Arduino strcpy 函数
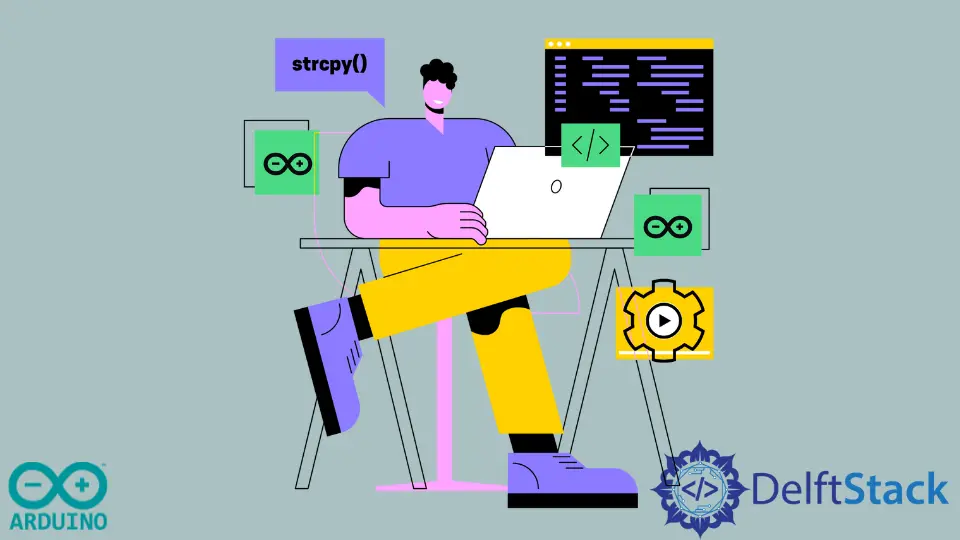
在本教程中,我们将讨论使用 Arduino 中的 strcpy()
函数将一个字符串从一个变量复制到另一个变量。
Arduino strcpy()
函数
strcpy()
函数可以将包含空字符的字符串从一个变量复制到另一个变量。strcpy()
函数的基本语法如下所示。
output = strcpy(dest, source);
strcpy()
函数的第一个输入应该是数据类型 char,第二个输入应该是数据类型 const char。该函数将复制的字符串作为字符串返回。
如果目标大小超过源大小,strcpy()
函数还将在目标字符串中添加一个 NUL 字符作为终止符。如果目标字符串已经存储了一个字符串,则先前的字符串将被新字符串覆盖。
例如,让我们定义一个字符串并使用 strcpy()
函数将其复制到另一个变量中。请参阅下面的代码。
void setup() {
const char* source = "Hello World";
char destination[17];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
输出:
Hello World
在上面的代码中,我们使用 Arduino 的串行监视器来打印字符串,该字符串存储在 destination
变量中。destination
变量的长度应该足够大以容纳整个 source
字符串。
如果长度小于 source
字符串,结果将被更改,strcpy()
函数将具有未定义的行为。
由于 source
和 destination
字符串的大小不同,strcpy()
函数会溢出,从而导致代码出现问题。Arduino 不会因为溢出而显示错误,可能需要一段时间才能找出问题所在。
例如,让我们将 destination
变量的大小更改为 5
,也就是上面代码中的 17
,然后检查结果。请参阅下面的代码。
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strcpy(destination, source);
Serial.println(destination);
}
void loop() {}
输出:
Èõüÿ
正如我们在上面的输出中看到的,结果已经改变,因为 destination
变量的大小小于 source
变量的大小。为了获得好的结果,我们必须确保 destination
变量的大小大于 source
变量的大小。
我们可以使用 strcpy()
函数的长度限制版本,即 strncpy()
。strncpy()
函数还将 source
字符串复制到 destination
变量,但它也将目标的长度作为输入。
仅当从 source
字符串中遇到 NUL 字符时,strncpy()
函数才会写入 NUL 字符以填充 destination
字符串的剩余空间。如果 source
字符串没有 NUL 字符,destination
字符串将不会以 NUL 字符结尾。
例如,让我们使用 strncpy()
函数重复上述示例。请参阅下面的代码。
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strncpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
输出:
Hello
此示例的输出包含 source
的前 5 个字符。所以,如果我们使用 strncpy()
函数,我们不必关心函数的溢出,因为 strncpy()
会根据 destination
大小复制 source
的字符数.
当我们不想复制整个字符串而只想将几个字符从源复制到目标时,此函数也很有用。strcpy()
和 strncpy()
函数还返回 char 数据类型的复制字符串。
strcpy()
函数还有另一个长度限制版本,即 strlcpy()
函数。strlcpy()
函数与 strncpy()
函数相同,不同之处在于 strlcpy()
函数的输出是源字符串的长度。
与 strncpy()
函数不同,strlcpy()
函数不会写入多个 NUL 字符来填充 destination
字符串的剩余空间,它只会将单个 NUL 字符写入 destination
字符串。destination
字符串将始终使用 strlcpy()
函数以单个 NUL 字符终止。
destination
字符串中存储的字符也将包括 NUL 字符。例如,如果 destination
字符串的大小为 5,由于 NUL 字符,我们只能在其中复制四个字符。
所以我们必须将 destination
字符串的大小增加到 6 以在其中复制 5 个字符。但是,strcpy()
和 strncpy()
函数并非如此,它们仅在 destination
字符串的大小大于 source
字符串的大小时添加 NUL 字符。
例如,让我们使用 strlcpy()
函数重复上述示例。请参阅下面的代码。
void setup() {
const char* source = "Hello World";
char destination[5];
Serial.begin(9600);
strlcpy(destination, source, sizeof(destination));
Serial.println(destination);
}
void loop() {}
输出:
Hell
正如我们在输出中看到的那样,即使 destination
大小为 5,source
字符串的四个字符也已复制到 destination
。这是因为 strlcpy()
函数还在 destination
字符串的结尾。