How to Solve the TypeError: An Integer Is Required in Python
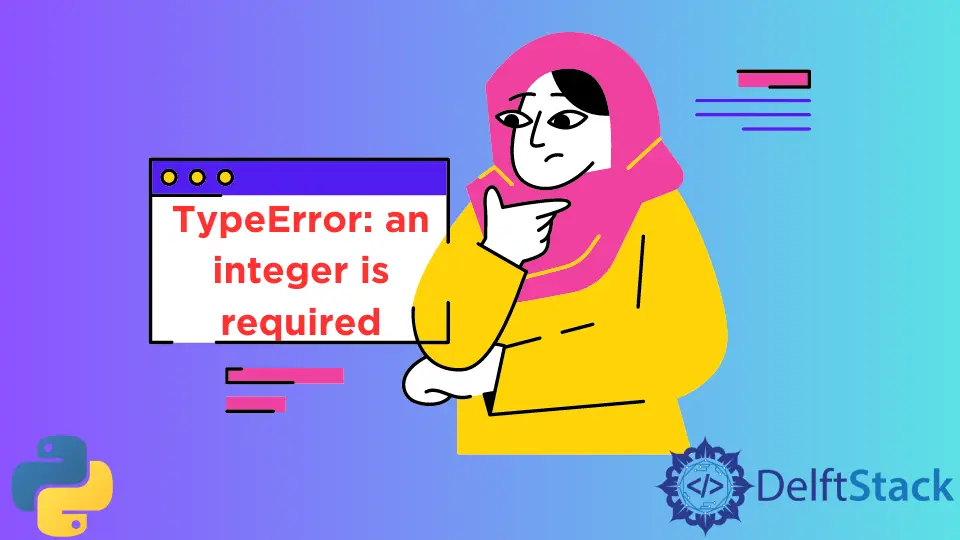
Another most common error that occurs during code in Python is TypeError
. It is an exception in Python.
You will get this error when you use an inappropriate data type of object in an operation.
This article will show how we get a TypeError
in Python. Also, we will discuss the topic by using necessary examples and explanations to make the topic easier.
How the TypeError
Occurs in Python
As we already discussed above, the cause of this error is the use of inappropriate data types in an operation. Suppose you have written the below Python program.
num = list(str(345678))
for i in num:
print(chr(i))
When you try to run the above example, you will get an error like the one below.
Traceback (most recent call last):
File "<string>", line 5, in <module>
TypeError: an integer is required (got type str)
The problem in the example above is that we passed an incompatible data type inside the function chr()
on the line print(chr(i))
.
Let’s see another example that produces the same error.
In this example, we will perform a divide operation on two variables. The Python code for this purpose is like below.
MyInt = 45
MyInt2 = "5"
Result = MyInt / MyInt2
print("Result is : ", Result)
In our example above, we divide the integer type variable by a string type variable incompatible with this operation. So after running this example, you will get an error like the one below.
Traceback (most recent call last):
File "<string>", line 3, in <module>
TypeError: unsupported operand type(s) for /: 'int' and 'str'
How to Resolve the TypeError: an integer is required
To resolve this error, we need to fix the data type. For our first example, you can fix the code as follows:
num = list(str(345678))
for i in num:
print(i)
Here you can notice that we just fixed the line where the data type is incompatible with the operation, and another part of the program remains the same.
After fixing the first example, you will get an output like the one below.
3
4
5
6
7
8
Now let’s solve our second example. To fix our second example, we need to remove the double quote from the second variable like below.
MyInt = 45
MyInt2 = 5
Result = MyInt / MyInt2
print("Result is : ", Result)
And you will see that the error was resolved, and you will get the output below.
Result is : 9.0
Please take note that the commands and programs discussed here are written in the Python programming language.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - Python TypeError
- How to Fix the Python TypeError: List Indices Must Be Integers, Not List
- How to Fix TypeError: Iteration Over Non-Sequence
- How to Fix TypeError: Must Be Real Number, Not STR
- How to Fix Python TypeError: Unhashable Type: List
- How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python