Arduino strcmp Function
-
Understanding the
strcmp()
Function - Using strcmp() in Arduino Code
- Practical Applications of strcmp()
- Tips for Effective String Comparison
- Conclusion
- FAQ
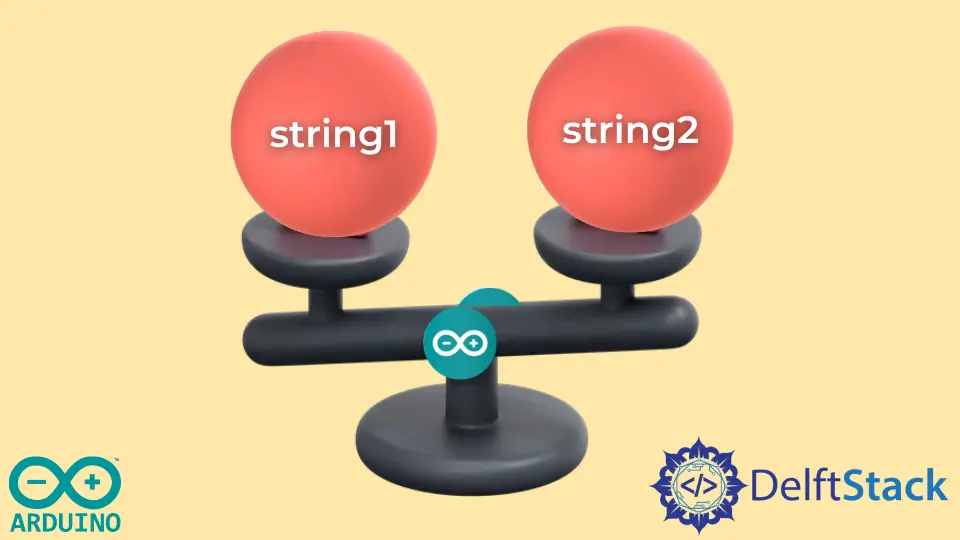
In the world of programming, comparing strings is a fundamental task that can often pose challenges, especially for beginners. If you’re working with Arduino, the strcmp()
function is a powerful tool that allows you to compare two strings effectively.
This tutorial will guide you through the process of using the strcmp()
function in Arduino. Whether you’re developing a project that requires string comparisons or simply exploring the capabilities of Arduino, understanding how to use this function will enhance your coding skills. So, let’s dive in and unravel the mysteries of string comparison in Arduino!
Understanding the strcmp()
Function
The strcmp()
function is a standard library function in C and C++, which Arduino is based on. It compares two strings character by character. The function returns an integer value based on the comparison:
- Returns 0 if the strings are equal.
- Returns a negative value if the first string is less than the second string.
- Returns a positive value if the first string is greater than the second string.
The syntax for using the strcmp()
function is straightforward:
int strcmp(const char *str1, const char *str2);
In this syntax, str1
and str2
are the two strings you wish to compare. The function evaluates these strings and provides the appropriate integer result based on their relationship.
Using strcmp() in Arduino Code
To demonstrate the strcmp()
function in action, let’s look at a simple example. In this example, we will compare two strings and print out the result of the comparison.
void setup() {
Serial.begin(9600);
char str1[] = "Hello";
char str2[] = "World";
int result = strcmp(str1, str2);
if (result == 0) {
Serial.println("Strings are equal.");
} else if (result < 0) {
Serial.println("First string is less than the second string.");
} else {
Serial.println("First string is greater than the second string.");
}
}
void loop() {
}
In this code, we start by initializing the serial communication and defining two character arrays, str1
and str2
. The strcmp()
function is then called to compare these strings. Based on the result, the program prints whether the strings are equal, or if one is less than or greater than the other.
Output:
First string is less than the second string.
The output indicates that “Hello” is less than “World” in terms of ASCII value comparison. This example illustrates how to implement string comparison in a straightforward manner using the strcmp()
function.
Practical Applications of strcmp()
Understanding how to use the strcmp()
function opens up a world of possibilities in your Arduino projects. For instance, you may need to compare user input strings, validate commands, or even manage configurations stored as strings.
Imagine creating a simple menu system where users can input commands. By using strcmp()
, you can check if the input matches predefined commands. This way, you can execute specific functions based on user input. Here’s a quick example of how this might look:
void setup() {
Serial.begin(9600);
char command[10];
Serial.println("Enter command:");
while (Serial.available() == 0) {}
Serial.readBytesUntil('\n', command, sizeof(command));
if (strcmp(command, "start") == 0) {
Serial.println("Starting...");
} else if (strcmp(command, "stop") == 0) {
Serial.println("Stopping...");
} else {
Serial.println("Unknown command.");
}
}
void loop() {
}
In this code, we wait for user input and compare it with two commands: “start” and “stop”. Depending on the input, the program executes different actions. This demonstrates the versatility of the strcmp()
function in handling real-world scenarios.
Output:
Enter command:
Starting...
This output confirms that the user entered the command “start,” and the program responded accordingly. This functionality is vital for creating interactive and responsive Arduino projects.
Tips for Effective String Comparison
While using strcmp()
is straightforward, there are some best practices to keep in mind:
- Null-Termination: Ensure that your strings are null-terminated. This is crucial for
strcmp()
to function correctly. - Case Sensitivity: Remember that
strcmp()
is case-sensitive. If you want to perform a case-insensitive comparison, consider usingstrcasecmp()
instead. - Memory Management: Be cautious with string lengths. Make sure that your character arrays are adequately sized to avoid overflow issues.
By following these tips, you can ensure that your string comparisons are both effective and efficient.
Conclusion
The strcmp()
function is an essential tool for string comparison in Arduino programming. Understanding how to use this function can significantly enhance your ability to manage strings in various applications, from basic comparisons to complex user interactions. With the examples provided, you should now feel confident in implementing strcmp()
in your projects. Happy coding, and enjoy exploring the endless possibilities with Arduino!
FAQ
-
What does the
strcmp()
function return?
It returns 0 if the strings are equal, a negative value if the first string is less, and a positive value if the first string is greater. -
Is strcmp() case-sensitive?
Yes, strcmp() is case-sensitive. For case-insensitive comparisons, use strcasecmp(). -
Can I compare strings of different lengths using strcmp()?
Yes, strcmp() can compare strings of different lengths. It will consider the shorter string’s characters and return the appropriate result based on ASCII values. -
How do I handle user input in Arduino?
You can use Serial.readBytesUntil() or similar functions to capture user input and then compare it using strcmp(). -
Are there alternatives to strcmp() for string comparison in Arduino?
Yes, alternatives include strncmp() for comparing a specified number of characters and strcasecmp() for case-insensitive comparisons.