How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python
-
Causes and Solutions for
TypeError: Not All Arguments Converted During String Formatting
in Python - Conclusion
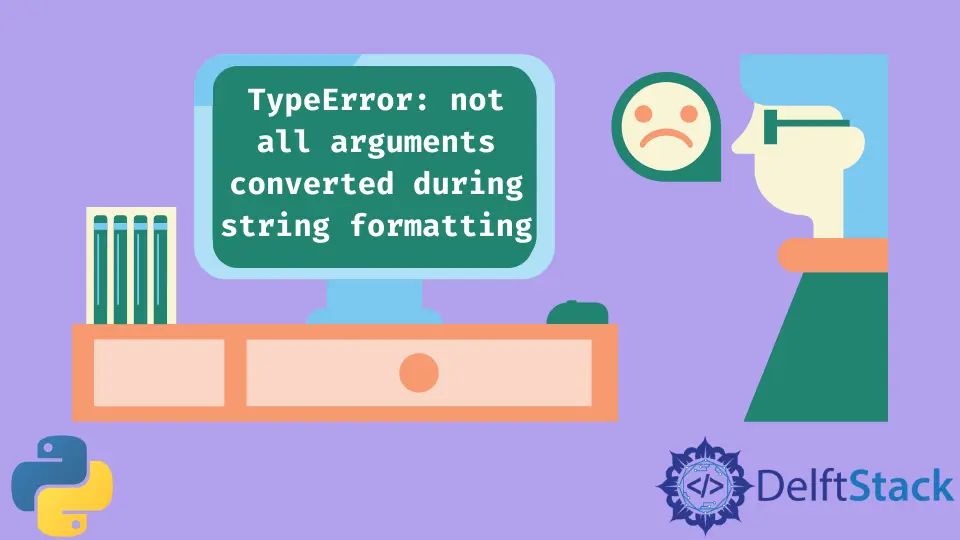
String formatting in Python can be done in various ways, and a modulo (%
) operator is one such method. It is one of the oldest methods of string formatting in Python, and using it in the wrong way might cause the TypeError: not all arguments converted during string formatting
to occur.
Not just that, many other reasons can cause the same. Let us learn more about this error and the possible solutions to fix it.
TypeError
is a Python exception raised when we try to operate on unsupported object types. There are many scenarios where we can get this error, but in this article, we will look at the Python TypeError
that occurs from the incorrect formatting of strings.
Without further ado, let us begin!
Causes and Solutions for TypeError: Not All Arguments Converted During String Formatting
in Python
There are many reasons why the TypeError: not all arguments converted during string formatting
might occur in Python. Let us discuss them one by one, along with the possible solutions.
Operations on the Wrong Data Type in Python
Look at the example given below. Here, we take the user’s age as input and then use the modulo operator (%
).
The result is stored in the variable ans
, which is then formatted to the string in the print
statement. But when we run this code, we get the TypeError
.
This happens because Python takes input in the form of a string. This means that the value 19
taken as the input is considered the str
data type by Python.
But can we use a modulo operator (%
) on a string? No, and hence, we get this error.
age = input("Enter your age: ")
ans = age % 10
print("Your lucky number is:", ans)
Output:
Enter your age: 19
Traceback (most recent call last):
File "<string>", line 2, in <module>
TypeError: not all arguments converted during string formatting
To fix this, we must take the input as an integer using the int()
function. This is done below.
age = int(input("Enter your age: "))
ans = age % 10
print("Your lucky number is:", ans)
Output:
Enter your age: 19
Your lucky number is: 9
You can see that this time we get the desired output since the int()
function converts the input to integer data type, and we can use the modulo operator (%
) on it.
Absence of Format Specifier With the String Interpolation Operator in Python
This case is a little bit similar to the one we discussed above. Look at the example given below.
The output shows us that the error occurred in line 2. But can you guess why?
The answer is simple. We know that anything inside single or double quotes in Python is a string.
Here, the value 19
is enclosed in double quotes, which means that it is a string data type, and we cannot use the modulo operator (%
) on it.
age = "19"
if age % 10:
print("Your age is a lucky number")
Output:
Traceback (most recent call last):
File "<string>", line 2, in <module>
TypeError: not all arguments converted during string formatting
To rectify this, we will use the int()
format specifier, as done below.
age = "19"
if int(age) % 10:
print("Your age is a lucky number")
Output:
Your age is a lucky number
This time the code runs fine.
Unequal Number of Format Specifiers and Values in Python
We know that we put as many format specifiers during string formatting as the number of values to be formatted. But what if there are more or fewer values than the number of format specifiers specified inside the string?
Look at this code. Here, we have three variables and want to insert only two of them into the string.
But when we pass the arguments in the print
statement, we pass all three of them, which no doubt results in the TypeError
.
lead_actor = "Harry Potter"
co_actor = "Ron Weasley"
actress = "Hermione Granger"
print("The males in the main role were: %s and %s." % (lead_actor, co_actor, actress))
Output:
Traceback (most recent call last):
File "<string>", line 5, in <module>
TypeError: not all arguments converted during string formatting
Let us remove the last variable, actress
, from the list of arguments and then re-run this program.
lead_actor = "Harry Potter"
co_actor = "Ron Weasley"
actress = "Hermione Granger"
print("The males in the main role were: %s and %s." % (lead_actor, co_actor))
Output:
The males in the main role were: Harry Potter and Ron Weasley.
You can see that this time the code runs fine because the number of format specifiers inside the string is equal to the number of variables passed as arguments.
Note that the format specifier to be used depends on the data type of the values, which in this case is %s
as we are dealing with the string data type.
Use of Different Format Specifiers in the Same String in Python
We know that there are several ways in which we can format a string in Python, but once you choose a method, you have to follow only that method in a single string. In other words, you cannot mix different types of string formatting methods in a single statement because it will lead to the TypeError: not all arguments converted during string formatting
.
Look at the code below to understand this concept better.
We are using placeholders({})
inside the string to format this string, but we do not pass the arguments as a tuple as we did earlier. Instead, one of the variables is preceded with a modulo operator (%
) while the other one is not.
This is what leads to the TypeError
.
lead_actor = "Harry Potter"
co_actor = "Ron Weasley"
actress = "Hermione Granger"
print("The males in the main role were: {0} and {1}." % lead_actor, co_actor)
Output:
Traceback (most recent call last):
File "<string>", line 5, in <module>
TypeError: not all arguments converted during string formatting
To fix this, we can do two things.
-
If you want to use
placeholders({})
inside the string, then you must use theformat()
method with the dot (.
) operator to pass the arguments. This is done below.lead_actor = "Harry Potter" co_actor = "Ron Weasley" actress = "Hermione Granger" print("The males in the main role were: {0} and {1}.".format(lead_actor, co_actor))
Output:
The males in the main role were: Harry Potter and Ron Weasley.
You can see that this time the code runs fine.
-
The other way could be to drop the use of
placeholders({})
and use format specifiers in their place along with passing the arguments as a tuple preceded by the modulo operator (%
). This is done as follows.lead_actor = "Harry Potter" co_actor = "Ron Weasley" actress = "Hermione Granger" print("The males in the main role were: %s and %s." % (lead_actor, co_actor))
Output:
The males in the main role were: Harry Potter and Ron Weasley.
Note that the modulo operator (
%
) for formatting is an old-style while, on the other hand, the use of the placeholders with the.format()
method is a new-style method of string formatting in Python.
This is it for this article. To learn more about string formatting in Python, refer to this documentation.
Conclusion
In this article, we talked about the various reasons that lead to TypeError: Not all arguments converted during string formatting
in Python, along with the possible fixes.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python