that can greatly enhance your programming projects. The compareTo()
function provides a straightforward way to determine the lexicographic order of strings, while the ==
operator is perfect for checking equality. Additionally, understanding case sensitivity and utilizing methods like equalsIgnoreCase()
can help you handle user input more effectively. With these tools at your disposal, you’ll be well-equipped to implement string comparisons in your Arduino applications.
FAQ
-
How does the
compareTo()
function work in Arduino?
ThecompareTo()
function compares two strings lexicographically and returns an integer indicating their relative order. -
Can I use compareTo() for case-insensitive comparisons?
No, compareTo() is case-sensitive. To compare strings without case sensitivity, use equalsIgnoreCase(). -
What does a negative return value from compareTo() indicate?
A negative return value indicates that the first string is lexicographically less than the second string. -
Is there a simpler way to check for string equality in Arduino?
Yes, you can use the equality operator (==) for a straightforward equality check. -
How can I handle user input with string comparisons?
You can use methods like equalsIgnoreCase() to compare user input while ignoring case differences.