How to Split String in Arduino
-
Use the
substring()
Function to Split a String in Arduino -
Use the
indexOf()
Function for Dynamic Splitting in Arduino - Split String Based on Newlines in Arduino
-
Use the C Function
strtok()
to Split a String in Arduino - Use Manual String Parsing to Split a String in Arduino
- Conclusion
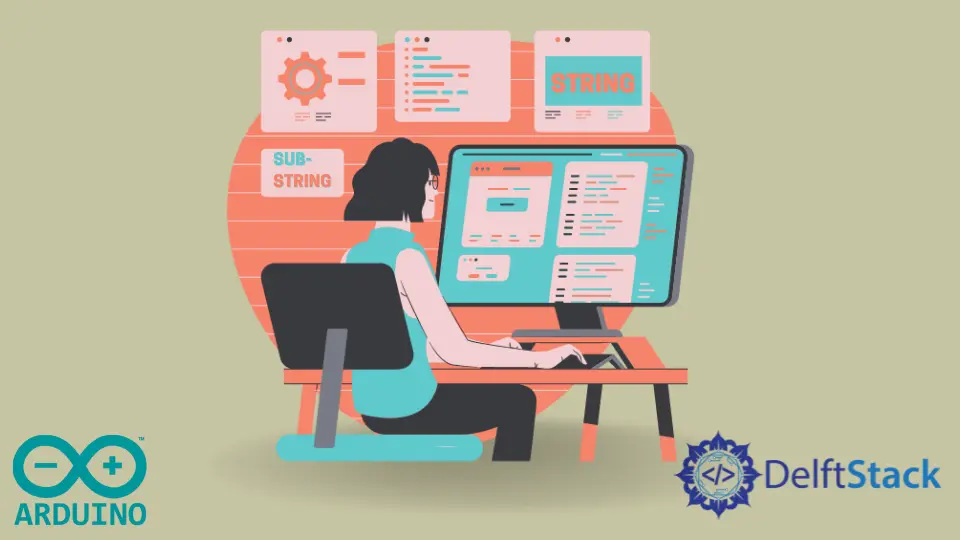
In Arduino programming, effective string manipulation is a foundational skill, and the ability to split strings into substrings is particularly crucial for a range of applications—from handling sensor data and communication protocols to managing user inputs. Recognizing this significance, this article delves into the intricacies of string manipulation in Arduino, offering a detailed exploration of diverse methods to split strings.
Whether you are a beginner or an experienced Arduino developer, understanding these techniques is essential. The article covers various approaches, including the built-in substring()
function for index-based extraction, dynamic splitting with indexOf()
, newline character-based splitting, utilizing the C standard library’s strtok()
function, and manual string parsing for nuanced control.
Each method is accompanied by clear explanations and illustrative example codes, providing readers with a comprehensive guide to effectively split strings in Arduino projects.
Use the substring()
Function to Split a String in Arduino
The substring()
function, a built-in feature of Arduino, provides an effective way to split strings. It allows you to extract specific portions of a string based on defined start and end indices.
The substring()
function has two arguments. The first argument is the starting index value from which we want to start the splitting process, and the second is the ending index value where the splitting process will stop.
Syntax:
String substring(int beginIndex, int endIndex);
Parameters:
beginIndex
: The given index from which the substring extraction begins.endIndex
: The index up to which the substring is extracted but not included.
Now, let’s delve into the details of how to use the substring()
function effectively for string splitting.
Let’s begin with a basic example to demonstrate the fundamental usage of the substring()
function:
String originalString = "Hello,World";
int startIndex = 0;
int length = 5;
void setup() {
Serial.begin(9600);
String substring = originalString.substring(startIndex, startIndex + length);
Serial.println(substring);
}
void loop() {
// Your main code here
}
Output:
This code initializes a string "Hello,World"
and defines global variables for the starting index and length of a substring. The setup()
function utilizes the substring()
function to extract a substring from the original string based on the specified start index and length.
The extracted substring, "Hello"
, is then printed to the Serial Monitor. The loop()
function is empty as it typically contains the main code for continuous execution in an Arduino sketch.
Overall, the code demonstrates the basic usage of the substring()
function for extracting specific portions of a string in Arduino.
Use the indexOf()
Function for Dynamic Splitting in Arduino
In scenarios where you need to split a string based on a specific character (e.g., a space or a comma) with an unknown index, the indexOf()
function comes to the rescue. This function dynamically finds the index of the desired character within the string.
By default, the indexOf()
function starts searching the string from the start to find the index of the given character, but we can also pass an index as a starting point using the second argument of the indexOf()
function.
Syntax:
int index = MyString.indexOf(val, from);
The index
local variable will store the val
variable index, which contains a character or string in the above code. The from
variable defines the starting index used as the starting point to find the index of the given character.
The indexOf()
function will return -1
if the index is not found in the given string.
Let’s begin with a basic example to illustrate the primary usage of the indexOf()
function:
String originalString = "Hello,World";
char searchChar = ',';
void setup() {
Serial.begin(9600);
int indexOfChar = originalString.indexOf(searchChar);
Serial.println("Index of ',': " + String(indexOfChar));
}
void loop() {
// Your main code here
}
Output:
In this example, the indexOf()
function is used to find the index of the first occurrence of ','
in "Hello,World"
. The result, which is the index (in this case, 5), is printed to the Serial Monitor.
Split String Based on Newlines in Arduino
Splitting a string based on newline characters ('\n'
) is a common requirement, especially when dealing with multiline text data. We can achieve this using the indexOf()
function to locate newline character indices.
