How to Fix the Python TypeError: List Indices Must Be Integers, Not List
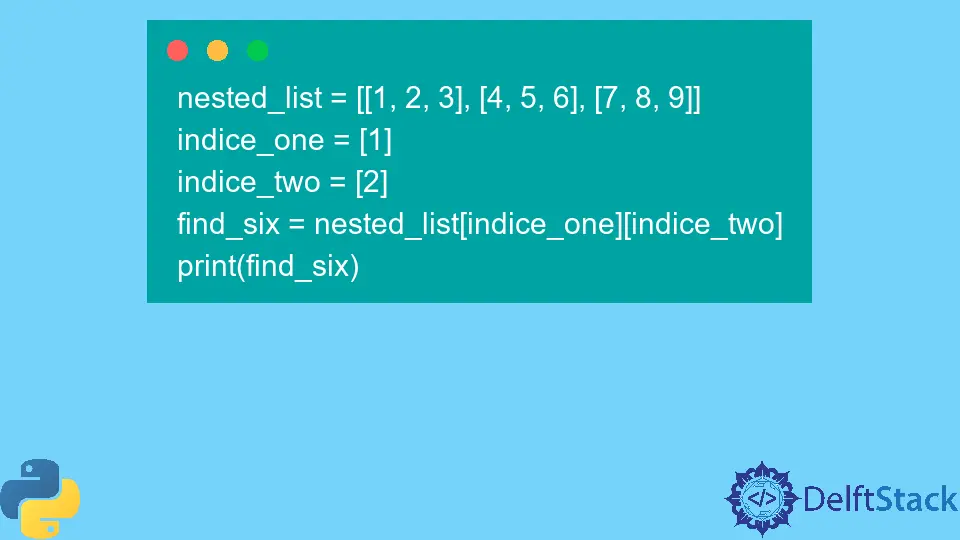
We will introduce a nested list and the common error many programmers face while trying to access elements from them with examples in Python.
Fix the TypeError: list indices must be integers, not list
in Python
Lists are one of Python’s most commonly used and versatile data types. Lists can be used in a variety of applications.
A nested list contains elements that contain a list inside them. It is also known as a nested array used to store, organize and manipulate multi-dimensional data.
An example of a nested list in Python is shown below.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
This is a common error when working with nested lists. When accessing an item in a nested list, we need to specify its indices twice.
The first will be for the outer list and the second for the inner list.
Let’s try to access 5 from the above example using Python, as shown below.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
find_five = nested_list[1][1]
print(find_five)
The output of the above code will be as shown below.
But if we try to access an element wrong, we can get the error message. Let’s try to recreate situations in which this error can occur.
For example, if we try to pass one index with both values instead of passing two indices separately, it will throw an error.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
find_five = nested_list[1, 1]
print(find_five)
This will throw the error as shown below.
There can be one more situation where we could get the same error when we have saved the indices inside a variable wrongly as a list instead of type int
, as shown below.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
indice_one = [1]
indice_two = [2]
find_six = nested_list[indice_one][indice_two]
print(find_six)
The output of the above code is shown below.
The best way to avoid this error is by calling items by indices of correct types. If you store the indices in variables, you need to assign them as integers, as shown below.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
indice_one = 1
indice_two = 2
find_six = nested_list[indice_one][indice_two]
print(find_six)
Assigning the values of indices as an int
type will never throw the error. The above code will run as shown below.
In conclusion, nested lists are a powerful data structure in Python, but it’s important to remember that lists can only be indexed using integers. If we encounter the TypeError: list indices must be integers, not list
error, we are trying to access a list using a value that is not an integer.
To resolve this error, use an integer value instead.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python TypeError
- How to Fix TypeError: Iteration Over Non-Sequence
- How to Fix TypeError: Must Be Real Number, Not STR
- How to Solve the TypeError: An Integer Is Required in Python
- How to Fix Python TypeError: Unhashable Type: List
- How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python