Arduino Strcpy Function
-
Introduction to Arduino
strcpy()
Function -
Use the
strcpy()
Function to Copy a String From One Variable to Another -
Use the
strncpy()
Function for Limited Length Copy -
Use the
strlcpy()
Function for Limited Length Copy -
Use the
strcpy()
Function to Copy a String From User Input via Serial - Conclusion
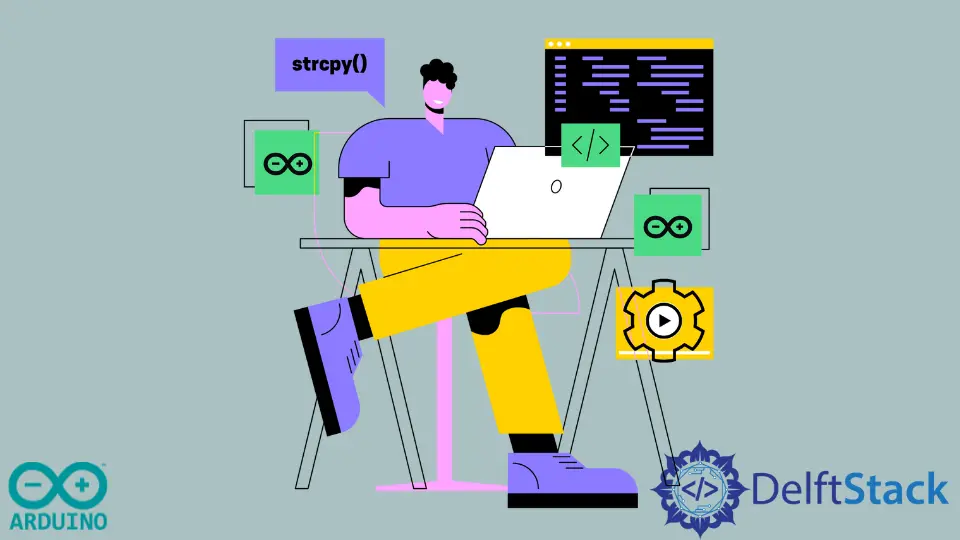
The strcpy()
function is a fundamental tool for manipulating strings, allowing you to copy one string from a source variable to a destination variable. Understanding how to use strcpy()
properly is essential for efficient string handling in your Arduino projects.
In this tutorial, we will discuss copying one string from one variable to another using the strcpy()
function in Arduino.
Introduction to Arduino strcpy()
Function
The strcpy()
function can copy a string, including the NULL character, from one variable to another.
Syntax:
output = strcpy(destination, source);
Parameters:
destination
: A character array where thesource
string will be copied.source
: Thesource
string, which is a constant character array.
The first input of the strcpy()
function should have the data type char
, and the second input should be the data type const char
. This function will return the copied string as a character string.
If the destination
size exceeds the source
size, the strcpy()
function will also add a NULL character as a terminator inside the destination
string. If the destination
string already has a string stored, the previous string will be overwritten by the new string.
Use the strcpy()
Function to Copy a String From One Variable to Another
For example, let’s define a string and copy it into another variable using the strcpy()
function. See the code below.
void setup() {
const char* source = "Hello World";
char destination[17];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
Output:
Hello World
In the above code, we have used the strcpy()
function to copy the Hello World
string from the source
array to the destination
array. Make sure the destination
array has enough space to hold the entire source
string.
Buffer size is critical when using strcpy()
because buffer overflows can lead to unexpected behavior in your code. Arduino will not show an error because of overflow, and it might take a while to figure out the problem.
To avoid this, make sure the destination
buffer is large enough to accommodate the source
string.
In the code below, we intentionally reduced the size of the destination
variable to 5
, which is less than the source
string’s length of 17
. Surprisingly, when using strcpy()
, the output is Hello World
.
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
Output:
Hello World
The output Hello World
is unexpected, given that the size of the destination
array is smaller than the source
string. In standard C/C++ programming, this would lead to a buffer overflow, which can result in unpredictable behavior.
However, Arduino’s implementation of strcpy()
might handle this situation differently, and in our case, it seems to copy the entire string without causing a noticeable buffer overflow.
Note: Keep in mind that this behavior may not be consistent across different systems or compilers, and it’s generally not a good practice to rely on such behavior. In order to ensure code reliability and avoid potential issues, it’s recommended to allocate
destination
arrays with sizes that can accommodate thesource
data without overflow.
Use the strncpy()
Function for Limited Length Copy
Using the strncpy()
function is a common approach when we want to copy a limited portion of one string into another string while ensuring that the destination
string doesn’t exceed a specified length.
