How to Fix Python TypeError: Unhashable Type: List
-
the
TypeError: unhashable type: 'list'
in Python - Hash Function in Python
-
Fix the
TypeError: unhashable type: 'list'
in Python
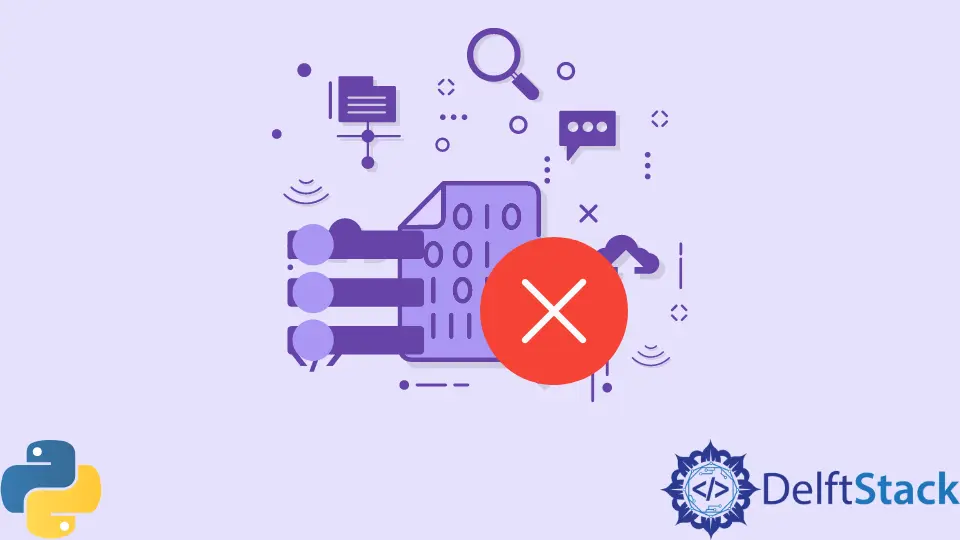
This article will discuss the TypeError: unhashable type: 'list'
and how to fix it in Python.
the TypeError: unhashable type: 'list'
in Python
This error occurs when you pass the unhashable objects like a list as a key to the Python dictionary or find a function’s hash value.
Dictionaries is a data structure in Python that works in key-value pairs, every key has a value against it, and to access the values of values, you will need the keys like array indices.
Syntax of a dictionary:
dic = {"key": "Values"}
Hashable objects are those whose value doesn’t change over time but remain the same tuples
and strings
are types of hashable objects.
Code:
# creating a dictionary
dic = {
# list as a key --> Error because lists are immutable
["a", "b"]: [1, 2]
}
print(dic)
Output:
TypeError: unhashable type: 'list'
We have used a list ["a","b"]
as the key
, but the compiler has thrown a TypeError: unhashable type: 'list'
.
Let’s manually find the hash value of the list.
Code:
lst = ["a", "b"]
hash_value = hash(lst)
print(hash_value)
Output:
TypeError: unhashable type: 'list'
The hash()
function is used to find the hash value of a given object, but the object must be immutable like string
, tuple
, etc.
Hash Function in Python
The hash()
function is an encryption technique that encrypts the immutable object and assigns a unique value to it, known as the object’s hash value. No matter the data’s size, it provides the same size of unique value.
Code:
string_val = "String Value"
tuple_val = (1, 2, 3, 4, 5)
msg = """Hey there!
Welcome to DelfStack"""
print("Hash of a string object\t\t", hash(string_val))
print("Hash of a tuple object\t\t", hash(tuple_val))
print("Hash of a string message\t", hash(tuple_val))
Output:
Hash of a string object -74188595
Hash of a tuple object -1883319094
Hash of a string message -1883319094
The hash values are the same size and unique for every value.
Fix the TypeError: unhashable type: 'list'
in Python
To fix the TypeError in Python, you have to use immutable objects as the keys of dictionaries and as arguments to the hash()
function. Notice in the above code the hash()
function works perfectly with mutable objects like tuple
and string
.
Let’s see how we can fix the TypeError: unhashable type: 'list'
in dictionaries.
Code:
# creating a dictionary
dic = {
# string as key
"a": [1, 2]
}
print(dic)
Output:
{'a': [1, 2]}
This time we provide a string "a"
as the key, which is fine to work with because strings
are mutable.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python
Related Article - Python TypeError
- How to Fix the Python TypeError: List Indices Must Be Integers, Not List
- How to Fix TypeError: Iteration Over Non-Sequence
- How to Fix TypeError: Must Be Real Number, Not STR
- How to Solve the TypeError: An Integer Is Required in Python
- How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python