How to Print Multiple Variables in Arduino Serial
-
Print Variable Values on Serial Monitor Using the
Serial.print()
Function in Arduino -
Print Variable Values on Serial Monitor Using the
Serial.println()
Function in Arduino - Conclusion
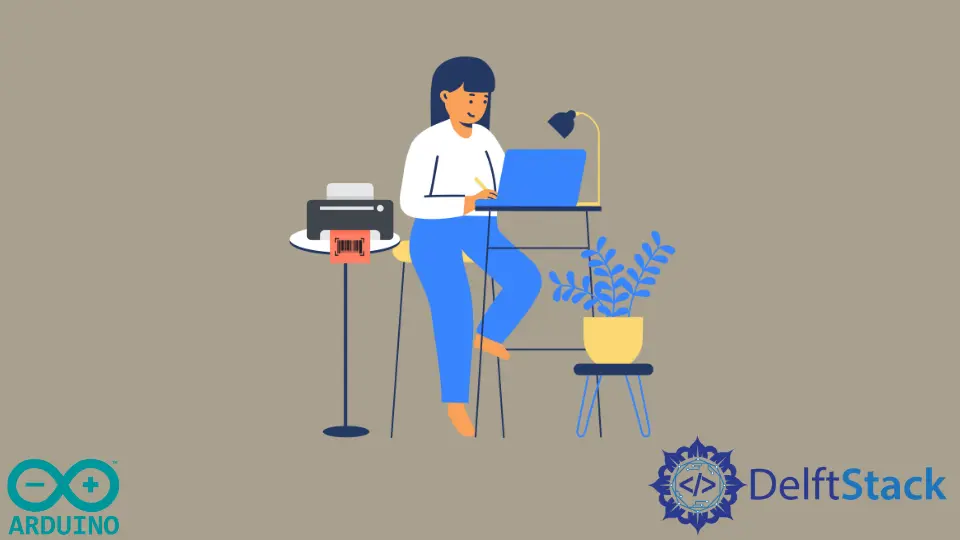
In this tutorial, we’ll look into the use of the Serial.print()
and Serial.println()
functions in Arduino for displaying variable values on the serial monitor. These functions provide a vital means of monitoring and debugging your Arduino programs.
Serial.print()
allows us to print variables or data on the same line, while Serial.println()
accomplishes the same task but automatically moves to the next line, ensuring a well-organized output.
Print Variable Values on Serial Monitor Using the Serial.print()
Function in Arduino
If you want to print one or more variable values on the serial monitor on the same line, you can easily do that using the Serial.print()
function. This function takes a variable as an input argument and prints that variable on the serial monitor.
Syntax:
Serial.print(data);
The Serial.print()
function in Arduino takes a single parameter, which is the data you want to print to the serial monitor.
Parameter:
data
: The parameter that represents the data you want to print. It can be a variable, a constant, or any valid expression of a supported data type (e.g., integer, float, character, string, etc.), and the function will convert this data into a text representation and send it to the serial monitor.
For example, consider we want to print an integer on the serial monitor. See the example code below.
void setup() {
int myInteger = 10;
Serial.begin(9600);
Serial.print(myInteger);
}
void loop() {}
Output:
10
In this Arduino code, we have a setup()
function where we initialize the program. We declare an integer variable named myInteger
and set it to the value 10
.
Then, we set up serial communication using Serial.begin(9600)
, establishing a connection with a baud rate of 9600
bits per second. Next, we use Serial.print(myInteger)
to send the value of the myInteger
variable to the serial monitor, and this means that the number 10
will be displayed in the serial monitor when the program starts.
The loop()
function is empty in this example so that it won’t execute any specific tasks repeatedly. This sketch primarily focuses on demonstrating how to send data (in this case, the integer value) to the serial monitor during the setup phase.
It’s a simple example of using the Serial.print()
function for basic output to assist in debugging and monitoring your Arduino program.
Consider another example where we want to print a floating-point number on the serial monitor up to two decimal places. See the example code below.
void setup() {
float myFloat = 1.1234;
Serial.begin(9600);
Serial.print(myFloat, 2);
}
void loop() {}
Output:
1.12
In this Arduino code, we declare a floating-point variable named myFloat
and assign it the value 1.1234
. Then, we initialize the serial communication with a baud rate of 9600
using Serial.begin(9600)
.
To send the value of myFloat
to the serial monitor, we use Serial.print(myFloat, 2)
, where the 2
inside the parentheses specifies that we want to display the floating-point number with two decimal places. When the program runs, we will see 1.12
printed to the serial monitor.
