How to Clear Serial Buffer in Arduino
-
Use the
Serial.begin()
Function to Clear Serial Buffer in Arduino -
Use the
Serial.flush()
Function to Clear Serial Buffer in Arduino -
Use the
Serial.read()
Function to Clear Serial Buffer in Arduino - Conclusion
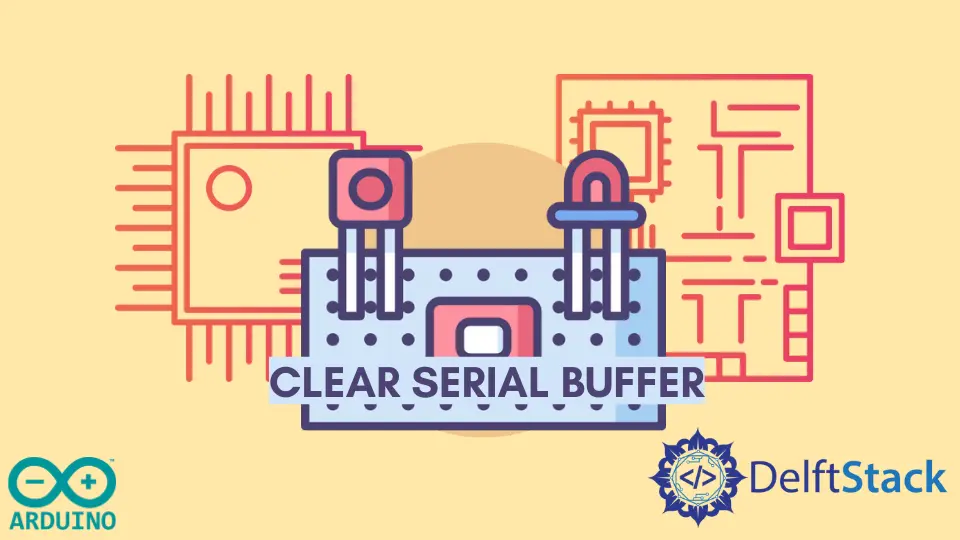
Arduino, a versatile microcontroller platform, is widely used for various projects involving sensors, actuators, and communication interfaces. When working with serial communication, it’s crucial to understand how to manage the serial buffer effectively.
This tutorial will discuss clearing the serial buffer using different methods in Arduino. But before that, let’s first understand what the serial buffer is.
In Arduino, the serial buffer is a temporary storage area for incoming data from the serial port. It holds data until the Arduino reads it or until new incoming data overwrites it.
Use the Serial.begin()
Function to Clear Serial Buffer in Arduino
Every Arduino board comes up with pins that can be used for serial communication, and we can use these pins to send and receive data through serial communication. The Serial
class of Arduino has a function that can read and write data to a serial pin.
We use the read()
function to read data from a serial pin, and the received data is stored inside a buffer before it is used inside the code. The buffer has a specific memory to store the data, and in case of a large amount of data, we have to clear the serial buffer so that it won’t run out of memory.
We can use the Serial.begin()
function to begin the serial after receiving data from the serial.
Syntax:
Serial.begin(speed);
speed
: This parameter specifies the baud rate at which the Arduino will communicate. It is an integer value representing the desired baud rate.
The Serial.begin()
function is used to initialize the serial with the given data rate, like 9600
bits per second, but when we initialize the serial, the previously stored data inside the serial buffer will be removed from the memory.
For example, let’s consider we want to read a string through a serial pin, and after receiving a chunk of data, we want to clear the serial buffer.
We can use the Serial.available()
function to check if the data is available in the serial buffer, and if it is available, we will store the data in a string array, and then we will clear the serial buffer using the Serial.begin()
function.
See the example code below.
String value;
void setup() {}
void loop() {
if (Serial.available()) {
value = "";
while (Serial.available()) {
char data = Serial.read();
value = value + data;
}
Serial.println(value);
Serial.end();
Serial.begin(9600);
}
}
We used the value
variable to store the character data we received from the serial. If data is available, we will add it to the array and end the serial communication using the Serial.end()
function and then initialize the serial to clear the serial buffer.
