在 Arduino 中连接字符串
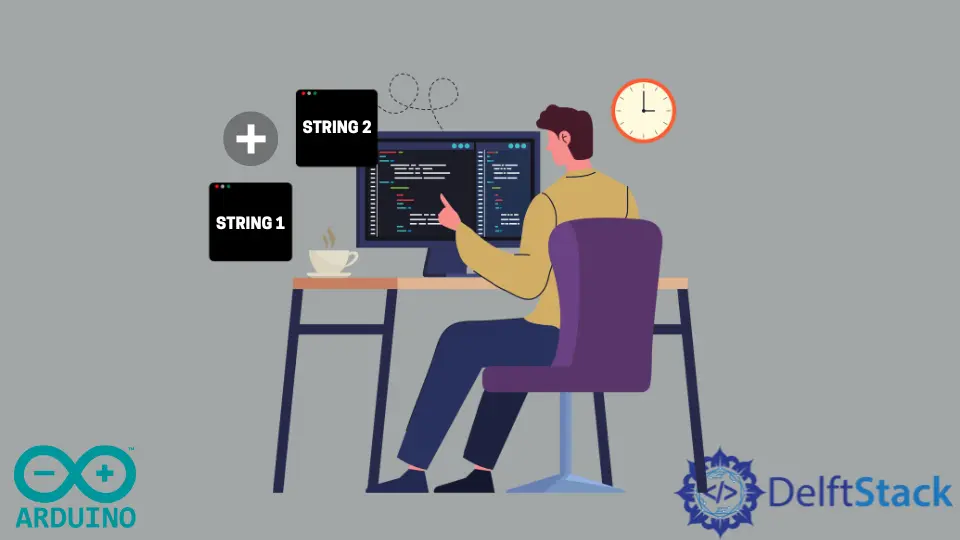
本教程将讨论使用 concat()
函数或 Arduino 中的附加运算符连接两个字符串。
使用 Arduino 中的 concat()
函数连接字符串
我们可以使用 concat()
函数在 Arduino 中连接两个字符串。concat()
函数将给给定的参数附加一个字符串。
如果连接操作成功,它将返回 true
,如果失败,则返回 false
。
concat()
函数的基本语法如下所示。
MyString.concat(MyParameter);
在上面的语法中,MyString
变量是一个字符串对象,其中存储了一个字符串,而 MyParameter
变量包含我们要附加到字符串的参数。参数可以是数据类型 long、int、double、float、char、byte 和 string。
请注意,在执行 concat()
函数后,存储在 MyString
变量中的字符串将被更改,因为给定的参数将附加到它,结果将保存在 MyString
变量中。
假设我们不想更改原始字符串。在这种情况下,我们可以创建另一个字符串变量,它也包含与第一个变量相同的字符串,我们将在 concat()
函数中使用第二个字符串变量。
例如,让我们创建两个字符串变量并使用 concat()
函数将它们连接起来。请参阅下面的代码。
void setup() {
String s1 = "hello";
String s2 = " World";
s1.concat(s2);
Serial.begin(9600);
Serial.println(s1);
}
void loop() {}
输出:
hello World
在上面的代码中,我们使用了 Arduino 的串口监视器来显示连接的结果。我们在第二个字符串变量中使用了空格,它也将出现在输出中的两个字符串之间。
我们还可以在条件语句中使用 concat()
函数的输出来检查连接操作是成功还是失败。
例如,我们可以使用 if
语句来检查 concat()
函数的输出。如果输出为真,我们将打印一条消息,表明操作已成功;如果输出为假,我们将打印操作不成功。
例如,让我们连接两个字符串并根据 concat()
函数的输出显示成功或失败消息。请参阅下面的代码。
void setup() {
String s1 = "hello";
String s2 = " World";
bool b = s1.concat(s2);
Serial.begin(9600);
Serial.println(s1);
if (b) {
Serial.println("operation has been successful");
} else {
Serial.println("operation is not successful");
}
}
void loop() {}
输出:
hello World
operation has been successful
在上面的代码中,我们使用布尔变量 b
来存储 concat()
函数的输出,并且我们使用 if
语句来检查布尔值。如果输出为真,成功的信息将打印在串口监视器上,如果输出为假,另一条信息将打印在串口监视器上。
在上面的输出中,我们可以看到连接成功。查看此链接以获取有关 concat()
函数的更多详细信息。
在 Arduino 中使用附加运算符 +
连接字符串
我们还可以使用附加运算符+
连接其他数据类型的字符串或变量,允许的数据类型与 concat()
函数相同。我们还可以使用 append 运算符在一行中多次连接多个字符串或其他数据类型的变量。
下面给出了与附加运算符连接的基本语法。
MyString = Parameter1 + parameter2 + ... + parameterN;
在上述语法中,MyString
变量是一个用于存储输出的字符串对象,参数包含我们要附加其他参数的值。参数可以是数据类型 long、int、double、float、char、byte 和 string。
例如,让我们创建两个字符串变量和一个整数变量,并使用附加运算符将它们连接起来。请参阅下面的代码。
void setup() {
String s1 = "hello";
String s2 = " World";
int n = 10;
String s3 = s1 + " ,,," + s2 + " " + n;
Serial.begin(9600);
Serial.println(s3);
}
void loop() {}
输出:
hello ,,, World 10
在上面的代码中,我们创建了一个字符串对象来存储连接结果,我们还使用了其他字符串,如三个逗号和一个空格的字符串。在其他数据类型的情况下,追加运算符将数据类型转换为字符串数据类型,然后将它们与其他字符串对象追加。
在一行中附加多个字符串的缺点是它也会占用大量内存,而 Arduino 的内存要少得多。查看此链接以获取有关附加运算符的更多详细信息。