Arduino strcpy 함수
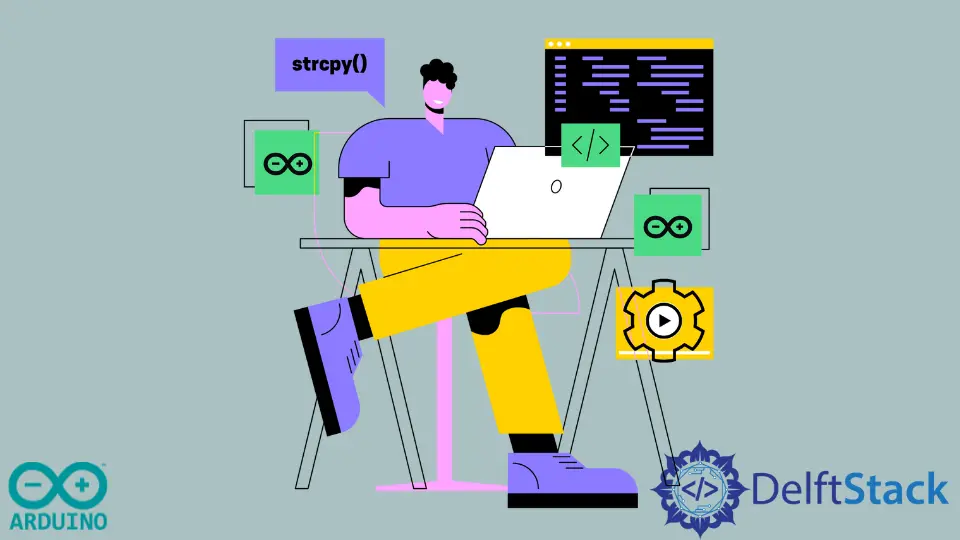
이 튜토리얼에서는 Arduino의 strcpy()
함수를 사용하여 한 변수에서 다른 변수로 문자열을 복사하는 방법에 대해 설명합니다.
Arduino strcpy()
기능
strcpy()
함수는 널 문자를 포함하는 문자열을 한 변수에서 다른 변수로 복사할 수 있습니다. strcpy()
함수의 기본 구문은 다음과 같습니다.
output = strcpy(dest, source);
strcpy()
함수의 첫 번째 입력은 char 데이터 유형이어야 하고 두 번째 입력은 데이터 유형 const char이어야 합니다. 이 함수는 복사된 문자열을 문자열로 반환합니다.
대상 크기가 소스 크기를 초과하는 경우 strcpy()
함수는 대상 문자열 내부에 종료자로 NUL 문자도 추가합니다. 대상 문자열에 이미 저장된 문자열이 있는 경우 새 문자열이 이전 문자열을 덮어씁니다.
예를 들어, 문자열을 정의하고 strcpy()
함수를 사용하여 다른 변수에 복사해 보겠습니다. 아래 코드를 참조하십시오.
void setup() {
const char* source = "Hello World";
char destination[17];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
출력:
Hello World
위의 코드에서는 destination
변수에 저장된 문자열을 출력하기 위해 Arduino의 직렬 모니터를 사용했습니다. destination
변수의 길이는 source
문자열 전체를 담을 수 있을 만큼 충분히 커야 합니다.
길이가 source
문자열보다 작으면 결과가 변경되고 strcpy()
함수는 정의되지 않은 동작을 합니다.
source
문자열과 destination
문자열의 크기 차이로 인해 strcpy()
함수가 오버플로되어 코드에 문제가 발생합니다. Arduino는 오버플로로 인해 오류를 표시하지 않으며 문제를 파악하는 데 시간이 걸릴 수 있습니다.
예를 들어 destination
변수의 크기를 위 코드에서 17
인 5
로 변경하고 결과를 확인해보자. 아래 코드를 참조하십시오.
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
출력:
Èõüÿ
위의 출력에서 볼 수 있듯이 destination
변수의 크기가 source
변수의 크기보다 작기 때문에 결과가 변경되었습니다. 좋은 결과를 얻으려면 destination
변수의 크기가 source
변수의 크기보다 커야 합니다.
strncpy()
인 strcpy()
함수의 길이 제한 버전을 사용할 수 있습니다. strncpy()
함수는 source
문자열도 destination
변수에 복사하지만 대상의 길이도 입력으로 사용합니다.
strncpy()
함수는 source
문자열에서 NUL 문자가 발생한 경우에만 destination
문자열의 나머지 공간을 채우기 위해 NUL 문자를 작성합니다. source
문자열에 NUL 문자가 없으면 destination
문자열은 NUL 문자로 끝나지 않습니다.
예를 들어 strncpy()
함수를 사용하여 위의 예를 반복해 보겠습니다. 아래 코드를 참조하십시오.
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strncpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
출력:
Hello
이 예의 출력에는 source
의 처음 5자가 포함됩니다. 따라서 strncpy()
함수를 사용하면 strncpy()
가 destination
크기에 따라 source
의 문자 수를 복사하기 때문에 함수의 오버플로에 대해 걱정할 필요가 없습니다. .
이 함수는 전체 문자열을 복사하고 싶지 않고 원본에서 대상으로 몇 문자만 복사하려는 경우에도 유용합니다. strcpy()
및 strncpy()
함수는 char 데이터 유형의 복사된 문자열도 반환합니다.
strlcpy()
함수인 strcpy()
함수의 또 다른 길이 제한 버전이 있습니다. strlcpy()
함수는 strncpy()
함수와 동일하고 차이점은 strlcpy()
함수의 출력은 소스 문자열의 길이라는 것입니다.
strncpy()
함수와 달리 strlcpy()
함수는 destination
문자열의 나머지 공간을 채우기 위해 여러 NUL 문자를 쓰지 않으며 destination
문자열에 단일 NUL 문자만 씁니다. destination
문자열은 strlcpy()
함수를 사용하여 항상 단일 NUL 문자로 종료됩니다.
destination
문자열 내부에 저장된 문자에는 NUL 문자도 포함됩니다. 예를 들어 destination
문자열의 크기가 5이면 NUL 문자 때문에 4자만 복사할 수 있습니다.
따라서 destination
문자열의 크기를 6으로 늘려 5자를 복사해야 합니다. 그러나 destination
문자열의 크기가 source
문자열의 크기보다 큰 경우에만 NUL 문자를 추가하는 strcpy()
및 strncpy()
함수의 경우는 아닙니다.
예를 들어 strlcpy()
함수를 사용하여 위의 예를 반복해 보겠습니다. 아래 코드를 참조하십시오.
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strlcpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
출력:
Hell
출력에서 볼 수 있듯이 destination
크기가 5인 경우에도 source
문자열의 4개 문자가 destination
에 복사되었습니다. 이는 strlcpy()
함수가 destination
문자열의 끝에 NUL 문자도 추가했기 때문입니다.