How to Convert String Into Array in JavaScript
-
Use the
JSON.parse()
Expression to Convert a String Into an Array -
Use the
Array.from()
Expression to Convert a String Into an Array -
Use the
...
Spread Operator to Convert a String Into an Array
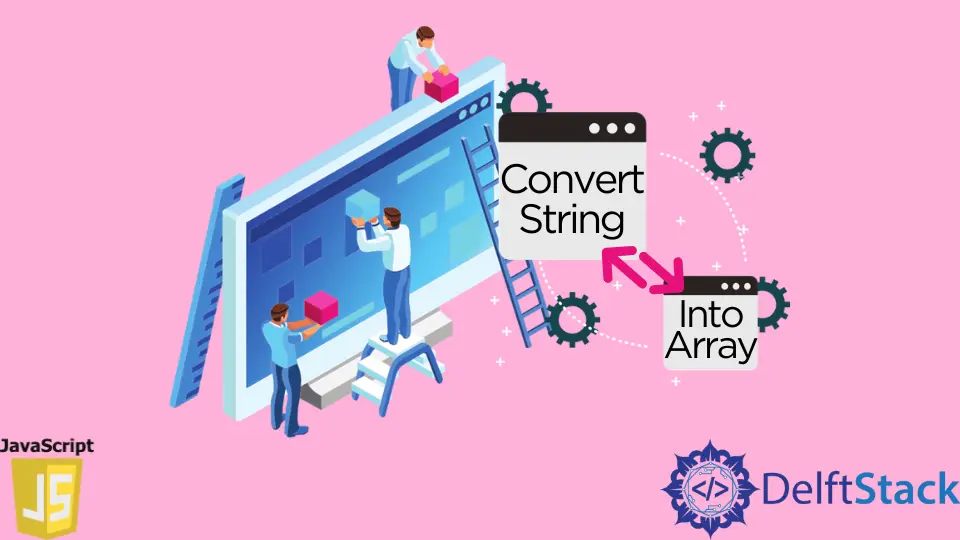
We will introduce three methods of converting a string into an array in JavaScript. We will convert how to convert the string to the numeric array as well as into an array of strings.
Use the JSON.parse()
Expression to Convert a String Into an Array
The JSON.parse()
expression is used to parse the data received from the web server into the objects and arrays. If the received data is in the form of a JSON object, it will convert it into a JavaScript object. And, if the data is a JSON value derived from an array, JSON.parse()
converts the data into a JavaScript array. We can use JSON.parse()
to convert a string of numbers separated by commas into an array. We can concatenate the brackets with the string to convert it to an array of numbers.
For example, create a variable data
and store a value 0,1,2,3
as a string in the variable. Use JSON.parse()
on the data
variable and concatenate the opening and closing brackets before and after the variable. Store the expression in a variable arr
. Then, log the variable in the console. Also, log the typeof
the first element of the array in the console.
In the example below, we converted a string of numbers into an array of numbers. We can know the type of the array items using the typeof
keyword. The output section shows that the array elements are of the number
type.
Example Code:
var data = '0,1,2,3';
var arr = JSON.parse('[' + data + ']');
console.log(arr)
console.log(typeof arr[0])
Output:
(4) [0, 1, 2, 3]
number
Use the Array.from()
Expression to Convert a String Into an Array
The Array.from()
method lets you create an array from a string of a certain length. We can pass the string that needs to be converted into the method, and the method will convert it into an array of strings. However, this method is not helpful if we have .
in between strings as it will also add ,
into the array of strings.
For example, create a variable num
and store a value 1234
as a string in the variable. Call the from()
method with an object Array
and supply the num
variable as the parameter in the function. Store the expression in a variable arr
. Then, use console.log()
to display the variable in the console. Also, log the typeof
the first element of the array in the console.
The example below converts a string of numbers into an array of strings. Each character of the string is converted as a string element of the array. The output section shows that the array elements are of the string
type.
Example Code:
var num = '1234';
var arr = Array.from(data);
console.log(arr)
console.log(typeof arr[0])
Output:
(4) ["1", "2", "3", "4"]
string
Use the ...
Spread Operator to Convert a String Into an Array
The spread operator is denoted by three dots followed by a variable ...data
. It is a widely used ES6 function. This method converts a string into an array of strings. However, this method is not useful if we have the commas in between strings as it will also add ,
into the array of strings. We can pass the variable containing the string inside big brackets. And before the variable, we can add three dots. i.e [...data]
.
For example, store a value of 1234
as a string in a variable data
. Write the variable data
inside the brackets and add three dots before it. Store this expression in a variable arr
. Then, log the variable in the console. Also, use the typeof
keyword before the first element of the array and log it into the console.
In the example, we converted a string of numbers into an array of strings as in the second method. But, this time, we used the spread operator. The output section shows that the array elements are of the string
type.
Example code:
var data = '1234';
var arr = [...data];
console.log(arr)
console.log(typeof arr[0])
Output:
(4) ["1", "2", "3", "4"]
string
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript