Arduino strcpy 函式
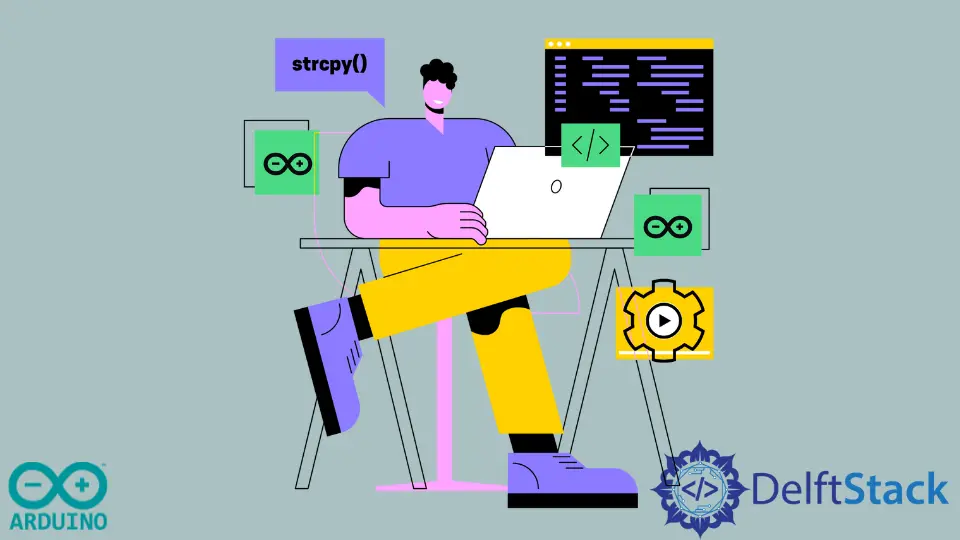
在本教程中,我們將討論使用 Arduino 中的 strcpy()
函式將一個字串從一個變數複製到另一個變數。
Arduino strcpy()
函式
strcpy()
函式可以將包含空字元的字串從一個變數複製到另一個變數。strcpy()
函式的基本語法如下所示。
output = strcpy(dest, source);
strcpy()
函式的第一個輸入應該是資料型別 char,第二個輸入應該是資料型別 const char。該函式將複製的字串作為字串返回。
如果目標大小超過源大小,strcpy()
函式還將在目標字串中新增一個 NUL 字元作為終止符。如果目標字串已經儲存了一個字串,則先前的字串將被新字串覆蓋。
例如,讓我們定義一個字串並使用 strcpy()
函式將其複製到另一個變數中。請參閱下面的程式碼。
void setup() {
const char* source = "Hello World";
char destination[17];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
輸出:
Hello World
在上面的程式碼中,我們使用 Arduino 的序列監視器來列印字串,該字串儲存在 destination
變數中。destination
變數的長度應該足夠大以容納整個 source
字串。
如果長度小於 source
字串,結果將被更改,strcpy()
函式將具有未定義的行為。
由於 source
和 destination
字串的大小不同,strcpy()
函式會溢位,從而導致程式碼出現問題。Arduino 不會因為溢位而顯示錯誤,可能需要一段時間才能找出問題所在。
例如,讓我們將 destination
變數的大小更改為 5
,也就是上面程式碼中的 17
,然後檢查結果。請參閱下面的程式碼。
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
輸出:
Èõüÿ
正如我們在上面的輸出中看到的,結果已經改變,因為 destination
變數的大小小於 source
變數的大小。為了獲得好的結果,我們必須確保 destination
變數的大小大於 source
變數的大小。
我們可以使用 strcpy()
函式的長度限制版本,即 strncpy()
。strncpy()
函式還將 source
字串複製到 destination
變數,但它也將目標的長度作為輸入。
僅當從 source
字串中遇到 NUL 字元時,strncpy()
函式才會寫入 NUL 字元以填充 destination
字串的剩餘空間。如果 source
字串沒有 NUL 字元,destination
字串將不會以 NUL 字元結尾。
例如,讓我們使用 strncpy()
函式重複上述示例。請參閱下面的程式碼。
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strncpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
輸出:
Hello
此示例的輸出包含 source
的前 5 個字元。所以,如果我們使用 strncpy()
函式,我們不必關心函式的溢位,因為 strncpy()
會根據 destination
大小複製 source
的字元數.
當我們不想複製整個字串而只想將幾個字元從源複製到目標時,此函式也很有用。strcpy()
和 strncpy()
函式還返回 char 資料型別的複製字串。
strcpy()
函式還有另一個長度限制版本,即 strlcpy()
函式。strlcpy()
函式與 strncpy()
函式相同,不同之處在於 strlcpy()
函式的輸出是源字串的長度。
與 strncpy()
函式不同,strlcpy()
函式不會寫入多個 NUL 字元來填充 destination
字串的剩餘空間,它只會將單個 NUL 字元寫入 destination
字串。destination
字串將始終使用 strlcpy()
函式以單個 NUL 字元終止。
destination
字串中儲存的字元也將包括 NUL 字元。例如,如果 destination
字串的大小為 5,由於 NUL 字元,我們只能在其中複製四個字元。
所以我們必須將 destination
字串的大小增加到 6 以在其中複製 5 個字元。但是,strcpy()
和 strncpy()
函式並非如此,它們僅在 destination
字串的大小大於 source
字串的大小時新增 NUL 字元。
例如,讓我們使用 strlcpy()
函式重複上述示例。請參閱下面的程式碼。
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strlcpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
輸出:
Hell
正如我們在輸出中看到的那樣,即使 destination
大小為 5,source
字串的四個字元也已複製到 destination
。這是因為 strlcpy()
函式還在 destination
字串的結尾。