As for the loop()
function, it remains empty in this code, indicating that no specific actions will be performed repeatedly. This code demonstrates how to send a floating-point number with a specified number of decimal places to the serial monitor, which can be useful for various applications, including sensor readings and data output.
Let’s have another example where we want to print multiple variables in the same line. See the example code below.
void setup() {
int first = 100;
int second = 200;
Serial.begin(9600);
Serial.print(first);
Serial.print("\t");
Serial.print(second);
}
void loop() {}
Output:
100 200
In this Arduino code, we define two integer variables, first
and second
, and assign them values of 100
and 200
, respectively. Afterward, we set up serial communication using Serial.begin(9600)
.
To display these values on the serial monitor, we use Serial.print(first)
to print the first
value, and then Serial.print("\t")
to add a tab character for formatting, followed by Serial.print(second)
to display the second
value. As a result, we’ll see 100 200
on the serial monitor.
The loop()
function remains empty, indicating that this code is primarily focused on sending these initial values to the serial monitor and doesn’t require any ongoing processes. This code demonstrates how to print multiple variables with appropriate formatting to the serial monitor, which can be handy for various monitoring and debugging tasks.
You can print as many variables as you like, and you can also use different characters to separate them from one another, like a comma.
Note that the Serial.print()
function only prints variables in a single line. If you want to print variables on multiple lines, you have to use the Serial.println()
function.
Print Variable Values on Serial Monitor Using the Serial.println()
Function in Arduino
If you want to print variables on different lines, you can do that easily using the Serial.println()
function in Arduino. This function performs the same as the Serial.print()
function, with the difference that this function goes to the next line after printing the variable value.
Syntax:
Serial.println(data);
The parameter for the Serial.println()
function in Arduino is the data you want to print to the serial monitor.
Parameter:
data
: The parameter that represents the data you want to print. It can be a variable, a constant, or any valid expression of a supported data type (e.g., integer, float, character, string, etc.), and the function will convert this data into a text representation and send it to the serial monitor, followed by a newline character to start a new line.
For example, consider you want to print multiple variables on different lines. See the example code below.
void setup() {
int first = 100;
int second = 200;
Serial.begin(9600);
Serial.println(first);
Serial.println(second);
}
void loop() {}
Output:
100
200
In this Arduino code, we declare two integer variables, first
and second
, assigning them values of 100
and 200
, respectively. Then, we initialize the serial communication using Serial.begin(9600)
.
To display these values on the serial monitor, we utilize Serial.println(first)
and Serial.println(second)
. This results in the values 100
and 200
being printed on the serial monitor, each on its own line.
The loop()
function remains empty in this code, indicating that there are no ongoing processes, and the code focuses on sending these initial values to the serial monitor for monitoring or debugging purposes. It demonstrates how to conveniently print variables on separate lines in the serial monitor.
Now, we want to print multiple values on multiple lines. See the example code below.
int first = 0;
int second = 10;
void setup() { Serial.begin(9600); }
void loop() {
while (first <= 10) {
Serial.print(first);
Serial.print("\t");
Serial.println(second);
first = first + 1;
second = second - 1;
}
while (1) {
}
}
In this Arduino code, we initialize two integer variables, first
set to 0
and second
set to 10
. In the setup()
function, we initiate serial communication using Serial.begin(9600)
.
Moving to the loop()
function, we enter a while
loop where as long as first
is less than or equal to 10
, we print the current values of first
and second
separated by a tab ("\t"
) and move to the next line. Inside this loop, first
is incremented by 1
, and second
is decremented by 1
during each iteration, and this continues until first
reaches 11
, at which point the loop exits.
After that, the code enters an infinite loop with while(1)
to halt any further execution.
Output:
0 10
1 9
2 8
3 7
4 6
5 5
6 4
7 3
8 2
9 1
10 0
The output shows where first
and second
values are printed side by side in the serial monitor, starting from 0
and 10
, respectively, and decreasing second
by 1
in each iteration until first
reaches 11
. Once first
exceeds 10
, the program enters an infinite loop and stops further execution.
This code is useful for observing the decrementing relationship between the two variables in real time through the serial monitor.
Conclusion
In this article, we’ve explored how to leverage the Serial.print()
and Serial.println()
functions to output variable values on the Arduino serial monitor. These functions offer versatility, whether we need to display data on a single line or format it neatly across multiple lines.
We can easily monitor, debug, and fine-tune your Arduino projects with this knowledge, ensuring they function smoothly and efficiently. These functions are crucial tools for any Arduino enthusiast, whether they are printing integers, floating-point numbers with specified decimal places, or many variables with changing formatting.