Example Code:
String My_S = "hello world\nGreetings\nPeople";
void setup() {
Serial.begin(9600);
Serial.println(My_S);
int index = My_S.lastIndexOf('\n');
int length = My_S.length();
String sub_S = My_S.substring(index, length);
Serial.println(sub_S);
}
void loop() {
// Your main code here
}
Output:
This code initializes a String
variable My_S
with the value "hello world\nGreetings\nPeople"
. In the setup
function, it prints the entire content of the string data to the Serial Monitor.
Subsequently, it utilizes the lastIndexOf()
function to find the index of the last newline character ('\n'
) in the string.
It then determines the length of the string using the length()
function. Afterward, the substring()
function extracts a substring from the original string, starting from the last newline character to the end.
This substring is printed to the Serial Monitor as well. The loop
function is left empty, implying that the operations in the setup function are executed only once, ensuring that the output is not repeated in a continuous loop.
Use the C Function strtok()
to Split a String in Arduino
The strtok()
function is a C standard library function used to break a string into a sequence of tokens. It takes two arguments: the input string to be tokenized and a delimiter character that specifies where the string should be split.
The function returns a pointer to the next token in the input string until no more tokens are found, at which point it returns NULL
.
Syntax:
char *strtok(char *str, const char *delim);
Parameters:
-
str
is the string input to tokenize. For subsequent calls, this argument should be set toNULL
to continue tokenizing the same string. -
delim
is a string containing one or more delimiter characters, and the function will split the string input wherever it finds any of these delimiter characters.
Let’s begin with a basic example to illustrate the fundamental usage of the strtok()
function in an Arduino sketch:
char originalString[] = "Alice,Bob,Charlie,David";
char delimiters[] = ",";
void setup() {
Serial.begin(9600);
// Tokenize the original string
char *token = strtok(originalString, delimiters);
// Print each token
while (token != NULL) {
Serial.println(token);
token = strtok(NULL, delimiters);
}
}
void loop() {
// Your main code here
}
Output:
This code tokenizes and prints substrings from the original string "Alice,Bob,Charlie,David"
using a comma (','
) as the char
separator. It employs the strtok()
function to find and print each token sequentially in the Serial Monitor within the setup
function.
The loop
function is left empty, ensuring that the tokenization and printing process occurs only once.
Dynamic Delimiter and Tokenization
Let’s explore a more dynamic example where the delimiters and the string to be tokenized are dynamically determined:
char originalString[] = "Sensor1:Value1|Sensor2:Value2|Sensor3:Value3";
char delimiters[] = ":|";
void setup() {
Serial.begin(9600);
// Tokenize the original string
char *token = strtok(originalString, delimiters);
// Print each token
while (token != NULL) {
Serial.println(token);
token = strtok(NULL, delimiters);
}
}
void loop() {
// Your main code here
}
Output:
This code tokenizes and prints substrings from the original string "Sensor1:Value1|Sensor2:Value2|Sensor3:Value3"
using two delimiters, colon ':'
and pipe '|'
. The strtok()
function is employed in the setup
function to find and print each token sequentially in the Serial Monitor.
The loop
function is left empty, ensuring that the tokenization and printing process occurs only once during the setup, displaying the extracted substrings on separate lines in the Serial Monitor.
Use Manual String Parsing to Split a String in Arduino
Manual string parsing allows you to have fine-grained control over the process of extracting substrings from a given string. It involves iterating through the characters of the string, detecting specific conditions, and extracting substrings accordingly.
This method is particularly useful when dealing with dynamic or complex string formats.
Let’s write a basic example to illustrate the fundamental concept of manual string parsing:
String originalString = "sensor1:value1;sensor2:value2";
char delimiter = ':';
void setup() {
Serial.begin(9600);
int startIndex = 0;
// Iterate through the string
for (int i = 0; i < originalString.length(); i++) {
if (originalString[i] == delimiter) {
// Extract substring
String substring = originalString.substring(startIndex, i);
Serial.println(substring);
// Update the start index
startIndex = i + 1;
}
}
// Print the last substring
String lastSubstring = originalString.substring(startIndex);
Serial.println(lastSubstring);
}
void loop() {
// Your main code here
}
Output:
This code manually parses the string "sensor1:value1;sensor2:value2"
using a colon ':'
as a char
separator. In the setup()
function, a loop iterates through the characters, extracting substrings between colons and printing them.
The last substring, which may not end with the delimiter, is printed separately. This code demonstrates a manual string parsing technique for extracting substrings based on a specified delimiter, providing control over the process.
The loop()
function is left empty, as it is typically used for the main continuous code in an Arduino sketch.
Conclusion
This article explores diverse methods for splitting strings in Arduino, addressing the essential task of string manipulation. It covers the usage of the substring()
function, providing detailed explanations and example code for extracting specific portions of a string based on defined start and end indices.
The dynamic splitting capabilities of the indexOf()
function are discussed, especially useful for scenarios where the index of the splitting character is unknown.
The article further demonstrates the process of splitting strings based on newline characters using the lastIndexOf()
and substring()
functions, offering a practical example.
It introduces the C standard library function strtok()
for string tokenization, exemplified through an application of splitting names separated by commas. Lastly, manual string parsing is introduced as a method for fine-grained control over substring extraction.
By presenting a diverse range of methods with clear explanations and example codes, the article equips Arduino programmers with a comprehensive understanding of string-splitting techniques. Whether dealing with specific indices, dynamic scenarios, or complex string formats, the knowledge gained from this article empowers programmers to confidently address various string manipulation challenges in their Arduino projects.