The strncpy()
function will write NULL characters to fill the remaining space of the destination
string only if a NULL character is encountered from the source
string. If the source
string does not have a NULL character, the destination
string will not be terminated with a NULL character.
The syntax below is used to copy a portion of one string (source
) into another string (destination
) while specifying a maximum length (length
) of characters to copy.
Syntax:
strncpy(destination, source, length);
Parameters:
destination
: A character array where thesource
string will be copied.source
: Thesource
string, which is a constant character array.length
: The maximum number of characters to copy from thesource
to thedestination
. It ensures that only up tolength
characters are copied, preventing buffer overflow.
For example, let’s repeat the above example using the strncpy()
function. See the code below.
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strncpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
Output:
Hello
The output of this example contains the first 5 characters of the source
. So, if we use the strncpy()
function, we don’t have to care about the overflow of the function because strncpy()
will copy the number of characters of the source
according to the destination
size.
This function is also useful when we don’t want to copy the whole string and only want to copy a few characters from the source
to the destination
. The strcpy()
and strncpy()
functions also return the copied string of the char
data type.
Use the strlcpy()
Function for Limited Length Copy
The strlcpy()
is another length-limited version of strcpy()
. Unlike strncpy()
, the strlcpy()
ensures that the destination string is always null-terminated and that the length of the destination
includes the null character.
The strlcpy()
function, unlike strncpy()
, writes only a single NULL character to the destination
string and doesn’t fill with multiple NULL characters. This ensures that a single NULL character always terminates the destination
string.
The syntax below is used to copy a portion of one string (source
) into another string (destination
) while specifying a maximum size (size
) of characters to copy.
Syntax:
strlcpy(destination, source, size);
Parameters:
destination
: The target character array where characters from thesource
string will be copied. It should have adequate space to hold the copied data.source
: The original string containing the text to be copied into thedestination
array.size
: The maximum number of characters to copy fromsource
todestination
. This helps prevent buffer overflows.
It’s important to note that the characters stored in the destination
string include the NULL character. For instance, if the destination
string’s size is 5
, you can copy only four characters due to the presence of the NULL character.
To copy 5
characters, you would need to increase the destination
string’s size to 6
. This behavior contrasts with strcpy()
and strncpy()
, which add a NULL character only if the destination
size exceeds the source
size.
For example, let’s repeat the above example using the strlcpy()
function. See the code below.
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strlcpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
Output:
Hell
As we can see in the output, four characters of the source
string have been copied to the destination
even if the destination
size is 5
. This is because the strlcpy()
function also added the NULL character at the end of the destination
string.
Now, in the following section, let’s have another example of using the strcpy()
function in Arduino.
Use the strcpy()
Function to Copy a String From User Input via Serial
Copying a string from user input via the strcpy()
function in Arduino involves reading text entered by the user through the Arduino Serial Monitor and then copying that text into a character array using the strcpy()
function. This allows you to store and manipulate the user’s input within your Arduino sketch.
char inputBuffer[64];
char destination[64];
void setup() {
Serial.begin(9600);
Serial.println("Enter a string: ");
}
void loop() {
if (Serial.available()) {
int bytesRead =
Serial.readBytesUntil('\n', inputBuffer, sizeof(inputBuffer) - 1);
inputBuffer[bytesRead] = '\0';
strcpy(destination, inputBuffer);
Serial.print("Copied string: ");
Serial.println(destination);
memset(inputBuffer, 0, sizeof(inputBuffer));
}
}
Output:
Enter a string:
Copied string: Hi
In the above code, we set up a connection with the Serial Monitor, which allows communication between the Arduino and your computer. When you run the code, you’ll see a message on the Serial Monitor that says "Enter a string: "
.
The code keeps an eye on the Serial Monitor for any input you provide. When you type "Hi"
and press Enter, the code recognizes your input using the Serial.available()
function.
The Serial.readBytesUntil('\n', inputBuffer, sizeof(inputBuffer) - 1)
part reads the characters you type until it reaches the Enter key, storing them in a memory space called inputBuffer
. It makes sure not to take up too much space, and it also adds a special character (null-terminator) to signal the end of your input.
Next, the code copies what you typed from inputBuffer
to another place called destination
using the strcpy()
function. This allows the code to work with your input.
As a result, you’ll see the copied input displayed on the Serial Monitor as "Copied string: Hi"
.
Conclusion
In conclusion, the strcpy()
function in Arduino is a powerful tool for string manipulation, enabling you to copy strings, read user input, and build dynamic strings. However, it’s important to manage buffer sizes carefully to avoid overflows and ensure your code functions as expected.
Whether you’re working with sensor data or user input, understanding how to use strcpy()
effectively is essential for successful Arduino projects.