We used a loop to store all the available data before ending the serial communication and clearing the buffer.
If we want to send data, we can use the Serial.flush()
function to wait for all the data to be transmitted, and then we can end the serial communication and clear the buffer.
Use the Serial.flush()
Function to Clear Serial Buffer in Arduino
The Serial.flush()
function is a valuable tool in Arduino for managing serial communication. Its purpose is to ensure that all outgoing data has been transmitted before continuing with the program execution. Additionally, it clears both the transmit and receive buffers.
Syntax:
Serial.flush();
This single line of code is all you need to effectively clear the serial buffers.
When Serial.flush()
is called, the Arduino pauses the program execution until all outgoing data has been transmitted. This ensures that any data waiting in the transmit buffer is sent before moving on.
Additionally, Serial.flush()
clears the receive buffer, discarding any incoming data that hasn’t been read yet. This is particularly useful in scenarios where incoming data might be outdated or irrelevant.
Example code:
void setup() { Serial.begin(9600); }
void loop() {
// Simulating data transmission
Serial.println("Sending data...");
// Delay to allow time for data transmission
delay(1000);
// Clearing the serial buffer
Serial.flush();
// Checking if the buffer is cleared
if (Serial.available() == 0) {
Serial.println("Serial buffer cleared.");
} else {
Serial.println("Serial buffer not cleared.");
}
// Delay before sending the next set of data
delay(2000);
}
Here’s what each part of the syntax represents:
Serial
: This refers to theSerial
object, which is used for serial communication in Arduino.read()
: This is the function that reads one byte of incoming data from the serial buffer and returns it as an integer. If no data is available, it returns-1
.
To clear the serial buffer using Serial.read()
, we can employ a simple loop that reads and discards all available data in the buffer.
void setup() {
Serial.begin(9600); // Initialize serial communication at 9600 baud rate
}
void clearSerialBuffer() {
while (Serial.available()) {
Serial.read(); // Read and discard one byte of data from the buffer
}
}
void loop() {
// Simulate receiving some data
Serial.println("Received data: Hello, World!");
delay(1000); // Delay for demonstration purposes
// Clear the serial buffer
clearSerialBuffer();
// Check if the buffer is cleared
if (Serial.available() == 0) {
Serial.println("Serial buffer cleared successfully.");
} else {
Serial.println("Serial buffer was not cleared.");
}
delay(5000); // Delay for demonstration purposes
}
In the setup()
function, the serial communication is initialized at a baud rate of 9600
. There’s also a custom function named clearSerialBuffer()
defined, which uses a while
loop to read and discard any available data from the serial buffer.
In the loop()
function, it simulates receiving data by printing "Received data: Hello, World!"
to the serial monitor. A one-second delay is added for demonstration purposes, and then the clearSerialBuffer()
function is called to clear the serial buffer.
The code then checks if the buffer is cleared by using Serial.available()
. If the result is 0
, it prints "Serial buffer cleared successfully"
; otherwise, it prints "Serial buffer was not cleared."
.
Finally, there’s a five-second delay before the loop repeats. This code is useful for scenarios where you want to ensure that the serial buffer is cleared after receiving data to prevent any residual data from interfering with subsequent communications.
Output:
Received data: Hello, World!
Serial buffer cleared successfully.
Conclusion
This article explores efficient ways to manage the serial buffer in Arduino. It covers three methods: using Serial.begin()
, leveraging Serial.flush()
, and employing Serial.read()
.
The first one, Serial.begin()
, is a function that initializes serial communication, automatically clearing the buffer. This is useful for starting with a clean slate.
Next, Serial.flush()
is a powerful function that ensures all outgoing data is sent before the program proceeds, clearing both receive and transmit buffers. This is handy when incoming data might be outdated.
Lastly, Serial.read()
is a function that reads and discards available data, effectively clearing the buffer. Going by this method is useful after receiving data to prevent interference.
Each method is illustrated with clear explanations and example codes. By mastering these techniques, you can maintain efficient serial communication in your Arduino projects.