在 Arduino 中連線字串
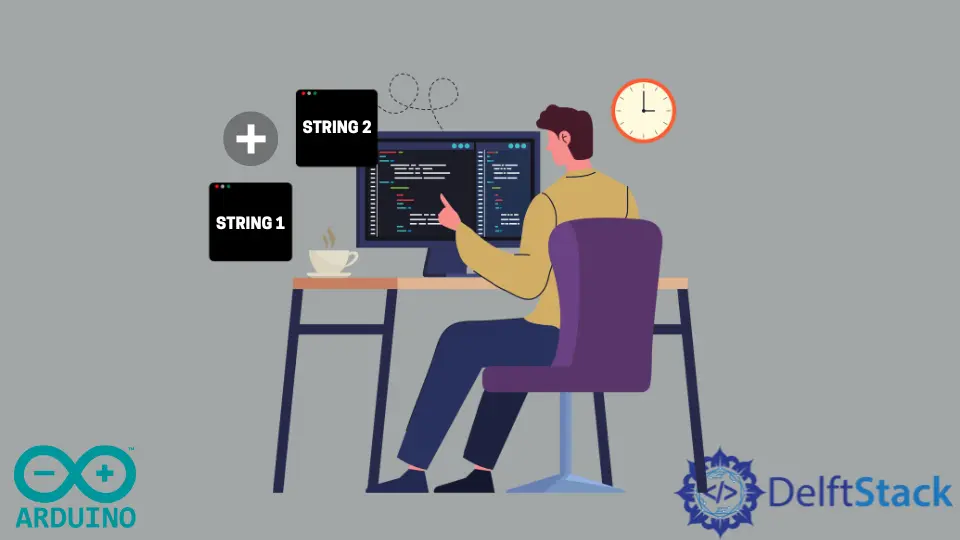
本教程將討論使用 concat()
函式或 Arduino 中的附加運算子連線兩個字串。
使用 Arduino 中的 concat()
函式連線字串
我們可以使用 concat()
函式在 Arduino 中連線兩個字串。concat()
函式將給給定的引數附加一個字串。
如果連線操作成功,它將返回 true
,如果失敗,則返回 false
。
concat()
函式的基本語法如下所示。
MyString.concat(MyParameter);
在上面的語法中,MyString
變數是一個字串物件,其中儲存了一個字串,而 MyParameter
變數包含我們要附加到字串的引數。引數可以是資料型別 long、int、double、float、char、byte 和 string。
請注意,在執行 concat()
函式後,儲存在 MyString
變數中的字串將被更改,因為給定的引數將附加到它,結果將儲存在 MyString
變數中。
假設我們不想更改原始字串。在這種情況下,我們可以建立另一個字串變數,它也包含與第一個變數相同的字串,我們將在 concat()
函式中使用第二個字串變數。
例如,讓我們建立兩個字串變數並使用 concat()
函式將它們連線起來。請參閱下面的程式碼。
void setup() {
String s1 = "hello";
String s2 = " World";
s1.concat(s2);
Serial.begin(9600);
Serial.println(s1);
}
void loop() {}
輸出:
hello World
在上面的程式碼中,我們使用了 Arduino 的串列埠監視器來顯示連線的結果。我們在第二個字串變數中使用了空格,它也將出現在輸出中的兩個字串之間。
我們還可以在條件語句中使用 concat()
函式的輸出來檢查連線操作是成功還是失敗。
例如,我們可以使用 if
語句來檢查 concat()
函式的輸出。如果輸出為真,我們將列印一條訊息,表明操作已成功;如果輸出為假,我們將列印操作不成功。
例如,讓我們連線兩個字串並根據 concat()
函式的輸出顯示成功或失敗訊息。請參閱下面的程式碼。
void setup() {
String s1 = "hello";
String s2 = " World";
bool b = s1.concat(s2);
Serial.begin(9600);
Serial.println(s1);
if (b) {
Serial.println("operation has been successful");
} else {
Serial.println("operation is not successful");
}
}
void loop() {}
輸出:
hello World
operation has been successful
在上面的程式碼中,我們使用布林變數 b
來儲存 concat()
函式的輸出,並且我們使用 if
語句來檢查布林值。如果輸出為真,成功的資訊將列印在串列埠監視器上,如果輸出為假,另一條資訊將列印在串列埠監視器上。
在上面的輸出中,我們可以看到連線成功。檢視此連結以獲取有關 concat()
函式的更多詳細資訊。
在 Arduino 中使用附加運算子 +
連線字串
我們還可以使用附加運算子+
連線其他資料型別的字串或變數,允許的資料型別與 concat()
函式相同。我們還可以使用 append 運算子在一行中多次連線多個字串或其他資料型別的變數。
下面給出了與附加運算子連線的基本語法。
MyString = Parameter1 + parameter2 + ... + parameterN;
在上述語法中,MyString
變數是一個用於儲存輸出的字串物件,引數包含我們要附加其他引數的值。引數可以是資料型別 long、int、double、float、char、byte 和 string。
例如,讓我們建立兩個字串變數和一個整數變數,並使用附加運算子將它們連線起來。請參閱下面的程式碼。
void setup() {
String s1 = "hello";
String s2 = " World";
int n = 10;
String s3 = s1 + " ,,," + s2 + " " + n;
Serial.begin(9600);
Serial.println(s3);
}
void loop() {}
輸出:
hello ,,, World 10
在上面的程式碼中,我們建立了一個字串物件來儲存連線結果,我們還使用了其他字串,如三個逗號和一個空格的字串。在其他資料型別的情況下,追加運算子將資料型別轉換為字串資料型別,然後將它們與其他字串物件追加。
在一行中附加多個字串的缺點是它也會佔用大量記憶體,而 Arduino 的記憶體要少得多。檢視此連結以獲取有關附加運算子的更多詳細資訊。